Getting Started with Go: A Beginner’s Guide
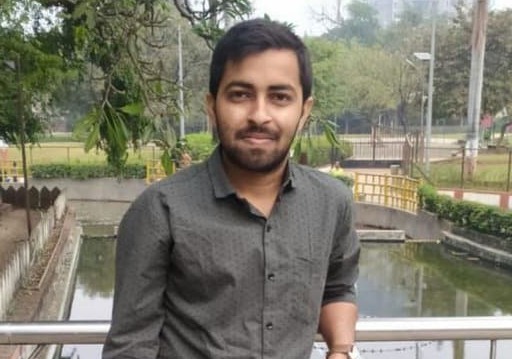
Introduction
Go (or Golang) is a simple, powerful, and fast programming language created by Google. It’s particularly popular for web servers, cloud software, DevOps, and concurrent applications. If you’re coming from JavaScript, Python, or another language, you’ll find Go’s minimalism refreshing and quickly get productive!
In this post, you’ll learn the essential Go concepts, see how Go compares to other languages, and write your first simple program. Ready? Let’s dive in!
1. Installing Go
Download Go from the official website.
Install and set up your
PATH
as instructed.Test your installation:
go version
2. Your First Go Program
Let’s print “Hello, World!”:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Save the code as main.go
, then run:
go run main.go
3. Key Concepts in Go
a. Simple Syntax
Every Go program starts with a
package
and amain
function.You import standard or third-party packages.
b. Variables and Types
var age int = 30
name := "Alice" // short form, Go infers the type
c. Control Structures
for i := 0; i < 5; i++ {
fmt.Println(i)
}
if age > 18 {
fmt.Println("Adult")
} else {
fmt.Println("Minor")
}
4. Functions
Go functions are simple and can return multiple values.
func add(a int, b int) int {
return a + b
}
result := add(2, 3) // result is 5
Multiple return values:
func swap(a, b string) (string, string) {
return b, a
}
5. Slices and Maps
Dynamic arrays are called slices:
numbers := []int{1, 2, 3, 4}
numbers = append(numbers, 5)
for i, num := range numbers {
fmt.Println(i, num)
}
Dictionaries are maps:
ages := map[string]int{"Bob": 25, "Alice": 30}
fmt.Println(ages["Alice"])
6. Structs and Methods
Structs let you group related data; methods give them behavior.
type Person struct {
Name string
Age int
}
func (p Person) Greet() {
fmt.Println("Hello, my name is", p.Name)
}
p := Person{Name: "Raj", Age: 28}
p.Greet()
7. Error Handling
Go handles errors explicitly—no exceptions!
import "strconv"
num, err := strconv.Atoi("123")
if err != nil {
fmt.Println("Conversion failed:", err)
} else {
fmt.Println(num)
}
8. Simple Web Server
Want to build a web server? Check this out:
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello from Go web server!")
})
http.ListenAndServe(":8080", nil)
}
Run, then visit http://localhost:8080 in your browser!
9. Where to Learn More
A Tour of Go — interactive Go lessons
Go by Example — concise code examples
Subscribe to my newsletter
Read articles from Ramdas Hedgapure directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
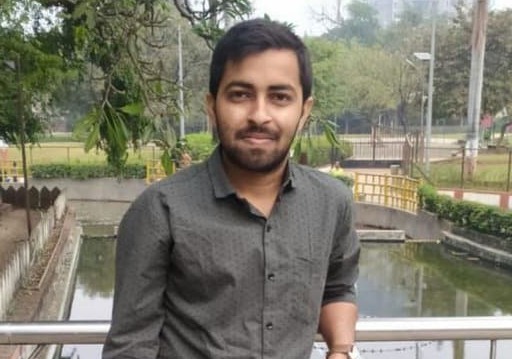