An Introduction to TypeScript for JavaScript Developers
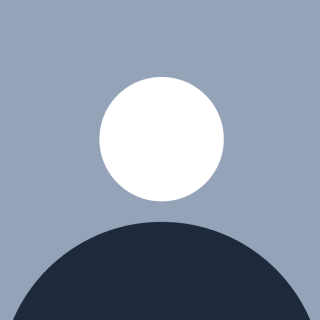

An Introduction to TypeScript for JavaScript Developers
TypeScript has rapidly become one of the most popular languages for web development, especially among JavaScript developers looking for better tooling, scalability, and maintainability. If you're a JavaScript developer curious about TypeScript, this guide will introduce you to its core concepts, benefits, and how to get started.
What is TypeScript?
TypeScript is a superset of JavaScript developed by Microsoft that adds static typing to the language. It compiles down to plain JavaScript, meaning it runs anywhere JavaScript does—browsers, Node.js, Deno, etc.
Unlike JavaScript, which is dynamically typed, TypeScript allows developers to define types for variables, function parameters, and return values. This leads to fewer runtime errors and better code predictability.
Why Use TypeScript?
Static Typing – Catches errors during development rather than runtime.
Enhanced IDE Support – Autocompletion, refactoring tools, and inline documentation.
Better Scalability – Makes large codebases easier to maintain.
Modern JavaScript Features – Supports ES6+ features while compiling to older JS versions for compatibility.
Getting Started with TypeScript
Installation
To use TypeScript, you’ll need Node.js installed. Then, install TypeScript globally via npm:
bash
Copy
Download
npm install -g typescript
Verify the installation with:
bash
Copy
Download
tsc --version
Writing Your First TypeScript Code
Create a file hello.ts
:
typescript
Copy
Download
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("TypeScript"));
Compile it to JavaScript:
bash
Copy
Download
tsc hello.ts
This generates hello.js
, which you can run with Node:
bash
Copy
Download
node hello.js
TypeScript Basics
Type Annotations
TypeScript introduces type annotations to specify the kind of value a variable holds:
typescript
Copy
Download
let age: number = 25;
let name: string = "Alice";
let isActive: boolean = true;
Interfaces
Interfaces define the structure of objects:
typescript
Copy
Download
interface User {
id: number;
name: string;
email?: string; // Optional property
}
const user: User = {
id: 1,
name: "John Doe"
};
Classes
TypeScript enhances JavaScript classes with type checking:
typescript
Copy
Download
class Person {
constructor(public name: string, private age: number) {}
greet() {
console.log(</span><span class="token string">Hello, my name is </span><span class="token interpolation"><span class="token interpolation-punctuation punctuation">${</span><span class="token keyword">this</span><span class="token punctuation">.</span>name<span class="token interpolation-punctuation punctuation">}</span></span><span class="token template-punctuation string">);
}
}
const person = new Person("Alice", 30);
person.greet();
Union and Intersection Types
Union Types (
|
) – A variable can be one of several types.Intersection Types (
&
) – Combines multiple types into one.
typescript
Copy
Download
type ID = number | string;
function printId(id: ID) {
console.log(</span><span class="token string">ID: </span><span class="token interpolation"><span class="token interpolation-punctuation punctuation">${</span>id<span class="token interpolation-punctuation punctuation">}</span></span><span class="token template-punctuation string">);
}
printId(101); // Works
printId("abc123"); // Also works
Generics
Generics allow reusable components that work with multiple types:
typescript
Copy
Download
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("Hello");
let numOutput = identity<number>(42);
Integrating TypeScript with JavaScript Projects
If you have an existing JavaScript project, you can gradually adopt TypeScript:
Rename
.js
files to.ts
and fix type errors.Use
allowJs
intsconfig.json
to mix JS and TS files.Add type definitions for third-party libraries using
@types
packages.
Example tsconfig.json
:
json
Copy
Download
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"strict": true,
"allowJs": true
},
"include": ["src/**/*"]
}
TypeScript with Frontend Frameworks
React + TypeScript
Create a React app with TypeScript:
bash
Copy
Download
npx create-react-app my-app --template typescript
Example React component:
typescript
Copy
Download
import React from 'react';
interface Props {
title: string;
count: number;
}
const Counter: React.FC<Props> = ({ title, count }) => {
return (
<div>
<h1>{title}</h1>
<p>Count: {count}</p>
</div>
);
};
export default Counter;
Node.js + TypeScript
Install dependencies:
bash
Copy
Download
npm install typescript ts-node @types/node --save-dev
Example server.ts
:
typescript
Copy
Download
import express from 'express';
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello TypeScript!');
});
app.listen(port, () => {
console.log(</span><span class="token string">Server running at http://localhost:</span><span class="token interpolation"><span class="token interpolation-punctuation punctuation">${</span>port<span class="token interpolation-punctuation punctuation">}</span></span><span class="token template-punctuation string">);
});
Conclusion
TypeScript brings strong typing, better tooling, and improved maintainability to JavaScript projects. Whether you're working on a small script or a large-scale application, TypeScript can help you write more reliable code.
If you're ready to dive deeper, check out the official TypeScript documentation.
And if you're looking to grow your YouTube channel, consider using MediaGeneous, a powerful platform for social media promotion and marketing.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by