A Beginner’s Guide to Cron Jobs and NodeMailer in Node.js (Part 1)
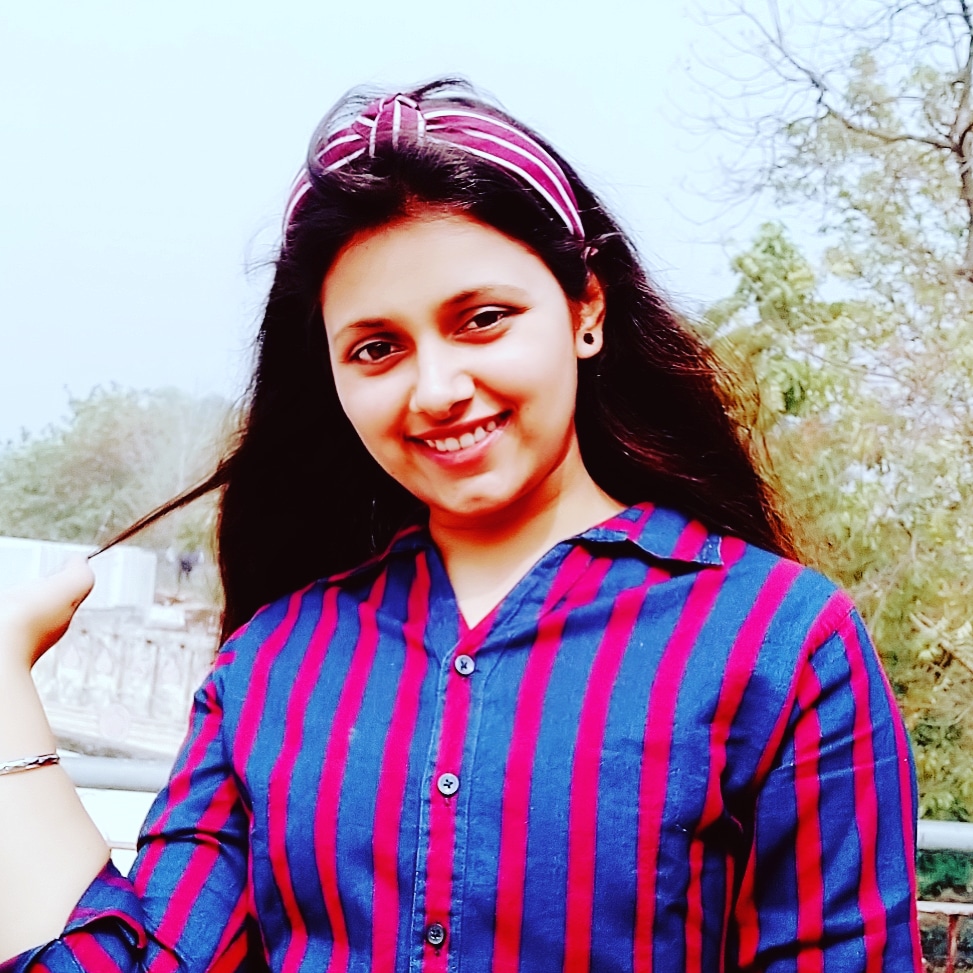

A Beginner’s Guide to Cron Jobs and NodeMailer in Node.js (Part 1)
In modern web applications, automation plays a crucial role in improving efficiency. Tasks like sending scheduled emails, cleaning up old database records, or running periodic reports can be automated using cron jobs. Additionally, sending emails (like password reset links or notifications) is a common requirement, which can be achieved using NodeMailer.
In this blog post (Part 1), we will cover:
What are Cron Jobs?
Setting Up Cron Jobs in Node.js
Using Cron Jobs with Express & MongoDB
Cron Expression Format Explained
Best Practices for Cron Jobs
In Part 2, we will dive into NodeMailer and how to integrate it with Express and MongoDB for sending emails.
1. What Are Cron Jobs?
Definition
A cron job is a scheduled task that runs automatically at specified intervals. The term "cron" comes from Unix-based systems, where a cron daemon runs tasks in the background at predefined times.
Use Cases in Node.js Applications
Sending automated emails (daily reports, notifications).
Cleaning up old database records (e.g., expired sessions, temporary files).
Generating periodic reports (weekly analytics, monthly summaries).
Backing up databases at scheduled times.
2. Setting Up Cron Jobs in Node.js
To use cron jobs in Node.js, we can use the node-cron package.
Installation
First, install the package:
npm install node-cron
Basic Usage
Here’s a simple example that runs a task every minute:
const cron = require("node-cron");
// Runs every minute
cron.schedule("* * * * *", () => {
console.log("Cron job executed at:", new Date());
});
* * * * *
is a cron expression that defines the schedule (we’ll explain this later).The callback function runs whenever the scheduled time is reached.
3. Using Cron Jobs with Express & MongoDB
Cron jobs become even more powerful when combined with Express (for HTTP servers) and MongoDB (for database operations).
Example: Deleting Expired Records in MongoDB
Suppose we have a Task
model where tasks expire after 24 hours. We can set up a cron job to automatically delete old tasks every hour.
Step 1: Define the Mongoose Model
const mongoose = require("mongoose");
// Define a Task model with auto-expiry
const Task = mongoose.model(
"Task",
new mongoose.Schema({
name: String,
createdAt: { type: Date, default: Date.now, expires: "1d" }, // Auto-delete after 1 day
})
);
Step 2: Schedule the Cron Job
const cron = require("node-cron");
// Runs every hour at the 0th minute (e.g., 1:00, 2:00, etc.)
cron.schedule("0 * * * *", async () => {
try {
const result = await Task.deleteMany({
createdAt: { $lt: new Date(Date.now() - 24 * 60 * 60 * 1000) },
});
console.log("Old tasks deleted:", result.deletedCount);
} catch (error) {
console.error("Error deleting old tasks:", error);
}
});
0 * * * *
means "Run at minute 0 of every hour."$lt
(less than) selects records older than 24 hours.
Step 3: Full Express + MongoDB + Cron Job Example
const express = require("express");
const mongoose = require("mongoose");
const cron = require("node-cron");
const app = express();
// Connect to MongoDB
mongoose.connect("mongodb://localhost:27017/mydb");
// Define Task model (same as above)
const Task = mongoose.model("Task", ...);
// Schedule cron job (same as above)
cron.schedule("0 * * * *", async () => { ... });
// Start Express server
app.listen(3000, () => console.log("Server running on port 3000"));
4. Cron Expression Format Explained
Cron jobs follow a specific syntax:
* * * * * <command>
| | | | |
| | | | └── Day of the week (0-7, 0 or 7 = Sunday)
| | | └──── Month (1-12)
| | └────── Day of the month (1-31)
| └──────── Hour (0-23)
└────────── Minute (0-59)
Examples
Expression | Meaning |
*/5 * * * * | Every 5 minutes |
0 12 * * * | Every day at 12:00 PM |
0 0 * * 1 | Every Monday at midnight |
0 0 1 * * | First day of every month at midnight |
5. Best Practices for Cron Jobs
Avoid Overloading the Database
Optimize queries (e.g., use indexing).
Run jobs during low-traffic hours.
Log Cron Job Executions
- Helps debug failures.
cron.schedule("* * * * *", () => {
console.log("Job ran at:", new Date());
});
Use Process Managers (PM2)
- Ensures cron jobs keep running even if the app crashes.
pm2 start server.js
Keep Cron Jobs Separate
- Store them in a separate file (
/jobs/cleanup.js
) instead of mixing with routes.
- Store them in a separate file (
What’s Next? (Part 2 Preview)
In Part 2, we will cover:
✅ What is NodeMailer?
✅ Setting Up Email Sending in Node.js
✅ Password Reset Functionality with NodeMailer + MongoDB
✅ Best Practices for Sending Emails
Stay tuned! 🚀
Final Thoughts
Cron jobs are a powerful way to automate repetitive tasks in Node.js applications. By combining them with Express and MongoDB, you can build efficient, self-maintaining systems.
🔹 Got questions? Drop them in the comments!
🔹 Enjoyed this guide? Share it with fellow developers!
Subscribe to my newsletter
Read articles from Anjali Saini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
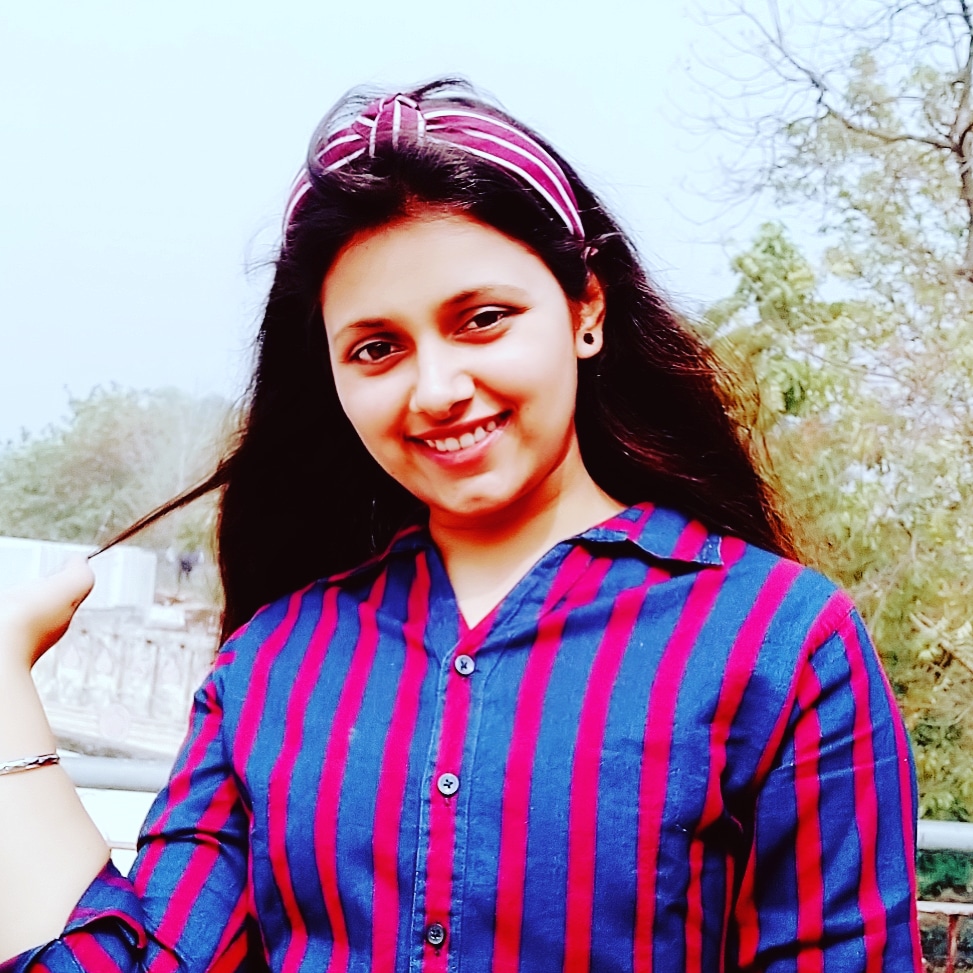
Anjali Saini
Anjali Saini
I am an Enthusiastic and self-motivated web-Developer . Currently i am learning to build end-to-end web-apps.