🚀How to Rapidly Launch an EC2 Instance with AWS CLI
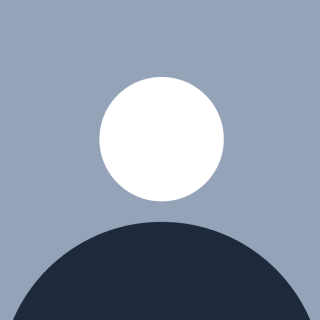

We've all been there—clicking through the AWS Console step by step to launch an EC2 instance:
✅ Select AMI
✅ Choose instance type
✅ Configure instance details
✅ Add storage
✅ Set security groups
✅ Add tags
✅ Launch...
It’s easy, but also repetitive. And if you're someone who spins up EC2s often for learning, testing, or short-term projects, it feels like reinventing the wheel every single time.
😩 The Problem
"Isn’t there a faster way? Something where I don’t have to go through the GUI every time?"
Yes, there is.
Think of it this way: what if we had a script that we could just copy-paste and boom 💥—your EC2 instance is up and running with all required settings?
💡 The Idea
Let’s automate EC2 provisioning using a simple AWS CLI script. You can reuse this script and customize it easily.
🔧 What This Script Will Do:
✅ Create a security group (if it doesn't exist)
🔓 Add inbound rules for SSH, HTTP, HTTPS
🔐 Create or reuse an existing key pair
🚀 Launch an EC2 instance with your configuration
📜 The Script (AWS CLI Bash Script)
bashCopyEdit#!/bin/bash
# ------------ CONFIGURABLE VARIABLES ------------ #
AMI_ID="ami-0c02fb55956c7d316" # Amazon Linux 2 (us-east-1)
INSTANCE_TYPE="t2.micro"
KEY_NAME="my-key"
SECURITY_GROUP_NAME="my-sec-group"
TAG_NAME="CLI-Instance"
REGION="us-east-1"
# ------------ CREATE SECURITY GROUP ------------ #
echo "Creating Security Group: $SECURITY_GROUP_NAME..."
SECURITY_GROUP_ID=$(aws ec2 create-security-group \
--group-name $SECURITY_GROUP_NAME \
--description "Security group with SSH, HTTP, HTTPS access" \
--region $REGION \
--query 'GroupId' \
--output text)
# ------------ ADD INBOUND RULES ------------ #
echo "Authorizing inbound rules..."
aws ec2 authorize-security-group-ingress --group-id $SECURITY_GROUP_ID --protocol tcp --port 22 --cidr 0.0.0.0/0 --region $REGION
aws ec2 authorize-security-group-ingress --group-id $SECURITY_GROUP_ID --protocol tcp --port 80 --cidr 0.0.0.0/0 --region $REGION
aws ec2 authorize-security-group-ingress --group-id $SECURITY_GROUP_ID --protocol tcp --port 443 --cidr 0.0.0.0/0 --region $REGION
# ------------ CREATE KEY PAIR (if not exists) ------------ #
if [ ! -f "${KEY_NAME}.pem" ]; then
echo "Creating new key pair: $KEY_NAME..."
aws ec2 create-key-pair --key-name $KEY_NAME \
--query 'KeyMaterial' \
--region $REGION \
--output text > ${KEY_NAME}.pem
chmod 400 ${KEY_NAME}.pem
else
echo "Key pair already exists: $KEY_NAME.pem"
fi
# ------------ RUN EC2 INSTANCE ------------ #
echo "Launching EC2 Instance..."
aws ec2 run-instances \
--image-id $AMI_ID \
--count 1 \
--instance-type $INSTANCE_TYPE \
--key-name $KEY_NAME \
--security-group-ids $SECURITY_GROUP_ID \
--tag-specifications "ResourceType=instance,Tags=[{Key=Name,Value=$TAG_NAME}]" \
--region $REGION
echo "✅ Instance launched successfully!"
🧪 How to Use
Save the script as
launch-ec2.sh
Give it permission:
chmod +x launch-ec2.sh
Run it:
./launch-ec2.sh
🧠 Why This Is Useful
💻 Speeds up learning environments
🧪 Ideal for testing and automation
📦 Great for DevOps practice
🔁 Reusable for daily work
🔄 If You Want to Go Further?
Add support for Elastic IP, Volumes, UserData
Turn this into a Terraform script
Integrate it in CI/CD pipelines
✨ Final Thoughts
Automating EC2 launches is a simple yet powerful step toward DevOps maturity. Whether you're learning, testing, or scaling, saving time and avoiding human errors will always benefit you.
So next time, skip the console clicks. Just copy, paste, and let your script do the magic.
Subscribe to my newsletter
Read articles from Prem Kr Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Prem Kr Gupta
Prem Kr Gupta
A software developer aiming to become a DevOps engineer, exploring and sharing insights on DevOps methodology.