Event Sourcing in Microservices with Axon Framework
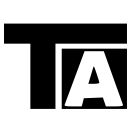
6 min read
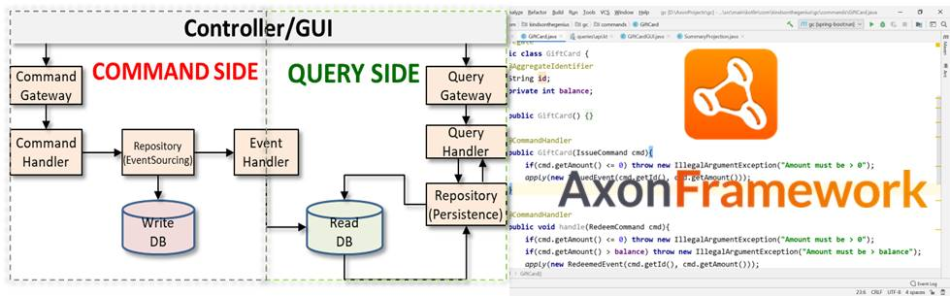
1. What is Event Sourcing?
Event sourcing is a design pattern where all state changes are captured as a sequence of events. Rather than saving the current state, the system records each change, allowing the state to be rebuilt by replaying these events. This method is powerful for systems where auditability and tracking are essential.
1.1 How Event Sourcing Works
In event sourcing, every change to the application’s state is stored as a separate event. This approach provides a single source of truth for reconstructing past states and understanding how the system arrived at its current state.
For example, in a banking application, each transaction is saved as an event: deposits, withdrawals, and transfers. When querying the account balance, the system calculates the balance by replaying all transactions related to that account.
The benefits of event sourcing go beyond simple state management:
- Audit Trail: Since each change is captured as an event, you have a complete audit trail of all actions.
- State Replay: You can rebuild the application’s state from any point in the past, useful for debugging or analytical purposes.
- Event-Driven Processing: Systems can respond to changes asynchronously, allowing for more responsive and scalable architectures.
2. Implementing Event Sourcing with Axon Framework
Axon Framework makes it easier to manage and store events in a microservices environment. It provides ready-made components for handling commands, storing events, and replaying events to rebuild the aggregate state.
2.1 Defining Aggregates and Commands
Aggregates are the core of Axon’s event sourcing model, representing the main entities in your domain. Commands are used to request changes to these aggregates.
Example Code: Defining an Aggregate
@Aggregate
public class AccountAggregate {
@AggregateIdentifier
private String accountId;
private double balance;
public AccountAggregate() {}
@CommandHandler
public AccountAggregate(CreateAccountCommand command) {
AggregateLifecycle.apply(new AccountCreatedEvent(command.getAccountId(), command.getInitialBalance()));
}
@EventSourcingHandler
public void on(AccountCreatedEvent event) {
this.accountId = event.getAccountId();
this.balance = event.getInitialBalance();
}
}
In this example:
- @Aggregate marks the AccountAggregate as the main entity for managing the account’s state.
- @CommandHandler processes incoming commands. In this case, CreateAccountCommand triggers an AccountCreatedEvent.
- @EventSourcingHandler captures and applies the event to update the aggregate state.
2.2 Storing and Replaying Events
Axon automatically stores events in an event store. You can replay these events to reconstruct an aggregate’s state whenever necessary.
Example Code: Handling Account Transactions
@CommandHandler
public void handle(DepositMoneyCommand command) {
if (command.getAmount() <= 0) {
throw new IllegalArgumentException("Deposit amount must be positive");
}
AggregateLifecycle.apply(new MoneyDepositedEvent(this.accountId, command.getAmount()));
}
@EventSourcingHandler
public void on(MoneyDepositedEvent event) {
this.balance += event.getAmount();
}
In this example, the DepositMoneyCommand triggers the MoneyDepositedEvent, updating the aggregate’s balance. By applying each event, Axon replays the account’s transaction history to rebuild the current balance.
2.3 Command and Query Responsibility Segregation (CQRS)
Axon Framework integrates CQRS, enabling separate models for handling write (command) and read (query) operations. This is particularly useful for microservices, where separating read and write operations improves scalability and performance.
Example Code: Using Query Handlers
@EventHandler
public void on(MoneyDepositedEvent event, @Autowired AccountRepository accountRepository) {
AccountEntity account = accountRepository.findById(event.getAccountId()).orElseThrow();
account.setBalance(account.getBalance() + event.getAmount());
accountRepository.save(account);
}
In this code, MoneyDepositedEvent triggers an update to the AccountEntity, the read model representation in the database, reflecting the latest balance.
3. Best Practices for Event Sourcing with Axon Framework
Axon Framework is highly flexible, but following best practices ensures you leverage its full potential effectively:
3.1 Snapshots for Performance Optimization
As aggregates grow, replaying every event can slow down the system. By taking snapshots at regular intervals, you can significantly reduce the load time for aggregates.
Example Code: Configuring Snapshots
@Bean
public SnapshotTriggerDefinition snapshotTriggerDefinition(Snapshotter snapshotter) {
return new EventCountSnapshotTriggerDefinition(snapshotter, 50);
}
This code creates a snapshot after every 50 events, allowing Axon to replay only recent events when rebuilding the aggregate.
3.2 Use a Dedicated Event Store
Although Axon allows you to use a relational database for event storage, it is best to use a dedicated event store like Axon Server. Purpose-built event stores offer optimized storage and retrieval for high-throughput systems.
3.3 Decoupling Command and Query Models
In a CQRS setup, avoid coupling the command and query sides. This separation allows each model to evolve independently, improving scalability and maintainability.
4. Addressing Common Challenges with Axon Framework
Event sourcing introduces unique challenges. Understanding and addressing them helps ensure a smooth implementation:
Achieving Consistency Across Microservices
In distributed systems, achieving consistency across services is essential. Axon Framework supports eventual consistency, but you can further enhance reliability by using tools like Axon’s distributed command bus.
Scaling Event Stores
For high-throughput systems, scaling the event store is critical. Options like Apache Kafka or Axon Server are well-suited for storing events in distributed architectures, ensuring reliable event processing and storage.
Managing Event Versioning
Over time, events may require new fields or changes. Axon allows you to handle these versions gracefully through backward-compatible event structures.
public class AccountCreatedEventV2 extends AccountCreatedEvent {
private String customerName;
public AccountCreatedEventV2(String accountId, double initialBalance, String customerName) {
super(accountId, initialBalance);
this.customerName = customerName;
}
// Getters and setters
}
5. Implementing Event-Driven Communication with Axon
Axon makes it easy to handle communication between microservices in an event-driven architecture. Using Axon’s command bus and event bus, services can publish and subscribe to events asynchronously, improving system scalability and responsiveness.
Example Code: Event Publisher
public class OrderAggregate {
@CommandHandler
public void handle(PlaceOrderCommand command) {
// Business logic
AggregateLifecycle.apply(new OrderPlacedEvent(command.getOrderId(), command.getOrderDetails()));
}
@EventSourcingHandler
public void on(OrderPlacedEvent event) {
// Update aggregate state
}
}
Here, the PlaceOrderCommand results in an OrderPlacedEvent, which other services can subscribe to, allowing them to react to the order placement asynchronously.
6. Conclusion
Event sourcing with Axon Framework provides a robust way to implement scalable, resilient microservices. Axon simplifies event handling, storage, and playback, and combined with CQRS, offers a powerful solution for complex business systems. By following best practices, such as using snapshots and dedicated event stores, you can ensure a production-ready setup.
If you have any questions or want to share your experience, feel free to leave a comment below!
Read more at : Event Sourcing in Microservices with Axon Framework
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
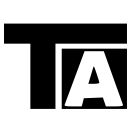
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.