Next.js Authentication: Client or Server? Comparing Hooks, Protected Routes & Middleware
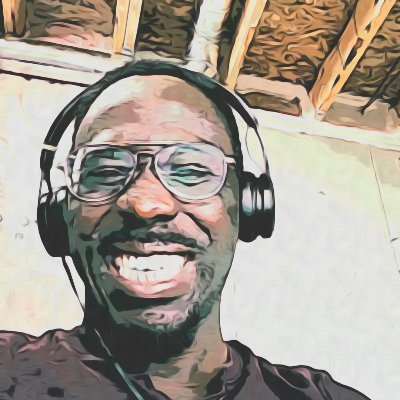

Authentication is fundamental. In Next.js, with its hybrid rendering capabilities, deciding how and where to implement authentication is a critical architectural choice. Do you handle it primarily on the client after the page loads, or do you enforce it on the server before anything sensitive reaches the browser?
As frontend engineers building complex applications, we need to understand the trade-offs. This article dives deep into Client-Side Authentication (CSA) versus Server-Side Authentication (SSA) within the Next.js ecosystem. We'll compare these core strategies and then examine the common design patterns associated with each – namely useAuth
hooks, ProtectedRoute
components, and the powerful Next.js Middleware. Let's weigh the options to build secure and user-friendly experiences.
The Big Picture: Client-Side vs. Server-Side Auth in Next.js
Before looking at specific code patterns, let's understand the fundamental difference between CSA and SSA in the context of Next.js:
Client-Side Authentication (CSA): Checks happen in the user's browser after the page's JavaScript has loaded. The initial HTML/JS might be served regardless of auth status, and client-side logic then decides whether to show content or redirect.
Server-Side Authentication (SSA): Checks happen on the server (or at the Edge) before the final response is sent to the browser. Unauthenticated users can be redirected or denied access before they receive the protected page's code or API data. Next.js Middleware is the prime example of this.
Here’s a high-level comparison:
Feature | Client-Side Auth (CSA) in Next.js | Server-Side Auth (SSA) in Next.js (e.g., Middleware) |
Where Check Occurs | Browser (after JS load) | Server / Edge (before response) |
Security Level | Low (UI obfuscation) | High (Prevents access to code/data) |
API Protection | Very Difficult / Insecure | Yes (Natural fit for Middleware) |
FOUC Risk | High (needs careful handling) | Low / None (Redirects happen server-side) |
Performance | Initial page load unaffected, but requires client-side JS execution & potential subsequent fetches/redirects. | Adds latency server-side/edge, but prevents sending unnecessary bundles to unauth users. Can be very fast on Edge. |
Initial Setup | Can feel simpler initially for pure React devs. | Requires understanding Middleware/server concepts. |
Typical Use Case | Public pages with optional logged-in features, simple UI gating after basic access is granted. | Protecting sensitive pages/sections, securing API routes, enforcing strict access control. |
The key takeaway: For anything requiring genuine security (user dashboards, sensitive data, protected APIs), SSA via Next.js Middleware is the necessary approach. CSA alone is insufficient for protecting resources.
Diving Deeper: Client-Side Authentication Design Patterns
When you implement logic that reacts to authentication state on the client, you typically use these patterns:
The
useAuth
Custom Hook (Client-Side State Management):Role in CSA: This pattern is the heart of managing auth state within the browser. It usually consumes a React Context and provides components with information like
user
,isAuthenticated
,isLoading
, and functions likelogin
/logout
. It often fetches session data client-side after the initial page load.Example:
// hooks/useAuth.js - Provides access to auth state client-side import { useContext } from 'react'; import { AuthContext } from '../context/AuthContext'; export const useAuth = () => { const context = useContext(AuthContext); if (!context) { throw new Error('useAuth must be used within an AuthProvider'); } return context; // { user, isLoading, login, logout } };
Pros: Centralizes client-side state, reusable, drives UI updates.
Cons: Doesn't provide security itself, relies on data being fetched/available client-side.
The
ProtectedRoute
Component (Client-Side UI Guarding):Role in CSA: This component uses the state from
useAuth
to conditionally render UI. It wraps pages or components and decides, based on the client-side auth state, whether to show its children, display a loader, or trigger a client-side redirect.Example:
// components/ClientProtectedRoute.js - Reacts to useAuth state import { useEffect } from 'react'; import { useRouter } from 'next/router'; import { useAuth } from '../hooks/useAuth'; import LoadingSpinner from './LoadingSpinner'; const ClientProtectedRoute = ({ children }) => { const { user, isLoading } = useAuth(); const router = useRouter(); useEffect(() => { if (!isLoading && !user) { router.push('/login'); // Client-side redirect } }, [user, isLoading, router]); if (isLoading || !user) { // Show loader while checking or if redirecting return <LoadingSpinner />; } return <>{children}</>; // Render protected content };
Pros: Granular UI control, can show loading states nicely.
Cons: Not secure! Bundle is still sent. Prone to FOUC. Adds client-side overhead.
Summary of CSA Patterns: These patterns are essential for building the user interface related to authentication (displaying user info, login/logout buttons, showing loading states). However, they must not be relied upon as the primary security mechanism for protecting routes or data.
Diving Deeper: Server-Side Authentication Design Patterns
To achieve robust security in Next.js, you need to leverage its server-side capabilities.
Next.js Middleware (The Modern Standard):
Role in SSA: This is the primary pattern for implementing SSA in modern Next.js. It intercepts requests on the server/edge before they reach your page or API route handlers. It can validate session tokens (e.g., from secure cookies), and redirect unauthenticated users immediately.
Example:
// middleware.js - Enforces access server-side import { NextResponse } from 'next/server'; import { getToken } from 'next-auth/jwt'; // Example helper export async function middleware(req) { const { pathname } = req.nextUrl; const protectedPaths = ['/dashboard', '/api/protected']; if (protectedPaths.some((p) => pathname.startsWith(p))) { const token = await getToken({ req, secret: process.env.SECRET }); if (!token) { const url = req.nextUrl.clone(); url.pathname = '/login'; return NextResponse.redirect(url); // Server-side redirect } } return NextResponse.next(); // Allow access } export const config = { matcher: ['/dashboard/:path*', '/api/protected/:path*'], };
Pros: High Security, protects pages and APIs, prevents FOUC, centralized logic, performant (especially on Edge).
Cons: Learning curve, runtime limitations (esp. Edge), client still needs state for UI (
useAuth
).
(Legacy Mention)
getServerSideProps
Checks:Role in SSA (Older): Before Middleware became stable, developers often put authentication checks inside
getServerSideProps
. If the check failed, they could return a redirect object.Why Middleware is Preferred: Middleware runs earlier, covers more cases (API routes, static pages with revalidation if needed), can run on the Edge, and keeps page-specific data fetching logic cleaner.
getServerSideProps
checks only protect pages using that specific data-fetching method.
Summary of SSA Patterns: Middleware is the go-to pattern for enforcing authentication server-side in Next.js. It's the foundation of a secure application.
The Synergy: Combining Server-Side Security with Client-Side UX
So, which approach or pattern is "best"? As often happens in engineering, the answer is "it depends," but the most robust solution involves using them together strategically:
Use Server-Side Auth (Middleware) for Security: Implement Middleware to protect all sensitive pages and API routes. This is your non-negotiable security layer. It validates sessions server-side and redirects unauthorized users before they access protected resources.
Use Client-Side Auth Patterns (
useAuth
) for UI State: Even after Middleware grants access, your client-side components need to know the user's status to render the correct UI. Use auseAuth
hook (fetching session data client-side via a protected API route) to manage this state.Use Client-Side Patterns (
ProtectedRoute
) for UX Polish (Optional): A client-sideProtectedRoute
can still be useful, not for security, but for handling the brief period whileuseAuth
is fetching the session on the client after Middleware has already granted access. It can display a loading spinner for a smoother transition.
Revised Request Flow Example:
User requests
/dashboard
.SSA (Middleware): Checks session cookie server-side. Fail? Redirects to
/login
. Success? Allows request./dashboard
page is served.CSA (
useAuth
): Hook initializes client-side,isLoading
is true.(Optional CSA
ProtectedRoute
): Shows a loading spinner based onuseAuth().isLoading
.CSA (
useAuth
): Fetches user details from/api/auth/session
(itself protected by Middleware).CSA (
useAuth
): Updates state withuser
data,isLoading
becomes false.UI renders user-specific content based on
useAuth
state.
Conclusion: Layer Your Defenses
Understanding the distinction between Client-Side and Server-Side Authentication in Next.js is crucial.
Rely on Server-Side Authentication (Middleware) as your primary security mechanism. It's the only way to truly protect pages and API routes before sensitive information is exposed.
Leverage Client-Side Authentication patterns (
useAuth
, minimalProtectedRoute
) for managing UI state and providing a smooth user experience after server-side access control has done its job.
Don't fall into the trap of thinking client-side checks alone provide security. By layering server-side enforcement with client-side state management and UX patterns, you build Next.js applications that are both robustly secure and delightfully interactive. Tools like NextAuth.js or Clerk often provide excellent abstractions that help implement this layered strategy effectively.
Subscribe to my newsletter
Read articles from Opeyemi Ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
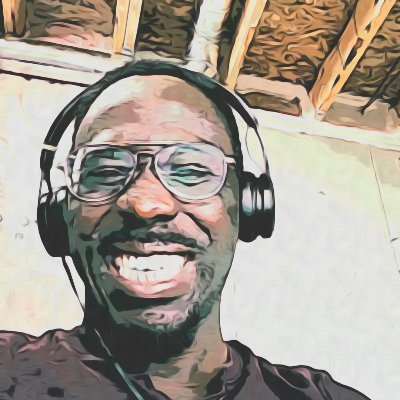