Maps vs Sets in JavaScript: Organizing Data the Right Way
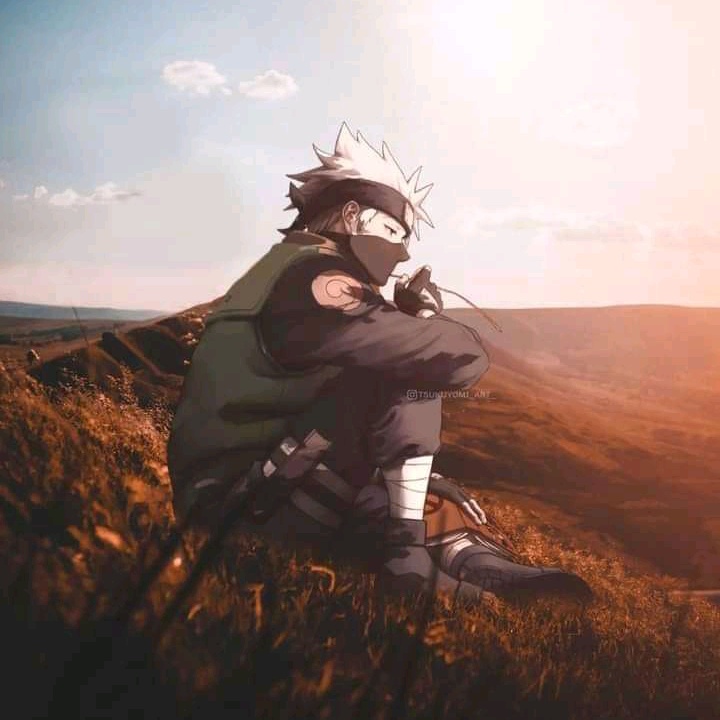

When it comes to handling data collections in JavaScript, arrays and objects have long been our go-to tools.
However, as applications have grown more complex, JavaScript has introduced two specialized data structures: Maps and Sets.
Both offer powerful, nuanced ways to organize and interact with data but they each solve slightly different problems.
In this article, we’ll dive deep into Maps and Sets, understand their syntax, differences, and see how they can simplify real-world projects.
What is a Map?
A Map is a collection of key-value pairs where:
Any type of value (objects, functions, primitives) can be used as a key.
Keys maintain the order of insertion.
Maps provide efficient, predictable access to values based on their keys.
Basic Syntax
const userRoles = new Map();
// Setting values
userRoles.set('Alice', 'Admin');
userRoles.set('Bob', 'Editor');
// Getting a value
console.log(userRoles.get('Alice')); // Output: Admin
// Checking existence
console.log(userRoles.has('Bob')); // Output: true
// Deleting a key
userRoles.delete('Bob');
// Size of the map
console.log(userRoles.size); // Output: 1
Real-World Example: Storing User Permissions
Imagine you're building an admin dashboard where users can have different roles.
Instead of using a plain object, a Map gives you more flexibility, especially when keys might not be simple strings.
const permissions = new Map();
const user1 = { name: 'Alice' };
const user2 = { name: 'Bob' };
permissions.set(user1, 'admin');
permissions.set(user2, 'editor');
console.log(permissions.get(user1)); // Output: admin
Why use a Map here?
Because if you used an object, JavaScript would coerce the keys (objects) into strings ("[object Object]"
), causing conflicts.
Maps allow actual objects as keys without that issue 😉.
What is a Set?
A Set is a collection of unique values, where:
Duplicate entries are automatically removed.
Values maintain insertion order.
Useful for ensuring uniqueness without extra manual checks.
Basic Syntax
const colors = new Set();
// Adding values
colors.add('red');
colors.add('blue');
colors.add('red'); // Duplicate! Will be ignored
console.log(colors.size); // Output: 2
console.log(colors.has('blue')); // Output: true
// Deleting a value
colors.delete('blue');
console.log(colors.has('blue')); // Output: false
Real-World Example: Tracking Unique Tags
Suppose you're building a blog and want to collect unique tags from articles.
const allTags = new Set();
function addTags(tagsArray) {
tagsArray.forEach(tag => allTags.add(tag));
}
addTags(['javascript', 'web', 'javascript', 'frontend']);
console.log([...allTags]); // Output: ['javascript', 'web', 'frontend']
Why use a Set here?
You don’t need to manually check if a tag already exists.
Sets handle duplicates automatically!
Commonly Used Methods
Here’s a quick reference table for the most commonly used methods for Maps and Sets:
Map Methods
Method | Purpose |
set(key, value) | Adds a key-value pair to the map |
get(key) | Retrieves the value associated with a key |
has(key) | Checks if a key exists in the map |
delete(key) | Removes a key and its value |
clear() | Removes all key-value pairs |
size | Returns the number of entries |
Set Methods
Method | Purpose |
add(value) | Adds a unique value to the set |
has(value) | Checks if a value exists in the set |
delete(value) | Removes a value from the set |
clear() | Removes all values from the set |
size | Returns the number of unique values |
Comparing Maps and Sets
Feature | Map | Set |
Stores | Key-value pairs | Unique values |
Duplicate Handling | Keys must be unique | Values must be unique |
Key Types | Any type (objects, functions) | Only values (no keys) |
Use Case | Fast lookups, object-keyed data | Ensuring uniqueness, filtering |
Practical Tip: When to Use Which?
Use Maps when you need to associate data with specific identifiers (keys), especially when keys are not strings.
Use Sets when you want to manage a collection of unique items, like tags, users online, or selected items.
Key Takeaways
Maps provide a flexible way to associate keys to values, they are great for complex key lookups.
Sets ensure a collection of items remains unique.
Both structures offer better performance and cleaner code in cases where arrays and objects would otherwise require additional logic.
Conclusion
Maps and Sets may seem like small additions to the JavaScript toolkit, but they offer nuanced improvements over traditional data structures.
They help you organize data more effectively, write cleaner code, and optimize performance without complicated workarounds.
This article is a part of the Nuances in Web Development series, where we dive deep into subtle but important concepts that sharpen your development skills. you can check out previous articles here and be sure you subscribe to our newsletter.
Subscribe to my newsletter
Read articles from Peter Bamigboye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
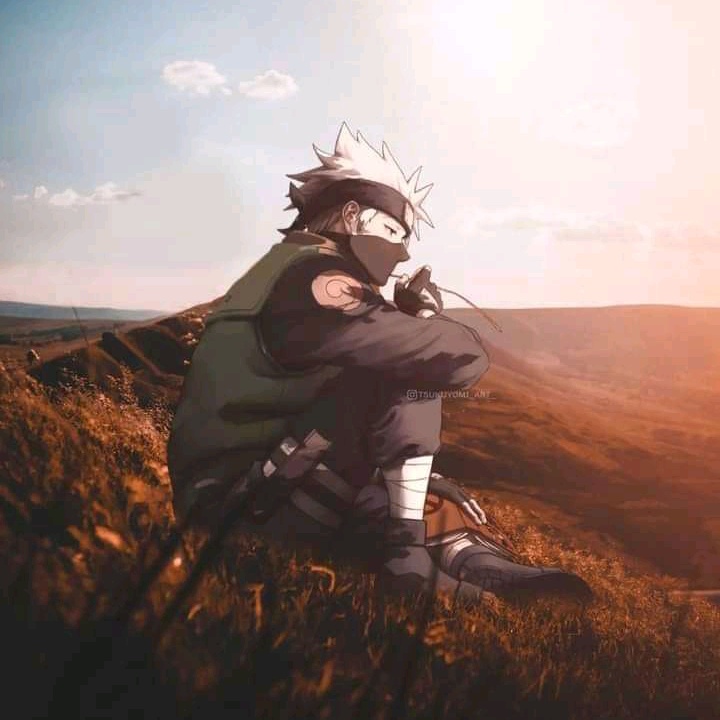
Peter Bamigboye
Peter Bamigboye
I am Peter, a front-end web developer who writes on current technologies that I'm learning or technologies that I feel the need to simplify.