Why 100 Lines of Code Can Outshine Just Twođź’»
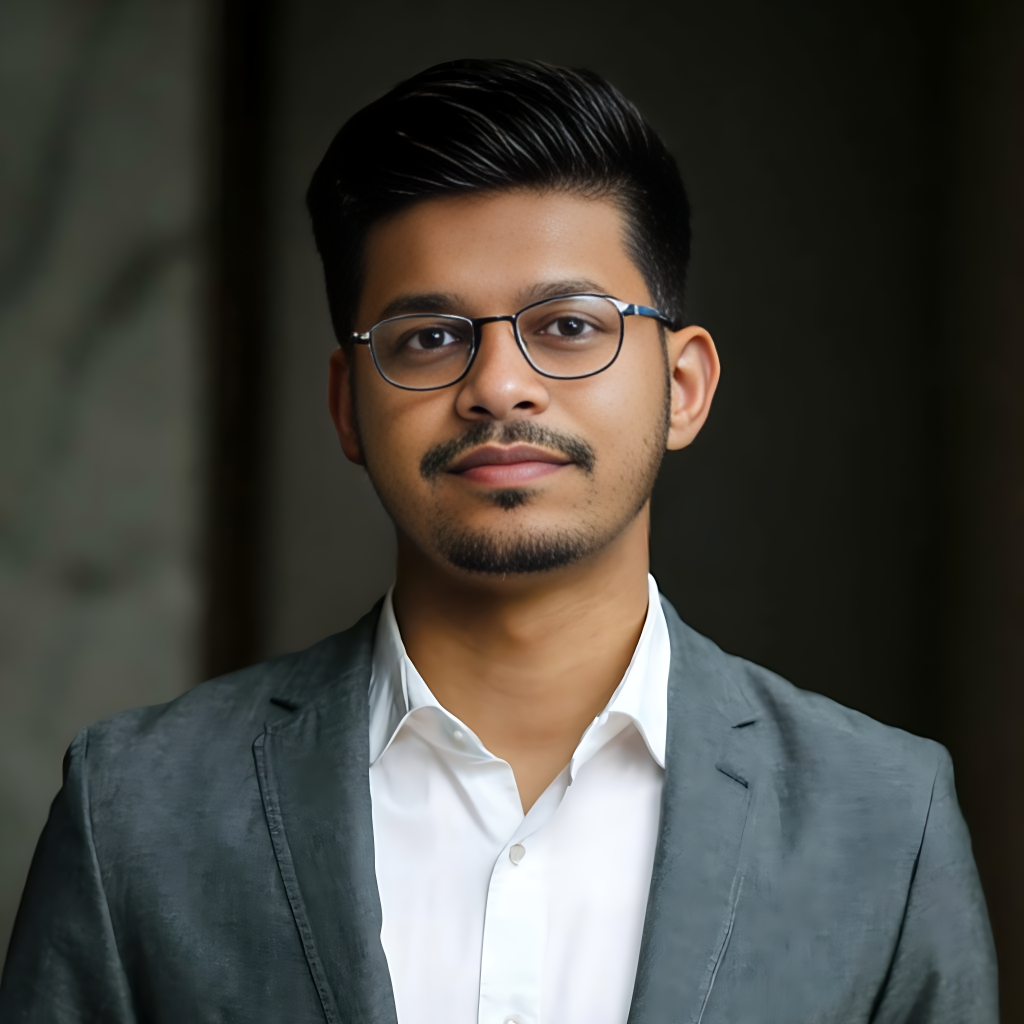

Introduction 🛜
In the world of programming, brevity is often praised — but is shorter always better? While concise code can be elegant, sometimes a longer, more explicit approach offers greater clarity, maintainability, and flexibility. Let’s explore why writing 100 lines of code might actually outshine squeezing everything into just two.
Problem statement 🍉
You are given 9 watermelons, and one of them is spoiled. How can you identify the spoiled one?
🍉Solution 1 : The Straightforward Approach (using a Loop)
We iterate through each watermelon, checking its weight. All fresh watermelons weigh 1 unit, but the spoiled one weighs 2. By using a simple for loop, we can identify the spoiled the watermelon based on this weight anomaly.
int watermelon[9] = {1, 1, 1, 1, 1, 1, 1, 2, 1};
for (int i = 0; i < 9; i++) {
if (watermelon[i] == 2) {
cout << "Spoiled watermelon found at index: " << i << endl;
return i;
}
}
This approach is simple, readable, and effective — but it comes at the cost of iterating through all elements.
🍉Solution 2 : Divide and Conquer Using Baskets
Instead of scanning all watermelons linearly , we group them into three baskets — each holding three watermelons. By comparing the total weight of each basket, we can efficently narrow down which group contains the spoiled the watermelon. This divide-and-conquer strategy reduces the necessary comparsions.
int n = 9;
int watermelon[n] = {1, 1, 1, 1, 1, 1, 1, 2, 1};
int basket_A = watermelon[0] + watermelon[1] + watermelon[2];
int basket_B = watermelon[3] + watermelon[4] + watermelon[5];
int basket_C = watermelon[6] + watermelon[7] + watermelon[8];
if (basket_A == basket_B) {
// Spoiled watermelon is in basket C
if (watermelon[6] == watermelon[7])
return 8;
else if (watermelon[6] < watermelon[7])
return 7;
else
return 6;
}
else if (basket_A > basket_B) {
// Spoiled watermelon is in basket A
if (watermelon[0] == watermelon[1])
return 2;
else if (watermelon[0] < watermelon[1])
return 1;
else
return 0;
}
else {
// Spoiled watermelon is in basket B
if (watermelon[3] == watermelon[4])
return 5;
else if (watermelon[3] < watermelon[4])
return 4;
else
return 3;
}
This approach is faster in theory, especially as the number of items increases — offering a balance of logic and efficiency over brute-force looping.
đź§ Conclusion
At first glance, Solution 1 — the simple for loop — seems ideal. It’s minimal, readable, and easy to understand. In just a few lines, it solves the problem and locates the spoiled watermelon. But as problems grow in complexity or scale, this simplicity can become a limitation.
Solution 2 — on the other hand, is longer and seemingly more complicated. It requires splitting the data into logical groups(baskets), applying conditional checks, and writing more lines of code. However, what you gain is efficiency, structure, foresight. This method reduces comparisons and performs intelligent by narrowing down the search scope using basic divide-and-conquer logic — something that scales far better for larger datasets.
KeyTakeways🗝️
Code isn’t just about solving a problem — it’s about solving it well. Longer code can mean :
Better readability, with each logical step made explicit.
Easier debugging and maintenance, especially in collaborative environments.
Enhanced performance, when the logic is optimized for efficiency.
Greater scalability, ready to handle future requirements.
Subscribe to my newsletter
Read articles from Rahul Dev directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
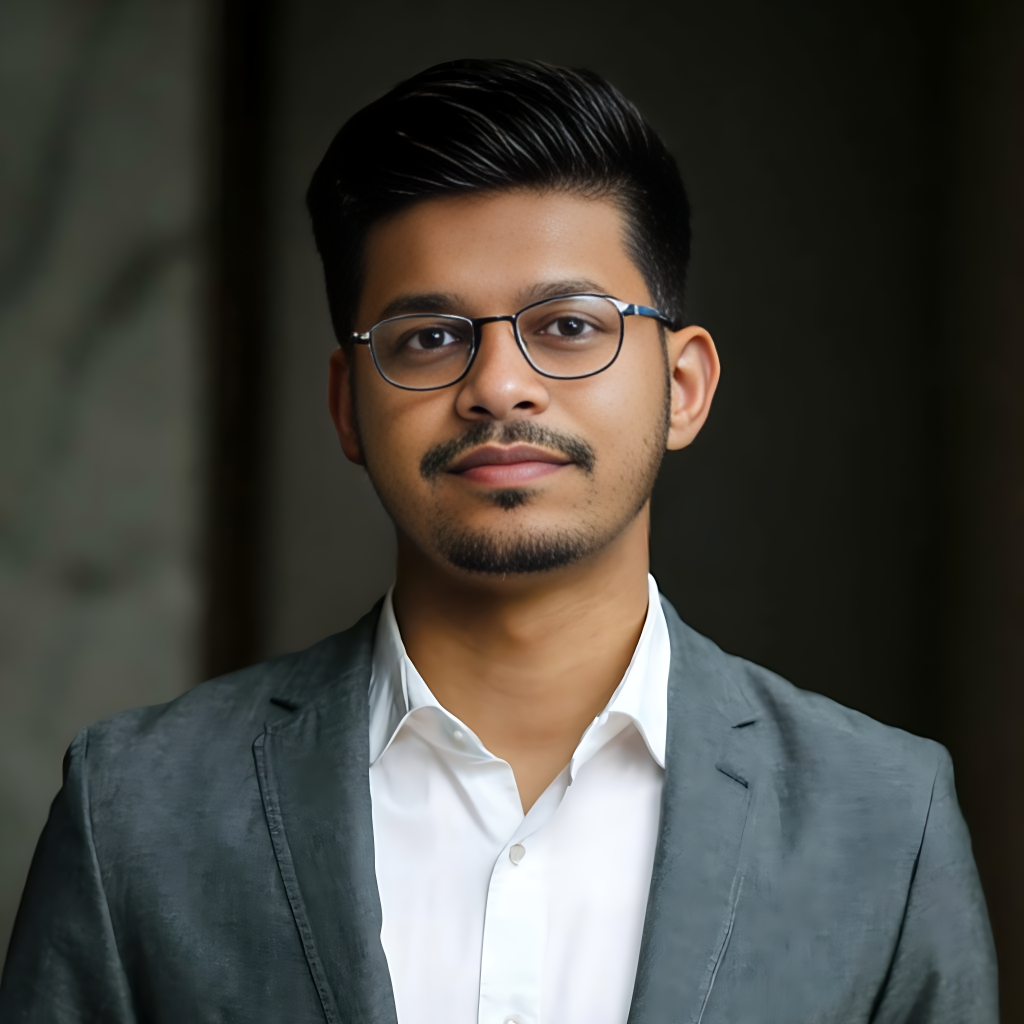