Step-by-Step Guide to Connecting Third-Party APIs with WordPress
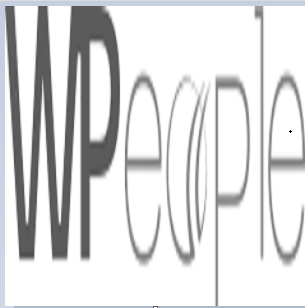
In today’s digital ecosystem, APIs (Application Programming Interfaces) are the backbone of integration. Whether you're pulling social media content, fetching real-time weather data, or connecting to a CRM system, integrating third-party APIs can supercharge your WordPress site’s functionality. But where do you start?
This comprehensive, step-by-step guide will walk you through the process of connecting third-party APIs to your WordPress site using multiple methods—from using built-in functions to writing custom code.
What Is a Third-Party API?
A third-party API is an external interface provided by a service that allows developers to retrieve or send data between systems. These APIs are commonly used to:
- Display external data (like weather, stock prices, or social feeds)
- Automate tasks (e.g., syncing contacts to a CRM)
- Enable features (e.g., payment processing, geolocation, or live chat)
Why Connect a Third-Party API to WordPress?
By connecting your WordPress site to a third-party API, you can:
- Expand your site’s features without reinventing the wheel
- Provide dynamic and real-time content
- Improve user experience through personalization
- Streamline business workflows and integrations
Common use cases include:
- Integrating Stripe or PayPal for payments
- Displaying Instagram or Twitter feeds
- Pulling data from a public database or open API
- Syncing form submissions with tools like Mailchimp or HubSpot
Step-by-Step: How to Connect a Third-Party API to WordPress
Let’s break it down into practical, actionable steps.
Step 1: Choose the Right API for Your Use Case
Start by identifying what you want to achieve.
Examples:
- Display weather data? → Use OpenWeatherMap API
- Payment processing? → Use Stripe API
- Social media feed? → Use Instagram Graph API
Make sure to:
- Read the API documentation carefully
- Understand authentication requirements (API key, OAuth, tokens)
- Check rate limits and pricing if applicable
Step 2: Get Your API Credentials
Most APIs require authentication for use. This usually involves signing up for the service and generating an API key or token.
Common authentication methods:
- API key: A unique string sent in the request
- Bearer token: Often used with OAuth2
- Client ID/Secret: Used for secure applications
Tip: Keep these credentials private. Never expose them in your frontend code.
Step 3: Decide How You’ll Make API Requests in WordPress
There are multiple ways to send API requests. Let’s focus on the most commonly used and recommended method:
Use the WordPress HTTP API
WordPress provides built-in functions for making HTTP requests:
- wp_remote_get() for GET requests
- wp_remote_post() for POST requests
Why use it?
- Built-in to WordPress
- Handles errors and responses properly
- Works well with caching plugins and filters
Step 4: Make a Test Request (Example: Fetching Weather Data)
Let’s say you’re using the OpenWeatherMap API to get the current weather for London.
Add the following code to your theme’s functions.php file or a custom plugin:
php
CopyEdit
function fetch_weather_data() {
$api_key = 'your_api_key_here';
$city = 'London';
$url = "https://api.openweathermap.org/data/2.5/weather?q={$city}&appid={$api_key}&units=metric";
$response = wp_remote_get($url);
if (is_wp_error($response)) {
return 'Unable to fetch weather data.';
}
$body = wp_remote_retrieve_body($response);
$data = json_decode($body);
if (isset($data->main->temp)) {
return 'Current temperature in ' . $city . ': ' . $data->main->temp . '°C';
}
return 'Weather data not available.';
}
To display the data on a page or post, create a shortcode:
php
CopyEdit
add_shortcode('weather_info', 'fetch_weather_data');
Then use [weather_info] in your post content or widget.
Step 5: Display API Data in Your Theme
Instead of using shortcodes, you can also inject API data directly into your theme files. Example:
php
CopyEdit
<?php echo fetch_weather_data(); ?>
Place it anywhere in your header.php, page.php, or a custom template.
Step 6: Handle API Errors Gracefully
You should always check for:
- Network errors
- Invalid responses
- Missing data fields
- Rate limits or quota issues
Use fallback values or display a user-friendly message when an error occurs:
php
CopyEdit
if (is_wp_error($response) || empty($data)) {
return 'Service temporarily unavailable. Please try again later.';
}
Step 7: Cache the API Response (Optional but Recommended)
To avoid exceeding rate limits or slowing down your site, cache the API response using WordPress transients:
php
CopyEdit
function fetch_weather_data_cached() {
$cache_key = 'weather_data_london';
$data = get_transient($cache_key);
if (false === $data) {
$api_key = 'your_api_key';
$city = 'London';
$url = "https://api.openweathermap.org/data/2.5/weather?q={$city}&appid={$api_key}&units=metric";
$response = wp_remote_get($url);
if (is_wp_error($response)) {
return 'Error fetching data.';
}
$body = wp_remote_retrieve_body($response);
$data = json_decode($body);
set_transient($cache_key, $data, 3600); // cache for 1 hour
}
return 'Temperature: ' . $data->main->temp . '°C';
}
Step 8: Securing Your API Integration
- Never expose API keys in frontend JavaScript.
- Store keys in wp-config.php or use environment variables.
- Use HTTPS for all API requests.
- Sanitize and validate any user input used in API calls.
Example: Store key in wp-config.php
php
CopyEdit
define('OPENWEATHER_API_KEY', 'your_api_key');
Then use it like:
php
CopyEdit
$api_key = OPENWEATHER_API_KEY;
Alternative Methods for API Integration
Depending on your preference or project scope, you can also explore:
1. Using a Plugin
Many popular APIs have dedicated plugins (e.g., Mailchimp, Stripe, Zapier). These offer:
- Easy integration
- Built-in settings panels
- Minimal coding required
2. JavaScript Fetch API
For frontend use (like loading dynamic content without refreshing the page):
javascript
CopyEdit
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById('output').innerHTML = data.title;
});
You’ll need to handle CORS issues and avoid exposing API keys.
3. Custom REST API Endpoints
Create your own endpoints in WordPress to serve or process external data:
php
CopyEdit
add_action('rest_api_init', function () {
register_rest_route('custom/v1', '/status', [
'methods' => 'GET',
'callback' => function() {
return ['status' => 'ok'];
}
]);
});
Useful for headless WordPress or decoupled frontend apps.
Common Pitfalls and How to Avoid Them
Pitfall | Solution |
Exposing API keys in the frontend | Store securely in PHP, not JS |
No caching → API rate limits | Use transients or object cache |
Bad user experience on API failure | Display fallback content |
Complex JSON parsing | Use json_decode() and test with sample responses |
Final Thoughts
Connecting third-party APIs to your WordPress website is easier than ever with WordPress’s built-in HTTP functions, plugins, and shortcode capabilities. Whether you're a beginner adding weather data to a blog or a developer building a SaaS-powered plugin, this integration can drastically enhance your site's functionality and automation potential.
Start small, test thoroughly, and always keep security and performance in mind.
Subscribe to my newsletter
Read articles from WPeopleOfficial directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
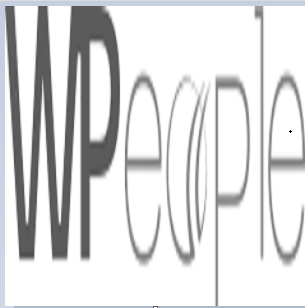
WPeopleOfficial
WPeopleOfficial
WPeople is a leading Custom WordPress Web Development Company across the globe, we specializes in creating tailored digital web solutions to help businesses thrive online. With a team of expert WordPress developers, designers, we are committed to delivering top-notch custom WordPress web development solutions that not only meet but exceed our clients' expectations. https://wpeople.net/