Learning Swift: The Basics of iOS Development
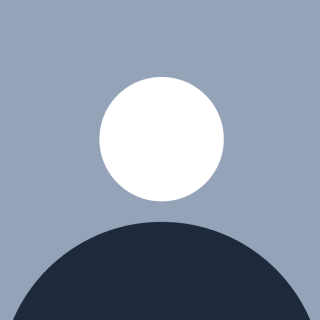

Learning Swift: The Basics of iOS Development
If you're looking to dive into iOS development, Swift is the language you need to learn. Developed by Apple, Swift is powerful, intuitive, and designed specifically for building apps across Apple’s ecosystem—iOS, macOS, watchOS, and tvOS. In this guide, we’ll cover the fundamentals of Swift and how you can start building your first iOS app.
Why Learn Swift?
Swift is the primary language for iOS development, offering several advantages:
Fast & Efficient: Swift is optimized for performance, often outperforming Objective-C.
Easy to Read: Its clean syntax makes it beginner-friendly.
Safe: Features like optionals and type safety reduce runtime crashes.
Modern: Swift includes powerful features like closures, generics, and protocol-oriented programming.
If you're serious about iOS development, mastering Swift is essential.
Setting Up Your Development Environment
Before writing any code, you’ll need:
A Mac: Xcode, Apple’s IDE, only runs on macOS.
Xcode: Download it for free from the Mac App Store.
An Apple Developer Account (Optional for beginners): Sign up at developer.apple.com.
Once installed, open Xcode and create a new Playground (for practice) or an iOS Project (for app development).
Swift Basics: Syntax & Core Concepts
1. Variables & Constants
Swift uses var
for variables and let
for constants.
swift
Copy
Download
var name = "John" // Mutable
let age = 25 // Immutable
2. Data Types
Swift is type-safe, meaning you must declare data types explicitly or let Swift infer them.
swift
Copy
Download
var score: Int = 100
var price: Double = 9.99
var isActive: Bool = true
var message: String = "Hello, Swift!"
3. Optionals
Optionals (?
) handle the absence of a value.
swift
Copy
Download
var optionalName: String? = "Alice"
print(optionalName!) // Force unwrapping (risky)
// Safe unwrapping
if let name = optionalName {
print(name)
}
4. Functions
Functions are declared with func
.
swift
Copy
Download
func greet(name: String) -> String {
return "Hello, \(name)!"
}
print(greet(name: "Bob"))
5. Control Flow
Swift supports standard if-else
, switch
, and loops.
swift
Copy
Download
// If-else
if score > 80 {
print("Great job!")
} else {
print("Keep trying!")
}
// For loop
for i in 1...5 {
print(i)
}
Building Your First iOS App
Let’s create a simple "Hello, World!" app.
Step 1: Create a New Xcode Project
Open Xcode → File → New → Project
Select iOS → App → Click Next
Name your project (e.g., "HelloWorld") → Choose Swift & Storyboard → Click Next
Step 2: Design the UI
Open Main.storyboard
Drag a Label and a Button onto the screen.
Use Auto Layout to position them.
Step 3: Connect UI to Code
Open ViewController.swift
Add an IBAction for the button:
swift
Copy
Download
@IBAction func showMessage(_ sender: UIButton) {
let alert = UIAlertController(
title: "Welcome",
message: "Hello, iOS Developer!",
preferredStyle: .alert
)
alert.addAction(UIAlertAction(title: "OK", style: .default))
present(alert, animated: true)
}
- Ctrl+Drag from the button to the code to link the action.
Step 4: Run the App
Press ⌘ + R to launch the app in the Simulator.
Next Steps in iOS Development
Once you’re comfortable with Swift basics, explore:
UIKit vs. SwiftUI: Apple’s two main frameworks for building interfaces.
Networking: Fetching data from APIs using URLSession.
Core Data: Storing data locally.
Publishing Your App: Learn about the App Store submission process.
Growing as a Developer
Learning Swift is just the beginning. If you’re documenting your journey on YouTube, consider promoting your channel to reach a wider audience. A great platform for social media growth is MediaGeneous, which helps creators boost their visibility through effective marketing strategies.
Conclusion
Swift is a versatile and powerful language for iOS development. By mastering the basics—variables, functions, optionals, and UIKit—you’ll be well on your way to building amazing apps. Keep practicing, explore Apple’s official Swift documentation, and join developer communities like Swift Forums to stay updated.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by