Git Commands & Workflows: Most of Us(Devs) Never/Rarely Use!
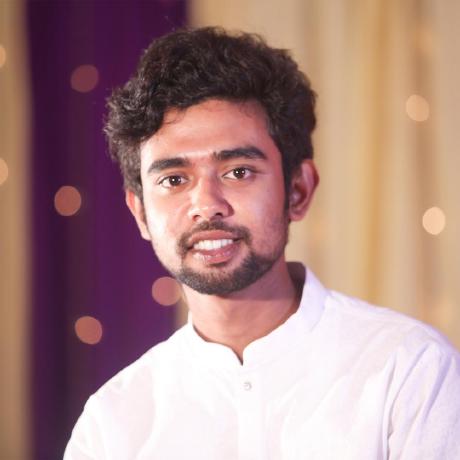

A compact deep dive into the power of Git & GitHub
🗂 Table of Contents
Intro: Snapshots, Not Diffs
Git Workflows: Find Your Flow
Git Internals: Inside Git’s Brain
Rare Used Git Commands: Commands You (Should) Use
Debugging with Git: Trace, Hunt & Find
Visualizing Git: See the Tree, Not Just the Leaves
Rewriting History: Be Careful, But Powerful
Advanced Branching: Level Up Your Git Skills
GitHub Power-Ups: Supercharge Your Repo
Git Stats: Numbers Behind this
Pro Tips & Aliases: Efficient with Your Git Flow
Resources to Master Git: Tools and Practice
Final Thoughts: Go Beyond Just Using Git
Understanding Git is easier when you stop thinking of it as just commands, and start visualizing it as:
A snapshot-based system (not diff-based)
A tree of commits connected by references
Local vs Remote: You have your repo, and the origin (remote copy)
Think of HEAD as your camera view. Commits as photos, and branches as your album names.
👉 So when we say Git is snapshot-based, not diff-based, we mean: Git saves the full content of files (with optimization for unchanged files), instead of just the changes (diffs).
🚦 Git Workflows – Quick Overview
Git Flow:
main
+develop
+ feature/hotfix/release branches — great for large, structured teams.GitHub Flow:
main
+ short-lived feature branches — ideal for fast-paced, continuous delivery.Trunk-Based: Single
main
branch with rapid CI/CD — best for small teams or modern DevOps.Feature Toggles: Use flags to deploy incomplete features — avoid long-lived branches.
Forking Model: Contributors fork, then PR — perfect for open-source collaboration.
💡 Long-lived branches = merge nightmares. Keep branches short-lived and focused.
🌍 Remote Repos: origin
vs upstream
origin → Your repo's default remote (usually the one you cloned)
upstream → The original source repo you forked from (common in open source)
git remote add upstream <https://github.com/original/repo.git>
git fetch upstream
git rebase upstream/main
🎯 Use
origin
to push your changes. Useupstream
to stay synced with the original repo.
🧬 Git Internals (Simplified)
Git stores everything as objects:
blob
= file contentstree
= folder structurecommit
= snapshot + metadatatag
= reference to a commit
.git/
folder = your repo's entire brain (history, objects, refs)SHA-1 hashes = unique IDs for commits — Git is content-addressable and immutable
🧠 Understand the plumbing (like git cat-file, git hash-object) to debug weird issues or build Git-based tools.
Essential Yet Unused Git Commands
These commands are like developer superpowers—rarely used, but incredibly useful when wielded right.
🧰 Workflow Utilities
Speed up daily Git tasks with smart shortcuts and hidden gems.
Command | What it Does | When to Use It |
git stash | Temporarily shelves uncommitted changes | You need to switch branches without committing junk |
git stash pop | Reapplies stashed changes | Once you're ready to resume your previous task |
git cherry-pick <commit> | Applies a specific commit from one branch to another | When you want just that one fix |
git switch <branch> | Switches branches (modern alt to checkout ) | For cleaner, intuitive branch management |
git clean -fd | Removes untracked files and dirs | When your repo feels messy and full of temp files |
🧪 Debug & Investigate
Hunt bugs, trace changes, and understand who did what, when, and why.
Command | What it Does | When to Use It |
git bisect | Finds the commit that introduced a bug using binary search | Bug appeared somewhere between two commits—find the culprit fast |
git blame <file> | Shows who changed what and when | Understand context behind a line of code |
git show <commit> | Displays changes from a specific commit | Review exactly what a commit did |
git log -S'someCode' | Search commit history for code changes | Find when a specific piece of code was added/removed |
🧠 Git is not just version control—it's a time machine for code clarity.
📜 Visualizing History
See your project’s evolution at a glance—structure beats scrolling.
Command | What it Does | When to Use It |
git log --graph --oneline --all | Visual tree of all branches and commits | Quickly understand the branch structure |
git reflog | Shows every move your HEAD made | Recover a lost commit or undo that “oops” moment |
git diff | View changes between commits, branches, or the working directory | For side-by-side comparisons |
git shortlog -sn | Lists commit count per contributor | Handy for stats or code ownership insight |
✍️ Rewriting History (Use with care)
Rewriting history can clean things up—but on local branches only unless you know what you're doing.
Command | What it Does | Use it for... |
git commit --amend | Modify the last commit (message or staged files) | Fix typos or add missed changes |
git rebase -i HEAD~n | Interactively squash, reorder, or edit past commits | Cleanup commit history before pushing |
git reset --soft HEAD~1 | Undo last commit but keep changes staged | Rephrase your commit message |
git reset --hard HEAD~1 | Completely erase last commit and changes | Only when you're absolutely sure! |
git revert <commit> | Creates a new commit that undoes the changes | Safely “undo” a pushed commit on shared branches |
⚠️ Never rebase, reset, or amend on shared branches without team consent. Breaks shared history = chaos.
🔄 Advanced Branching Strategies (Optional Add-on)
Command | What it Does | Why Use It |
git worktree add <dir> <branch> | Checkout multiple branches simultaneously in separate folders | Ideal for testing or parallel dev work |
git merge --squash | Combine all changes into one commit without actual merge | Clean history from feature branches before merging |
git remote prune origin | Cleans up old remote-tracking branches | Helps keep your local repo tidy |
🚀 GitHub Superpowers
GitHub CLI (
gh
) – Manage PRs, issues, and repos straight from your terminal→
gh pr create
,gh issue list
Actions – Automate CI/CD with YAML workflows
→ Build, test, deploy on push or PR
Secrets – Securely store API keys and tokens for automation
Packages – Host Docker, npm, Maven packages right from your repo
Projects & Discussions – Lightweight tools for planning and collaboration
⚡ Pro Tip: Combine Actions + CLI + Secrets = DevOps magic inside your repo.
Git & GitHub Stats (with Sources)
Metric | Value | Source |
Git Commands | 150+ total (20 used daily) | Git Docs |
GitHub Users | 100M+ (as of 2024) | GitHub Blog |
Repositories Hosted | 420M+ | Wikipedia |
Commits Per Day | 35M+ | Coinlaw |
GitHub Actions (Daily Runs) | 4M+ | GitHub Changelog |
Annual Revenue | $1B+ (ARR) | CNBC |
GitHub Employees | ~5,600 (2024) | Wikipedia |
⚙️ Pro-Level Git Tips & Aliases
Add these to your ~/.gitconfig
to speed up common commands:
[alias]
co = checkout
br = branch
st = status
lg = log --oneline --graph --decorate --all
🔧 Config Tweaks for Better Dev Flow
Auto-correct typos
→
git config --global help.autocorrect 1
Use VS Code as default editor
→
git config --global core.editor "code --wait"
Colorful CLI output
→
git config --global color.ui auto
💡 Tip: Use aliases like git lg to visualize your branch history at a glance
Learning Resources & Tools to Level Up
⚒️ Visual Git Tools
Tools like GitKraken, Sourcetree, GitHub Desktop can help you understand Git flow visually.
Ideal for visual learners or for onboarding junior devs.
🧠 Practice Tools
learngitbranching.js.org – Interactive visualization of Git commands.
OhMyGit! – A game to learn Git.
Git Workflows – Understand Gitflow, trunk-based, GitHub Flow, etc.
Disclosure
Crafted with deep questions, multiple AI chats, lots of Googling, real-world Git practice, and verified through trusted docs. Still learning. Still refining.
🎯 Final Thoughts
Most developers use Git. Fewer truly understand it. Only a few actually leverage its full power.
Mastering Git isn’t about memorizing commands—it's about learning how Git thinks. Once you understand the internal model, embrace smart workflows, and tap into GitHub’s hidden superpowers, you’ll go beyond just writing code—you’ll collaborate, debug, and deliver like a pro.
📬 Like insights like this?
This post is part of the Developer Data newsletter — bite-sized deep dives into the tools you use every day but rarely master.
👉 https://developer-data.beehiiv.com/subscribe
👉 https://www.threads.com/@rajjon.dey
👉 Save it as your Git cheatbook
Happy Coding! See you in the next deep dive. ✌️
Subscribe to my newsletter
Read articles from Rajon Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
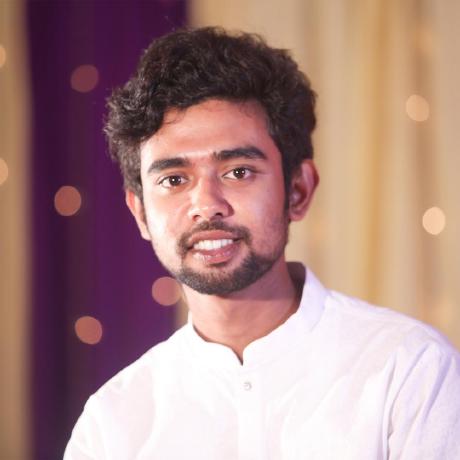
Rajon Dey
Rajon Dey
I'm a Software Developer, my passion is crafting smart, meaningful, and professional websites that enhance the online presence of individuals and businesses, making the web a more engaging, effective, and better platform. Get the data - https://developer-data.beehiiv.com/