What is the difference between map() and forEach() in JavaScript?
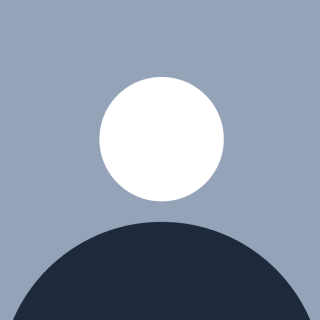
As a web developer, you'll often work with arrays. Two powerful tools — map()
and forEach()
— help you loop through them.
This guide breaks down what they do, when to use them, and how to avoid costly mistakes — with clean syntax, real-world use cases, and interview-ready clarity.
What is map()?
The map() method creates a new array by transforming every element in the original array using a callback function.
📌 Think of it as:
“I want a new version of this array, based on some change to each item.”
✅ Syntax:
array.map((currentValue, index, array) => {
// return transformed value
});
✅ Example:
const numbers = [1, 2, 3, 4];
const squares = numbers.map(num => num * num);
console.log(squares); // [1, 4, 9, 16]
What is forEach()
?
The forEach()
method runs a function on each element of the array — but does not return a new array.
📌 Think of it as:
“I want to do something with each item, but I don’t need a result array.”
✅ Syntax:
array.forEach((currentValue, index, array) => {
//perform action
});
✅ Example:
const numbers = [1, 2, 3];
numbers.forEach(num => {
console.log(`Current number: ${num}`);
});
map()
vs forEach()
– What's the Difference?
Feature | map() | forEach() |
Returns New Array | ✅ Yes | ❌ No |
Use Case | Transform data (create a new version) | Perform side effects (e.g., logging) |
Chainable | ✅ Yes | ❌ No |
Real-World Use Cases
✅ Use map()
when:
You’re transforming API data
Creating new arrays for UI (e.g., dropdowns, lists)
Example
const prices = [100, 200, 300];
const withTax = prices.map(price => price * 1.18);
console.log(withTax); // [118, 236, 354]
✅ Use forEach()
when:
You’re logging or validating each item
Making API requests one by one
You’re pushing items into another array.
Example :
const users = ['Alice', 'Bob', 'Charlie'];
users.forEach(user => {
console.log(`Sending welcome email to ${user}`);
});
Common Mistakes
Using
forEach()
when you expect a return value:const result = [1,2,3].forEach(num => num * 2); console.log(result); // undefined ❌
Forgetting that
map()
always returns a new array, even if you don’t use it:[1, 2, 3].map(num => console.log(num)); // map is used here just like forEach ❌
Quick Interview Tip
❓ What’s the main difference between map() and forEach()?
✅
map()
returns a new array with transformed values,forEach()
just executes a function and returns nothing.
Self-Test Challenge
Convert this forEach()
code to use map()
:
const names = [];
const users = ['Alice', 'Bob'];
users.forEach(user => names.push(user.toUpperCase()));
console.log(names); // ['ALICE', 'BOB']
Correct version using map()
:
const users = ['Alice', 'Bob'];
const names = users.map(user => user.toUpperCase());
console.log(names); // ['ALICE', 'BOB']
Conclusion: When to Use Each
Use map()
when:
- You need a new array with transformed data.
✅ Use forEach()
when:
- You just want to perform an action on each item.
💡 Remember:map()
= transform + returnforEach()
= do something, no return
// Original
const nums = [1, 2, 3];
// Using map()
const squares = nums.map(n => n * n);
// Result: [1, 4, 9]
// Using forEach()
nums.forEach(n => console.log(n * n));
// No result array — just logs
Subscribe to my newsletter
Read articles from Mamta Paswan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by