Android Services: How They Work and Why They Matter
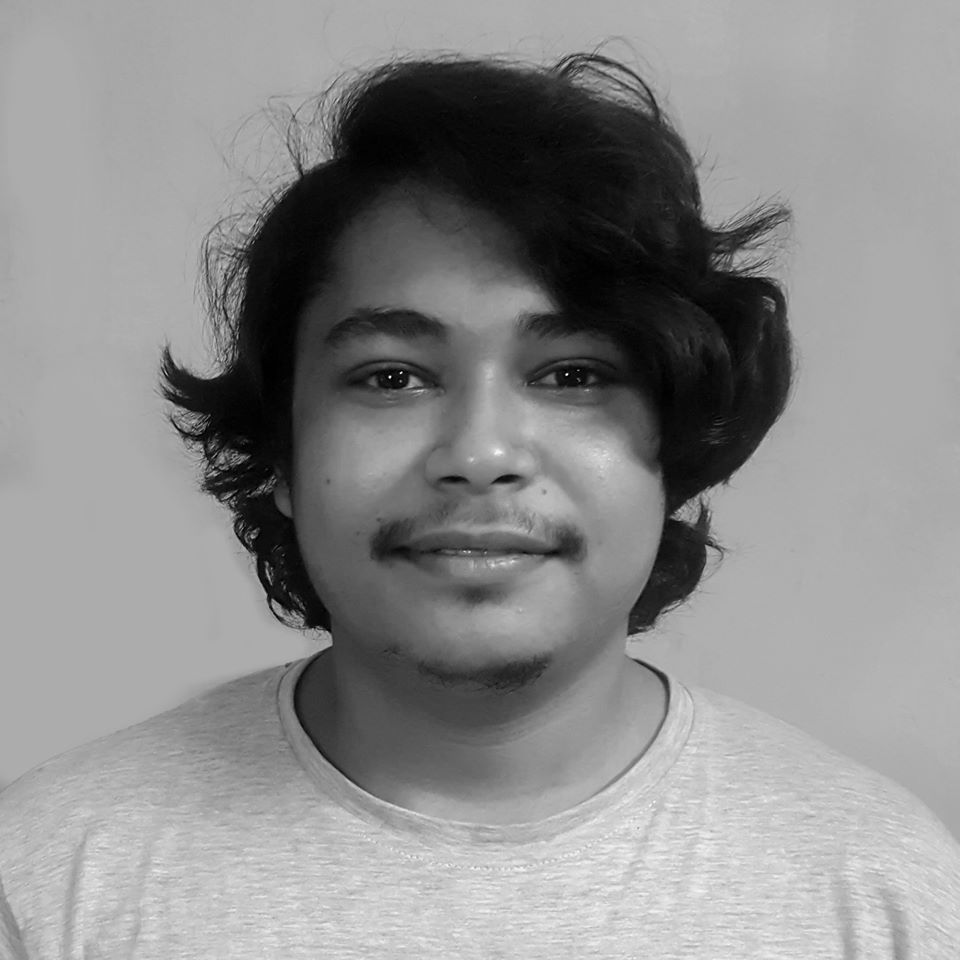
Table of contents
- Prologue: The Android Service Ecosystem
- 1. Core System Operations
- 2. Hardware Controllers
- 3. Connectivity & Communication
- 4. Security & Permissions
- 5. Media & Projection
- 6. Background & Scheduled Tasks
- 7. Niche & Specialized Services
- 8. System Utilities
- Why So Many Services?
- Final Thoughts

Prologue: The Android Service Ecosystem
Imagine your Android device as a bustling city. Apps are like citizens, requesting resources, communicating with each other, and performing tasks. But who keeps everything in order? Who makes sure the lights stay on, the Wi-Fi connects, and notifications arrive on time? Meet the Android Service Classes — the unsung heroes (or "managers") that act as intermediaries between apps and the underlying hardware or system resources.
In this technical storytelling guide, we’ll explore how these services work, their roles, and real-world examples. The code snippet you provided maps Android’s XXXManager
classes to their corresponding system service constants. Let’s decode them!
1. Core System Operations
These services are the "operating system’s backbone," handling processes, hardware, and user interactions.
ActivityManager
(ACTIVITY_SERVICE
)Role: Manages app lifecycles (starting/stopping activities, killing processes).
Example: When you press the "Back" button, it decides which app screen to show.
WindowManager
(WINDOW_SERVICE
)Role: Controls window stacking, animations, and screen overlays.
Example: Floating chat heads in Facebook Messenger.
PowerManager
(POWER_SERVICE
)Role: Manages wake locks to keep the CPU/screen active.
Example: YouTube keeps the screen on while you watch a video.
AlarmManager
(ALARM_SERVICE
)Role: Schedules tasks to run at precise times (even when the app is closed).
Example: A calendar app reminding you of a meeting at 3 PM.
UserManager
(USER_SERVICE
)Role: Manages multi-user profiles on a device (e.g., "Work" vs. "Personal" modes).
Example: Switching profiles on a shared family tablet.
StorageManager
(STORAGE_SERVICE
)Role: Manages storage volumes (internal, SD cards) and file access.
Example: Files app showing available storage space.
2. Hardware Controllers
These services act as "translators" between apps and physical hardware.
CameraManager
(CAMERA_SERVICE
)Role: Detects and configures camera hardware.
Example: Instagram switching between front and rear cameras.
SensorManager
(SENSOR_SERVICE
)Role: Provides access to motion, environmental, and position sensors.
Example: A compass app using the magnetometer.
BluetoothManager
(BLUETOOTH_SERVICE
)Role: Discovers and pairs Bluetooth devices.
Example: Connecting a smartwatch to your phone.
ConsumerIrManager
(CONSUMER_IR_SERVICE
)Role: Controls infrared (IR) blasters on older devices.
Example: Using your phone as a TV remote.
Vibrator
(VIBRATOR_SERVICE
)Role: Triggers haptic feedback (vibrations).
Example: Your phone buzzing when you receive a text.
DisplayManager
(DISPLAY_SERVICE
)Role: Manages multiple displays (e.g., casting to a TV).
Example: Mirroring your screen to a Chromecast.
3. Connectivity & Communication
These services handle networking, telephony, and data exchange.
ConnectivityManager
(CONNECTIVITY_SERVICE
)Role: Monitors network states (Wi-Fi, mobile data, VPNs).
Example: Netflix checking if you’re on Wi-Fi before streaming.
TelephonyManager
(TELEPHONY_SERVICE
)Role: Manages cellular network operations (calls, SMS, SIM info).
Example: Your dialer app showing "Calling..." during a call.
WifiManager
(WIFI_SERVICE
)Role: Scans for Wi-Fi networks and manages connections.
Example: Automatically connecting to your home Wi-Fi.
NsdManager
(NSD_SERVICE
)Role: Discovers services on a local network (Network Service Discovery).
Example: Chromecast appearing in YouTube’s cast menu.
WifiP2pManager
(WIFI_P2P_SERVICE
)Role: Enables peer-to-peer Wi-Fi Direct connections.
Example: Sharing files directly between phones without the internet.
4. Security & Permissions
These services enforce privacy and device policies.
DevicePolicyManager
(DEVICE_POLICY_SERVICE
)Role: Applies enterprise policies (e.g., mandatory encryption).
Example: Your company requiring a 6-digit PIN to access work emails.
AppOpsManager
(APP_OPS_SERVICE
)Role: Tracks app permissions at a granular level.
Example: Android’s "Permission Manager" showing which apps used your mic.
KeyguardManager
(KEYGUARD_SERVICE
)Role: Manages the lock screen (PIN, fingerprint, face unlock).
Example: Disabling the lock screen temporarily for a trusted Bluetooth device.
AccessibilityManager
(ACCESSIBILITY_SERVICE
)Role: Supports accessibility features (e.g., screen readers).
Example: TalkBack reading aloud text for visually impaired users.
5. Media & Projection
These services handle audio, video, and screen sharing.
AudioManager
(AUDIO_SERVICE
)Role: Controls volume levels and audio routing.
Example: Your phone switching to silent mode during a meeting.
MediaRouter
(MEDIA_ROUTER_SERVICE
)Role: Routes media playback to external devices (e.g., Chromecast).
Example: Casting a YouTube video to your TV.
MediaProjectionManager
(MEDIA_PROJECTION_SERVICE
)Role: Captures screen content (requires user consent).
Example: Recording gameplay footage with AZ Screen Recorder.
MediaSessionManager
(MEDIA_SESSION_SERVICE
)Role: Coordinates media playback across apps (play, pause, skip).
Example: Controlling Spotify from your smartwatch.
6. Background & Scheduled Tasks
These services manage work that happens behind the scenes.
JobScheduler
(JOB_SCHEDULER_SERVICE
)Role: Optimizes background tasks (e.g., runs only when charging).
Example: Google Photos backing up photos overnight.
DownloadManager
(DOWNLOAD_SERVICE
)Role: Manages large downloads (resumes after network failures).
Example: Downloading a movie from Netflix for offline viewing.
UsageStatsManager
(USAGE_STATS_SERVICE
)Role: Tracks app usage statistics (requires special permission).
Example: Digital Wellbeing showing your daily screen time.
7. Niche & Specialized Services
These handle unique scenarios or lesser-known features.
RestrictionsManager
(RESTRICTIONS_SERVICE
)Role: Enforces app restrictions (e.g., parental controls).
Example: Kids’ apps blocking in-app purchases.
LauncherApps
(LAUNCHER_APPS_SERVICE
)Role: Manages app shortcuts and home screen widgets.
Example: Long-pressing an app icon to reveal "App Shortcuts."
TvInputManager
(TV_INPUT_SERVICE
)Role: Controls TV inputs on Android TV devices.
Example: Switching between HDMI and Netflix on a smart TV.
CaptioningManager
(CAPTIONING_SERVICE
)Role: Manages closed captioning settings.
Example: Enabling subtitles in YouTube videos.
UiModeManager
(UI_MODE_SERVICE
)Role: Switches UI modes (e.g., night mode, car mode).
Example: Your phone enabling dark mode at sunset.
TextServicesManager
(TEXT_SERVICES_MANAGER_SERVICE
)Role: Manages spell checkers and input methods.
Example: Gboard’s autocorrect feature.
SubscriptionManager
(TELEPHONY_SUBSCRIPTION_SERVICE
)Role: Handles SIM/eSIM subscriptions (Android 5.0+).
Example: Switching between mobile data plans on a dual-SIM phone.
8. System Utilities
Miscellaneous but critical services.
LayoutInflater
(LAYOUT_INFLATER_SERVICE
)Role: Converts XML layouts into UI components.
Example: Rendering a custom dialog in your app.
ClipboardManager
(CLIPBOARD_SERVICE
)Role: Manages copy-paste operations.
Example: Copying a link from Chrome and pasting it into WhatsApp.
DropBoxManager
(DROPBOX_SERVICE
)Role: Logs system debug information (not the cloud storage app!).
Example: Crash logs stored for developer debugging.
InputMethodManager
(INPUT_METHOD_SERVICE
)Role: Switches between keyboards (Gboard, SwiftKey).
Example: Tapping a text field to bring up the keyboard.
InputManager
(INPUT_SERVICE
)Role: Handles global input events (key presses, touch gestures).
Example: Detecting a long-press on the home button.
Why So Many Services?
Android’s modular design delegates responsibilities to avoid "god classes." Each service:
Encapsulates complexity: Apps don’t need to reinvent Wi-Fi scanning or battery management.
Enforces permissions: Access to sensitive data (location, camera) is gatekept.
Optimizes resources: Background tasks are batched to save battery.
Final Thoughts
Next time you use your phone, imagine these services as silent stagehands in a theater:
The
Vibrator
service buzzes your pocket.The
NotificationManager
lights up your screen.The
LocationManager
guides you via GPS.
Without them, your apps would be like actors without a stage! 🎭
Pro Tip: Always check the Android version when using a service (e.g.,
JobScheduler
works only on Android 5.0+). UseBuild.VERSION.SDK_INT
to avoid crashes!
That’s it for today, Happy Coding…
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
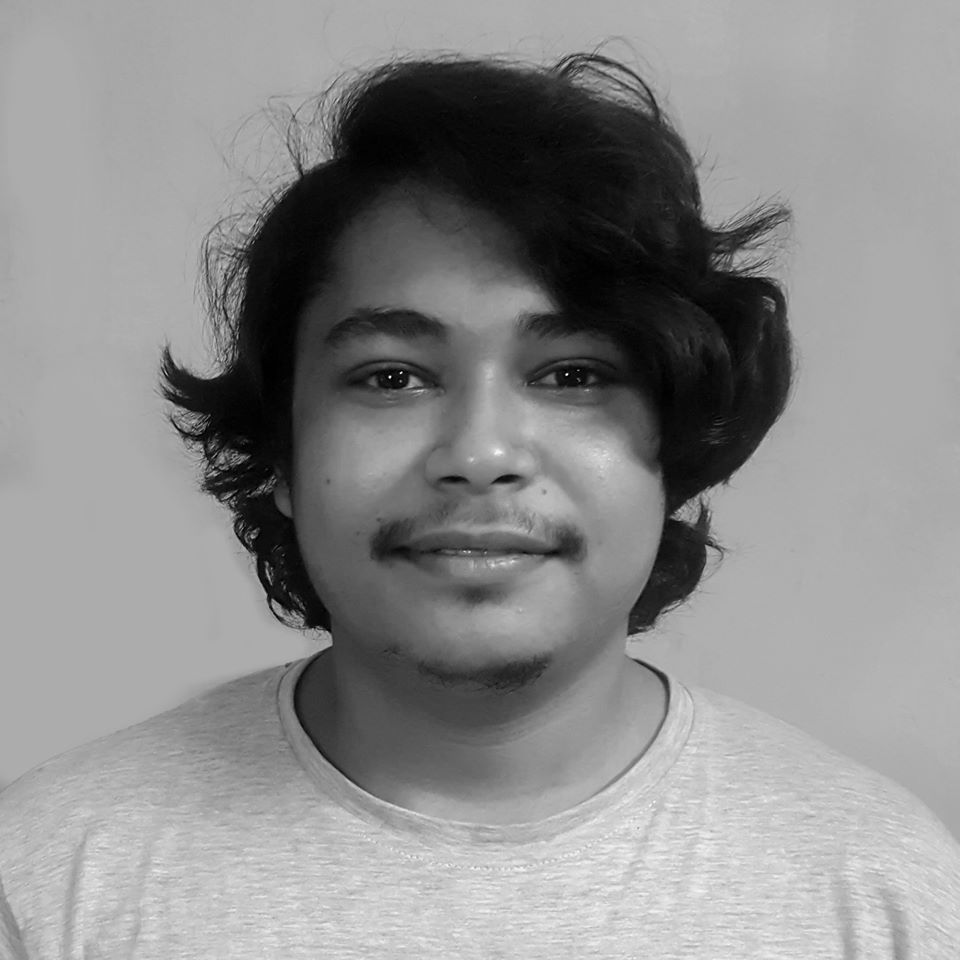
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.