How to create Single Page Application in React
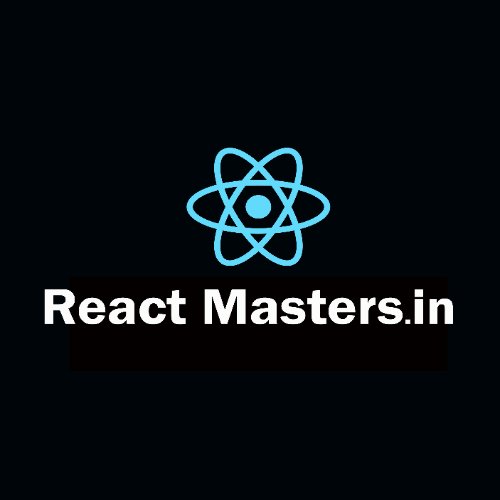
A Single Page Application (SPA) is a modern web application that loads a single HTML page and dynamically updates the content without refreshing the entire page. SPAs offer a seamless user experience, faster navigation, and better performance.
One of the most popular technologies for building SPAs is React JS, a JavaScript library developed by Meta (Facebook).
In this article, youโll learn how to create a simple SPA using React, along with routing, component creation, and project structuring. This guide is perfect for beginners who want to understand how SPAs work in a React environment.
๐ ๏ธ Prerequisites
Before getting started, make sure you have:
Basic knowledge of JavaScript and React
Node.js and npm installed on your system
A code editor like VS Code
๐ฆ Step 1: Set Up the React Project
Use Create React App to quickly scaffold your SPA.
Command:
bashCopyEditnpx create-react-app my-spa
cd my-spa
npm start
This sets up a basic React project with all the necessary configurations.
๐๏ธ Step 2: Understand the Project Structure
pgsqlCopyEditmy-spa/
โ
โโโ public/
โโโ src/
โ โโโ App.js
โ โโโ index.js
โ โโโ components/
โ โโโ Home.js
โ โโโ About.js
โ โโโ Contact.js
App.js
โ Main component that includes routing logiccomponents/
โ Contains all the reusable components
๐ฆ Step 3: Install React Router
To enable navigation between different pages without full reloads, install React Router.
Command:
bashCopyEditnpm install react-router-dom
๐งญ Step 4: Set Up Routing
Open App.js
and configure routes using react-router-dom
.
Sample Code (App.js):
javascriptCopyEditimport React from 'react';
import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
function App() {
return (
<Router>
<div>
<nav>
<Link to="/">Home</Link> |
<Link to="/about">About</Link> |
<Link to="/contact">Contact</Link>
</nav>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</div>
</Router>
);
}
export default App;
๐งฑ Step 5: Create Component Pages
Inside src/components
, create individual files:
Home.js
javascriptCopyEditimport React from 'react';
function Home() {
return <h2>Welcome to the Home Page</h2>;
}
export default Home;
About.js
javascriptCopyEditimport React from 'react';
function About() {
return <h2>About Us</h2>;
}
export default About;
Contact.js
javascriptCopyEditimport React from 'react';
function Contact() {
return <h2>Contact Page</h2>;
}
export default Contact;
๐จ Step 6: Add Basic Styling (Optional)
You can style your SPA using CSS or Tailwind CSS. Add styles in App.css
or apply inline styles for simplicity.
โ Step 7: Test Your SPA
Now, run your app using:
bashCopyEditnpm start
Youโll see your SPA with a navigation menu. Click through the links โ content updates without a full page reload!
๐ Additional Tips
Use 404 or fallback routes with
*
path to handle unknown URLs.Integrate state management tools like Redux or Context API for complex apps.
Consider code splitting for performance optimization in large SPAs.
Use React Helmet for managing SEO-related metadata dynamically.
Conclusion
Building a Single Page Application with React is a rewarding experience, especially for beginners looking to build modern, fast, and responsive web apps. With react-router-dom
, reusable components, and a solid project structure, you can scale your SPA with ease.
Start small, practice routing and component creation, and explore advanced features as you grow!
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
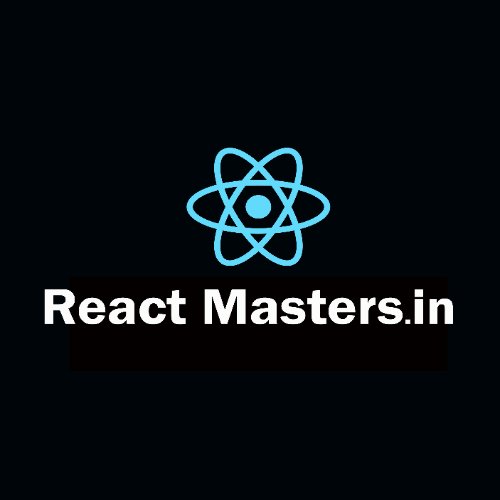
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.