That Time I Thought a Row Could Fix Everything (Spoiler: It Didn’t)
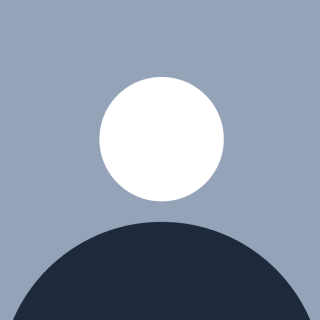
Table of contents
- Rows, Columns, and Boxes, Oh My!
- When You Need to Scroll (But Don’t Want to Cry)
- ConstraintLayout and FlowRow: When the Simple Stuff Fails You
- The Composable That Runs the Show: Scaffold
- Going Off the Grid: Custom Layouts
- Why Intrinsic Measurement Sounds Fancy (But Isn’t That Scary)
- Taking It to the Next Level: Pro Tips for Layout Performance
- The Secret Most Tutorials Won’t Tell You

Okay, confession time: when I first started using Jetpack Compose, I thought I could just slap a few Rows and Columns together and call it a layout. Boom. Done.
Yeah… no. That lasted about an hour before my UI turned into a jumbled mess of overlapping elements and scroll views that didn’t scroll.
Turns out, layout in Compose isn’t just about structure — it’s about behavior, intention, and understanding what each layout type brings to the table.
Let’s unpack that. No XML required. 😎
Rows, Columns, and Boxes, Oh My!
These are your bread and butter. But they do very different things.
Row = horizontal alignment (think buttons in a toolbar)
Column = vertical stacking (think input fields in a form)
Box = layering things on top of each other (think background + text overlay)
Compose gives you alignment and arrangement parameters — Arrangement is how children are spaced along the main axis. Alignment is how they line up across it.
Imagine a Column as a bookshelf. Arrangement controls how books are spaced top to bottom. Alignment controls whether they're left-justified, centered, or right-aligned on each shelf.
Get these mixed up, and you’ll wonder why your UI looks like it’s auditioning for abstract art.
When You Need to Scroll (But Don’t Want to Cry)
Let’s say you need a scrollable list. Please don’t use Column + verticalScroll()
and call it a day.
Instead, say hello to the Lazy layouts:
LazyColumn = vertical scrollable list
LazyRow = horizontal scrollable list
LazyVerticalGrid = scrollable grid (Pinterest-style vibes)
Why “lazy”? Because only what’s visible gets composed. Everything else? Skipped until needed.
It’s like a dinner buffet where food only appears when someone walks up with a plate. Efficient. Delicious. Zero waste.
Bonus: you can use key
in Lazy items to prevent unnecessary recomposition when your list updates. Learned that one the hard way after my list reordered itself every time I blinked.
ConstraintLayout and FlowRow: When the Simple Stuff Fails You
I resisted ConstraintLayout for a while. It reminded me too much of XML. But honestly? Sometimes it’s just what you need.
When your UI has overlapping rules, dependent alignments, or too much nesting with Rows/Columns, ConstraintLayout brings structure back to the chaos.
Then there’s FlowRow and FlowColumn, which auto-wrap content when space runs out.
Think: a dynamic list of filter chips or buttons that wrap like tags in Gmail. You can do it manually. But you’ll cry.
The Composable That Runs the Show: Scaffold
Scaffold is basically the stage manager of your UI. It handles things like:
App bars
Floating action buttons
Snackbars
Bottom nav bars
You bring the actors. Scaffold sets the stage.
Trust me — don’t try to manually space your content around a top bar and a floating action button. Been there. Looked terrible.
Going Off the Grid: Custom Layouts
Sometimes none of the built-ins will cut it.
That’s when you reach for the big guns: Custom Layouts and layout modifiers.
Let’s say you want to position an element based on the size of another. You can use the layout
modifier to measure, reposition, and customize layout behavior of a single child.
For more advanced cases, Compose gives you:
SubcomposeLayout
— lets you defer part of the composition until after measuring another partBoxWithConstraints
— lets you read parent constraints before children are composed
Just be warned: subcomposition is powerful, but heavy. Don’t use it unless your UI genuinely depends on another component’s size.
Why Intrinsic Measurement Sounds Fancy (But Isn’t That Scary)
Intrinsic measurement lets parents ask children: "Hey, how big would you like to be before I measure you?"
Helpful for cases like making all items in a Column the width of the widest child.
But again — don’t abuse it. It’s a pre-measure step, not a magic fix. Compose does provide default intrinsic size behavior, but you can override it in custom layouts for finer control.
Taking It to the Next Level: Pro Tips for Layout Performance
Avoid recomposition traps – Don’t shove changing logic into your layout.
Don’t read and write the same state during composition. Trust me, it’ll bite you.
Use
derivedStateOf
to cache expensive computations based on changing state.Always test performance in Release mode — Debug mode is deceptively slow.
And for Lazy lists:
Use
key
to avoid full list recomposition.Load only what's necessary. On-demand rendering is your best friend.
The Secret Most Tutorials Won’t Tell You
Layout isn’t just about how things look — it’s about how they behave when your app grows.
That’s what finally clicked for me.
I stopped treating layouts as pixel-level puzzles and started thinking about them as systems. Systems that should scale.
And once I embraced that, my Compose UIs finally started to feel... well, composed.
Your Turn 🎤
What’s the most frustrating layout bug you’ve hit in Compose? Or is there a layout type that still feels like black magic?
Drop it in the comments — I want to hear your war stories.
Let’s suffer and learn together. 😅
Subscribe to my newsletter
Read articles from Kavearhasi Viswanathan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by