Building a Multi-Page Application with React Router
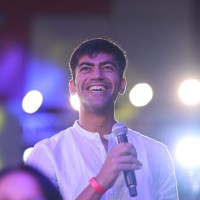
React Router is a powerful routing library built on top of React that helps you add new screens and flows to your application incredibly quickly, all while keeping the URL in sync with what's being displayed on the page.
React manipulates the DOM by injecting components dynamically, creating the illusion of multiple pages while maintaining a single page structure. React Router isn't just about matching a URL to a function or component: it's about building a full user interface that maps to the URL.
In this blog, we will talk about single-page applications (SPAs) and demonstrates how to implement routing with React Router DOM.
What is SPAs ?
Single Page Applications (SPAs) in React use a single page to manage navigation without refreshing. React manipulates the DOM by injecting components dynamically, creating the illusion of multiple pages while maintaining a single page structure. This is crucial for SPAs to function smoothly
We have to install React Router in our react project in order to use them. Use following command on your project file terminal.
npm install react-router-dom
Now you will import the necessary components from react-router-dom in your application.
import ReactDOM from "react-dom/client";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import Layout from "./pages/Layout";
import Home from "./pages/Home";
import Contact from "./pages/Contact";
export default function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<Layout />}>
<Route index element={<Home />} />
<Route path="contact" element={<Contact />} />
</Route>
</Routes>
</BrowserRouter>
);
}
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
Layout.js
import { Outlet, Link } from "react-router-dom";
const Layout = () => {
return (
<>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Outlet />
</>
)
};
export default Layout;
Home.js
const Home = () => {
return <h1>Home</h1>;
};
export default Home;
Contact.js
const About = () => {
return <h1>About</h1>;
};
export default About;
In v6.4, new routers were introduced that support the new data APIs:
createBrowserRouter
createMemoryRouter
createHashRouter
createStaticRouter
The following routers do not support the data APIs:
<BrowserRouter
>
:Uses the HTML5 history API for standard web apps.<
HashRouter>
:Uses hash-based routing for static servers.<MemoryRouter>
:Uses in-memory routing for testing and non-browser environments.<StaticRouter>
:Provides static routing for server-side rendering (SSR).
The above components will create browser, hash, memory and static history instances. React Router v6 makes the properties and methods of the history instance associated with your router available through the context in the router object.
createHashRouter is part of the React Router library and provides routing capabilities for single-page applications (SPAs). It's commonly used for building client-side navigation within applications.
Conclusion
React Router allows you to perform single-page routing without reloading the page. This enables faster user experiences because the browser doesn't need to request an entirely new document or re-evaluate CSS and JavaScript assets for the next page. It also enables more dynamic user experiences with things like animation.
Resources
Subscribe to my newsletter
Read articles from Piyush Nanwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
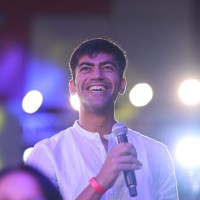
Piyush Nanwani
Piyush Nanwani
Experienced full-stack developer with a passion for tackling complex challenges. Dedicated to helping you become a top 1% mobile app developer. Passionate about mentoring, teaching, and continuous learning in new technologies.