PHP: Creating A Job Application System
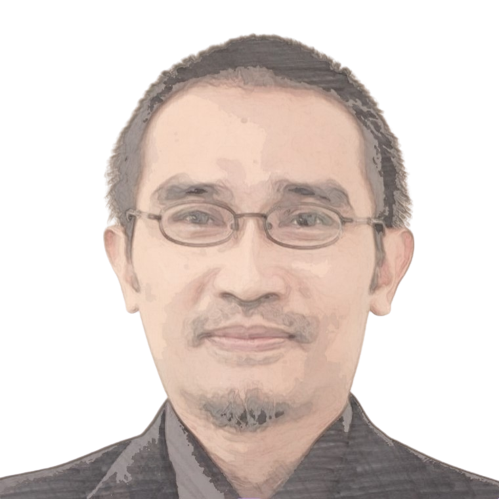
Learn how to create a simple job application system using plain PHP!
This blog will guide you through creating a simple job application system using plain PHP, demonstrating a basic flow where users submit their applications through a form on the main page. After filling out the form and uploading their resumes, the data is processed and stored in an SQLite database, followed by a redirect to a success page confirming their submission. You will primarily use PHP for server-side logic, along with HTML and CSS for the front end, utilizing PDO for database interactions. By the end of this tutorial, you’ll have a functional application that serves as a boilerplate for further enhancements, making it ready for production use.
[1] Create a blank PHP project
In Replit, create a new app. Select the PHP Web Server template. Name your project (e.g., vacancy101) and click Create App.
This step sets up the environment where your PHP application will run. By selecting the PHP Web Server template, you ensure that the necessary server infrastructure is in place.
[2] Set Up Project Structure
This project will store sqlite database in data
folder and uploaded pdf in uploads
folder.
To enhance security, it’s best to store these folders outside of the web-accessible directory. This prevents direct access to sensitive files via the web, reducing the risk of unauthorized access.
Here’s how the project structure will look in Replit:
~
|- data
|- uploads
|- workspace
|- vacancy
|- config.php
|- index.php
|- apply.php
|- process.php
|- style.css
|- setup.php
The files will serve different purposes in your application:
config.php: Contains database connection configurations.
index.php: The main landing page of your application.
apply.php: Handles the application submission logic.
process.php: Displays a success message after submission.
style.css: Contains styles for your application.
setup.php: Sets up the database and tables.
In the Shell window, run the following commands one after another (the Shell will start in the ~/workspace directory by default).
mkdir vacancy
cd vacancy
touch config.php index.php apply.php process.php style.css setup.php
[3] Run setup
Add the following codes to setup.php
:
<?php
// Database path
$dbPath = '../../data/vacancy.db';
try {
// Define folders to create
$folders = ["../../data", "../../uploads"];
// Create necessary folders
foreach ($folders as $folderName) {
if (!file_exists($folderName)) {
mkdir($folderName, 0755, true);
echo "Folder '$folderName' created successfully!\n";
} else {
echo "Folder '$folderName' already exists.\n";
}
}
// Create a new SQLite database connection
$pdo = new PDO("sqlite:$dbPath");
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Set error mode to exception
// SQL to create the applications table
$sql = "CREATE TABLE IF NOT EXISTS applications (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
mobile_no TEXT NOT NULL,
email TEXT NOT NULL,
resume TEXT NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
)";
// Execute the SQL command
$pdo->exec($sql);
echo "Table 'applications' created successfully.\n";
} catch (PDOException $e) {
echo "Database error: " . $e->getMessage();
} catch (Exception $e) {
echo "General error: " . $e->getMessage();
}
?>
In the Shell panel, run the script (assuming you are already in the ~/workspace/vacancy directory):
php setup.php
Outcome:
After setup.php
is executed, it creates the data
and uploads
folders and initializes the SQLite database along with the applications table for the vacancy application.
The notation ../../
is a relative path used in file systems to navigate directories. Specifically, it means:
..
: Move up one directory level.../../
: Move up two directory levels.
[4] Define connection configuration
Add the following codes to config.php
:
<?php
// Database path
$dbPath = '../../data/vacancy.db';
try {
// Create a new SQLite database connection
$pdo = new PDO("sqlite:$dbPath");
// Set the PDO error mode to exception
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
This code establishes a connection to your SQLite database, allowing other scripts to interact with the database through this connection configuration.
[5] Define the entry script
By default, the entry script is index.php
.
Add the following codes to the file:
<?php include 'config.php'; ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="../vacancy/style.css">
<title>Vacancy Application</title>
</head>
<body>
<h1>Welcome to the Vacancy Application Portal</h1>
<a href="../vacancy/apply.php">Apply Now</a>
</body>
</html>
This is the entry point of your application where users will land. It should provide options for users to apply for jobs or navigate to other sections.
Output:
[6] Define the apply script
Add the following codes to apply.php
:
<?php include 'config.php'; ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Apply for Vacancy</title>
</head>
<body>
<h1>Vacancy Application Form</h1>
<form action="process.php" method="POST" enctype="multipart/form-data">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<br>
<label for="mobile_no">Mobile Number:</label>
<input type="text" id="mobile_no" name="mobile_no" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<br>
<label for="resume">Resume (PDF only):</label>
<input type="file" id="resume" name="resume" accept=".pdf" required>
<br>
<input type="submit" value="Submit Application">
</form>
</body>
</html>
This script processes the job application form submission, validates inputs, handles file uploads, and inserts data into the database.
Output:
[7] Define process script
Add the following codes to process.php
:
<?php
require 'config.php';
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$name = $_POST['name'];
$mobile_no = $_POST['mobile_no'];
$email = $_POST['email'];
// File upload handling
$targetDir = "../../uploads/";
$resumeFile = $_FILES['resume'];
$targetFile = $targetDir . basename($resumeFile["name"]);
$uploadOk = 1;
$fileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION));
// Validate file type
if ($fileType != "pdf") {
echo "<div class='message error'>Sorry, only PDF files are allowed.</div>";
$uploadOk = 0;
}
// Check if upload is ok
if ($uploadOk == 1) {
if (move_uploaded_file($resumeFile["tmp_name"], $targetFile)) {
// Insert application details into the database
$stmt = $pdo->prepare("INSERT INTO applications (name, mobile_no, email, resume) VALUES (?, ?, ?, ?)");
$stmt->execute([$name, $mobile_no, $email, $targetFile]);
echo "<link rel='stylesheet' type='text/css' href='style.css'>";
echo "<div class='message success'><h1>Application Submitted Successfully!</h1></div>";
echo "<a class='button' href='index.php'>Back to Home</a>";
} else {
echo "<link rel='stylesheet' type='text/css' href='style.css'>";
echo "<div class='message error'>Sorry, there was an error uploading your file.</div>";
}
} else {
echo "<link rel='stylesheet' type='text/css' href='style.css'>";
echo "<div class='message error'>Your file was not uploaded.</div>";
}
} else {
header("Location: index.php");
exit();
}
?>
After a successful application submission, this page will provide feedback to the user, confirming that their application has been received.
Output:
[8] Define page style
Add the following codes to style.css
:
body {
font-family: Arial, sans-serif;
margin: 20px;
padding: 20px;
background-color: #f4f4f4;
}
h1 {
color: #333;
}
form {
background: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
label {
display: block;
margin: 10px 0 5px;
}
input[type="text"],
input[type="email"],
input[type="file"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[type="submit"] {
background: #5cb85c;
color: white;
border: none;
padding: 10px 15px;
border-radius: 5px;
cursor: pointer;
}
.message {
padding: 20px;
margin: 20px;
border-radius: 5px;
text-align: center;
}
.success {
background-color: #4caf50; /* Green */
color: white;
}
.error {
background-color: #f44336; /* Red */
color: white;
}
.button {
display: inline-block;
padding: 10px 20px;
margin-top: 20px;
background-color: #007bff; /* Blue */
color: white;
text-decoration: none;
border-radius: 5px;
}
.button:hover {
background-color: #0056b3; /* Darker blue */
}
This CSS file contains styles that enhance the appearance of your application, ensuring it is visually appealing and user-friendly.
[9] Define QuickView CLI script
Add the following codes to quickview.php
:
<?php
require 'config.php';
try {
// Query to select all records from the applications table
$stmt = $pdo->query("SELECT * FROM applications");
$applications = $stmt->fetchAll(PDO::FETCH_ASSOC);
// Check if there are any records
if (count($applications) > 0) {
// Output the table headers
echo "ID\tName\tMobile No\tEmail\tResume\tCreated At\n";
echo str_repeat("-", 80) . "\n"; // Separator line
// Loop through each application and output in tab-separated format
foreach ($applications as $application) {
echo "{$application['id']}\t{$application['name']}\t{$application['mobile_no']}\t{$application['email']}\t{$application['resume']}\t{$application['created_at']}\n";
}
} else {
echo "No applications found.\n";
}
} catch (PDOException $e) {
echo "Error: " . $e->getMessage() . "\n";
}
?>
This script allows you to view the contents of the database directly from the command line, making it easier to manage and review submitted applications.
Output:
[10] Conclusion
In conclusion, you have successfully built a basic job application system using plain PHP, providing a solid foundation for anyone looking to explore web development. This project not only demonstrates essential concepts such as form handling, file uploads, and database interactions but also serves as a boilerplate that can be easily enhanced for production. By adding features like user authentication, email notifications, and improved validation, you can transform this simple application into a comprehensive solution tailored to real-world needs. With this knowledge, you’re now equipped to further develop and customize your own job application system.
🤓
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
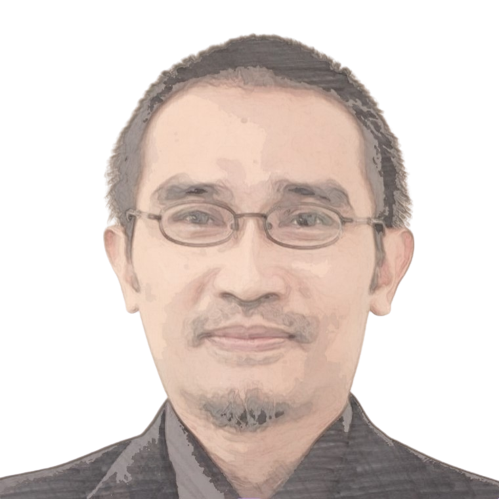
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).