Python Basics: Variables, Loops & Functions Explained Simply
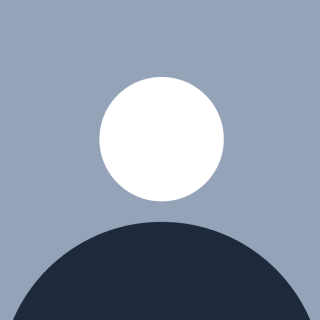

๐น Introduction to Python Programming
Python is like the cool friend who can suddenly make everything easier. Whether one is creating a calculator, automating mundane gestures, or simply dreaming of developing their own game, Python is often the first language anyone can turn to. But why?
๐ Join our full Python course to start learning step by step.
Being Python-Friendly for a Beginner
Because it has a clean readable syntax without a lot of techno babble. Python feels like English. You do not have to memorize some wild symbols or worry about setup-heavy jargon. It is just simple and direct, yet powerful.
What Can You Build with Python?
Python builds web apps, machine learning models, multimedia dashboards, even robots. Instagram, Dropbox, and Netflix all speak
Python. Now, that is a cool thing!
๐น Getting Started with Python
How to Install Python
To install Python and start coding, go to python.org. Click on download and install it just as you would install any application. Make sure you check the box that says "Add Python to PATH!"
Writing Your First Python Program
Open the terminal or IDLE, and type now:
python
print("Hello, world!")
Hit Enter. Boom! You just wrote your first Python program!
๐น Understanding Variables in Python.
What Are Variables?
Think of variables as containers; they contain data that you may want to access later. Think of it like a jar with a label- the variable stands for something we can retrieve and reuse.
python
name = "Alice"
age = 25
Here, name holds "Alice," and age holds 25.
Python Variable Naming Rules
Start with a letter or underscore (_)
Cannot begin with a number
Use snake_case for readability
Be descriptive: user_age is better than x
Different Data Types in Python
Python variables can store:
Integers: 5
Floats: 3.14
Strings: "hello"
Booleans: True, False
Lists: [1, 2, 3]
Dictionaries: {"name": "Alice", "age": 25}
๐น Introduction to Loops in Python
What Are Loops?
They let you execute the same set of commands repeatedly without having to copy the code a hundred times.
For Loop in Python
Perfect when you know exactly how many times to repeat something.
python
for i in range(5):
print("Loop number",i)
While Loop in Python
Perfect for when you donโt really know quite how many times youโre going to do something โ you simply go on until a state-of- affairs has been met.
python
x = 0
while x < 5:
print(x)
x += 1
Loop Control Statements
break: terminates loop execution prematurely
continue: skips all statements in the current iteration and jumps to the next iteration of the loop
pass: does nothing; it is used as a placeholder
Also Read: Why Is Python A Good Programming Language For Beginners
๐น Python Functions Made Easy
What Is a Function?
A function is a reusable block of code. It's like a recipe, one written once and used whenever a situation arises.
def greet():
print("Hello!")
Built-in Vs User-Defined Functions
Built-in: Already present, like print(), len(), and type()
User Defined: Created by you via def.
How to Define a Function
python
def say_hello(name):
print("Hello", name)
Call it using say_hello("Alice")
Function Parameters and Return Values
You can pass information into a function and possibly get a result back:
python
def add(a, b):
return a + b
๐น Practical Examples of Variables, Loops & Functions
Simple Calculator Using Functions
python
def add(a, b):
return a + b
print(add(10, 5))
Looping through a List of Names
python
names= ["Alice", "Bob", "Charlie"]
for name in names:
print("Hello", name)
Using Variables to Keep Score
python
score = 0
score += 10
print("Your score is", score)
๐น Common Mistakes Beginners Make
Variable Name Confusion
name and Name can be entirely different variables; Python is case-sensitive.
Infinite Loops
If you forget to update a variable used in a while loop, the program might never terminate.
Forget Return Statements
If a function is not specified with return, no value is returned; it simply performs an action.
๐น Tips to Improve Your Python Skills
Practice Small Projects
Start with building a calculator, to-do app, or number guessing game.
Read Others' Code
Check out GitHub, follow tutorials, and watch how others approach a problem.
Use Online Platforms for Coding Practice
Try HackerRank, LeetCode, or Codecademy to get your practice and challenges.
Conclusion
Python is a beginner's best friend, especially once you understand the concepts of variables, loops, and functions. These concepts form the basis of almost every program. You will use these tools at every stage, from printing 'Hello World' to building a weather app.
So go ahead, code, break things, fix things, and most importantly, have fun with it. Python is always there for you!
๐ Want to go beyond Python? Check out our full programming course library and take the next step in your coding journey.
At TCCI, we don't just teach computers โ we build careers. Join us and take the first step toward a brighter future.
Location: Bopal & Iskcon-Ambli in Ahmedabad, Gujarat
Call now on +91 9825618292
Visit Our Website: http://tccicomputercoaching.com/
Note: This Post was originally published on https://tccicomputercoaching.wordpress.com/2025/05/07/python-basics-variables-loops-functions-explained-simply/ and is shared here for educational purposes.
Subscribe to my newsletter
Read articles from TCCI Computer Coaching directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by