Automating the Unautomatable: How I Built a Python Bot to Snag Coveted Restaurant Reservations
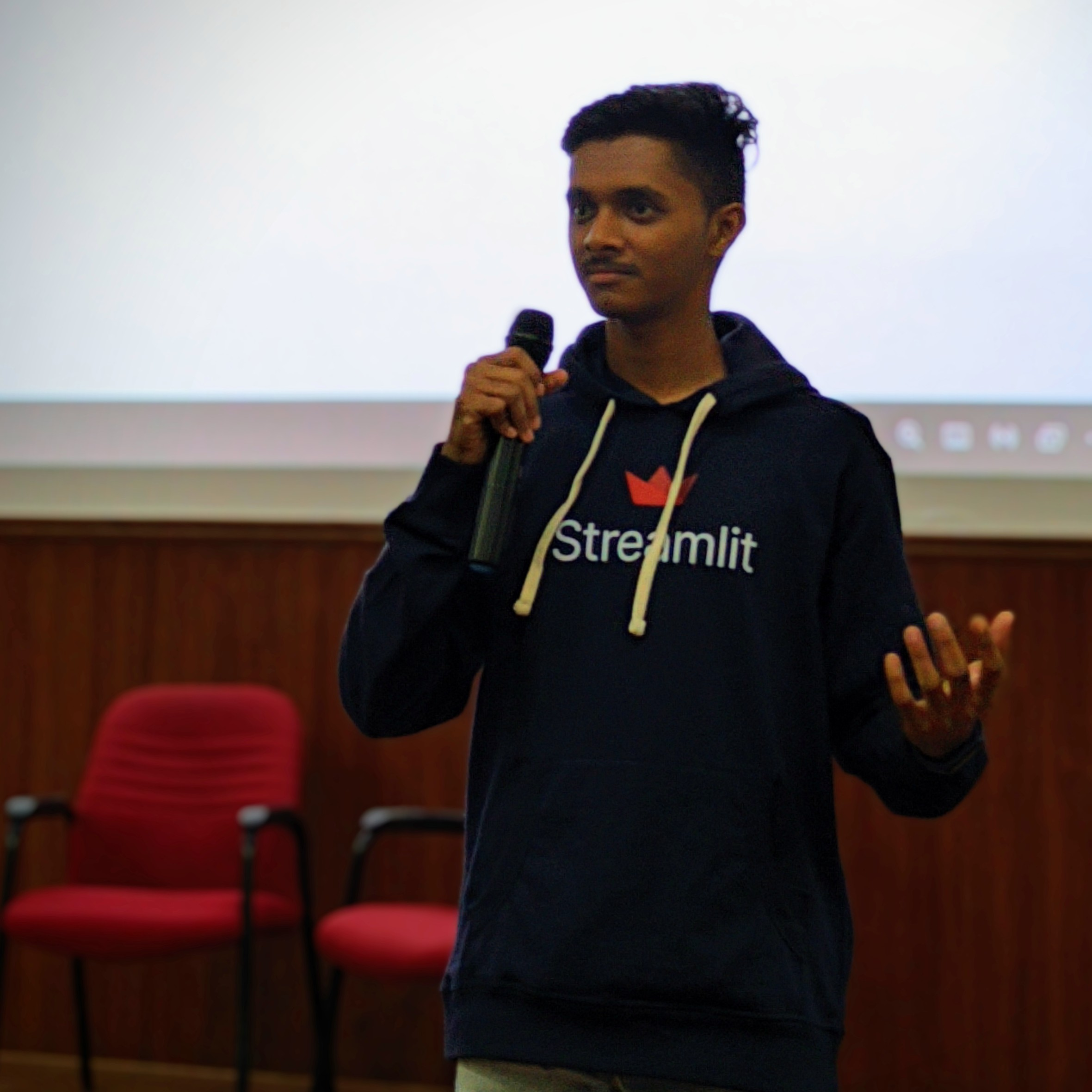

Hello!!
Another day and another automation script!!
This time its about automating the table reservation @ Naru Noodle - Bangalore : THE MOST FAMOUS RAMEN PLACE OF ALL TIME.
Ever felt that rush of anxiety when reservations for your favourite, perpetually booked-out restaurant go live? You're poised, fingers hovering, ready to click, only to find everything gone in a flash. It's a familiar frustration for many foodies, and it's exactly what inspired my latest project.
The Challenge: Snagging a Table at High-Demand Restaurants
Let's take a real-world example that many in my circle can relate to: Naru Noodle Bar. If you've ever tried to book a table there (their booking site is https://bookings.airmenus.in/eatnaru/order
), you'll know it's an incredibly tough ticket! Reservations famously open every Monday at 8 PM and, due to its immense popularity and very limited seating (just 8 counter seats and 3 tables!), slots are often snapped up literally within seconds. Blink, and you've missed your chance for the week. This intense competition was a big inspiration for building an automation tool – to level the playing field and give users a fair shot at securing a coveted spot without the stress of a manual click-race.
Introducing "Clicks Before Chopsticks": My Automation Solution
To tackle this, I developed "Clicks Before Chopsticks," a Python-based automation script that uses Selenium WebDriver to navigate the booking process for you. It’s designed to handle the entire flow, from selecting a date and time to filling out your details, stopping just short of the final confirmation so you can review everything.
How It Works: Step-by-Step
The automation performs a sequence of actions to secure a booking:
Browser Initialization: It starts by launching a Chrome browser instance using Selenium WebDriver, configured to maximize the window and navigate to the target booking website.
Date Selection: The script patiently waits for the restaurant's booking calendar to load. Once available, it identifies and clicks on your specified target date, even handling scenarios where dates might not be immediately visible.
Venue/Seating Selection: It then locates the specific venue or seating section (e.g., "RAMEN BAR SEATING" or a "DINNER" option) and clicks the corresponding "BOOK" button, validating that the selection was successful.
Time Slot Selection: After venue selection, it waits for the time slots to appear, identifies all available options, and selects your chosen time (e.g., "07:00 PM").
Guest Count Configuration: The script locates the guest counter and adjusts the number of guests (incrementing or decrementing) to your desired party size.
Form Filling: Finally, it inputs the necessary customer details: Name, Email, and Mobile Number. It also automatically accepts the terms and conditions, preparing the booking for your final manual review and confirmation.
Key Features
Automated Calendar Navigation: Intelligently selects dates.
Precise Time Slot Selection: Finds and clicks your preferred time.
Dynamic Guest Count: Easily sets the number of diners.
Automatic Form Filling: Saves you typing time for personal details.
Configurable Environments: Comes with pre-set URLs for both
test
(for safe experimentation on a dummy site) andproduction
(for the real deal, like Naru).Detailed Logging: Every step, success, or error is logged to a file and the console for transparency.
Flexible CLI: Use command-line arguments to override default booking parameters like date, time, name, etc.
MIT Licensed: Open source and free to use, modify, and share!
Under the Hood: Tech Stack & Setup
The project relies on a few key technologies:
Python (3.8+): The scripting language at its core.
Selenium WebDriver: For browser automation and web interaction.
webdriver-manager: Automatically manages the ChromeDriver, so you don't have to worry about version mismatches.
Configuration is Key
All core settings are neatly tucked away in config/
config.py
. Here you can tweak:
URLs for different booking sites: Python
Default booking parameters like target date, time, and guest count: Python
BOOKING_CONFIG = { "target_date": "19 May 2025", # Change as needed! "target_time": "07:00 PM", "guest_count": 4, # ... and venue labels for different environments }
Browser settings and even CSS/XPath selectors if the target site's structure changes.
Running the Automation
You can run the script directly from your terminal:
Basic Usage (uses default settings from
config.py
, typically targeting the test environment): BashAdvanced Usage (override defaults with command-line arguments): Bash
./run.py \ --environment test \ --date "20 May 2025" \ --time "08:30 PM" \ --guests 2 \ --name "Jane Doe" \ --email "jane@example.com" \ --mobile "0987654321"
Supported Command-Line Arguments:
--environment
:test
orproduction
--date
: "DD Month YYYY" (e.g., "19 May 2025")--time
: "HH:MM AM/PM" (e.g., "07:00 PM")--guests
: Number of guests--name
: Your name--email
: Your email--mobile
: Your mobile number
A Glimpse of the Action (Log Output)
Here's what a successful run looks like in the logs (this example is from the test environment):
2025-05-13 01:51:46,105 - INFO - Browser setup successful
2025-05-13 01:51:48,456 - INFO - Navigated to https://bookings.airmenus.in/CafeAir/CafeAir
2025-05-13 01:51:48,457 - INFO - Waiting for booking calendar to appear...
2025-05-13 01:51:48,469 - INFO - Calendar found!
2025-0_5-13 01:51:48,469 - INFO - Looking for date: 13 May 2025
2025-05-13 01:51:48,739 - INFO - Date found: 13 May 2025
2025-05-13 01:51:48,748 - INFO - Searching for booking option: 'DINNER (Counter Seats)'
2025-05-13 01:51:48,771 - INFO - BOOK button clicked successfully
2025-05-13 01:51:50,771 - INFO - Attempting to select time slot: 08:30 PM
2025-05-13 01:51:52,828 - INFO - Found 2 time slot options
2025-05-13 01:51:52,862 - INFO - Found matching time slot: 08:30 PM
2025-05-13 01:51:52,870 - INFO - Setting guest count to 4
# ... (guest count updates) ...
2025-05-13 01:51:55,500 - INFO - Updated guest count: 4
2025-05-13 01:51:55,500 - INFO - Attempting to click continue button
2025-05-13 01:51:55,519 - INFO - Filling booking form
2025-05-13 01:51:55,850 - INFO - Form filled successfully
2025-05-13 01:51:55,850 - INFO - Booking process completed successfully!
2025-05-13 01:51:55,850 - INFO - Please review the details and confirm the booking manually.
Project Structure Overview
The codebase is organized for clarity and maintainability:
clicks-before-chopsticks/
├── config/
│ └── config.py # All your configurations
├── src/
│ └── booking_automation/
│ ├── __init__.py
│ ├── main.py # CLI and main execution flow
│ ├── browser.py # Selenium browser management
│ └── booking_operations.py # Logic for booking steps
├── logs/ # Log files are stored here
├── requirements.txt # Project dependencies
├── run.py # Executable script
├── LICENSE
└── README.md
Error Handling & Logging
Comprehensive error handling is built-in to manage issues like elements not found, click failures, or network problems. Each error is logged with details to help with debugging. The script will inform you about the progress and any hitches along the way.
Open Source & Your Contributions
This project is licensed under the MIT License, so you're welcome to fork it, adapt it for other booking sites, or enhance its features. Contributions, suggestions, and feedback are highly encouraged!
Check out the full code and README on GitHub:
https://github.com/myselfshravan/**
https://drive.google.com/file/d/1yEdbqhmbqIkCMkb0k45RvwgXMchGvnqz/view
"Clicks Before Chopsticks" started as a personal project to solve a common annoyance, but I hope it can be useful to others too. Web automation is a powerful tool, and this is just one practical application.
Thanks for reading!
Subscribe to my newsletter
Read articles from Shravan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
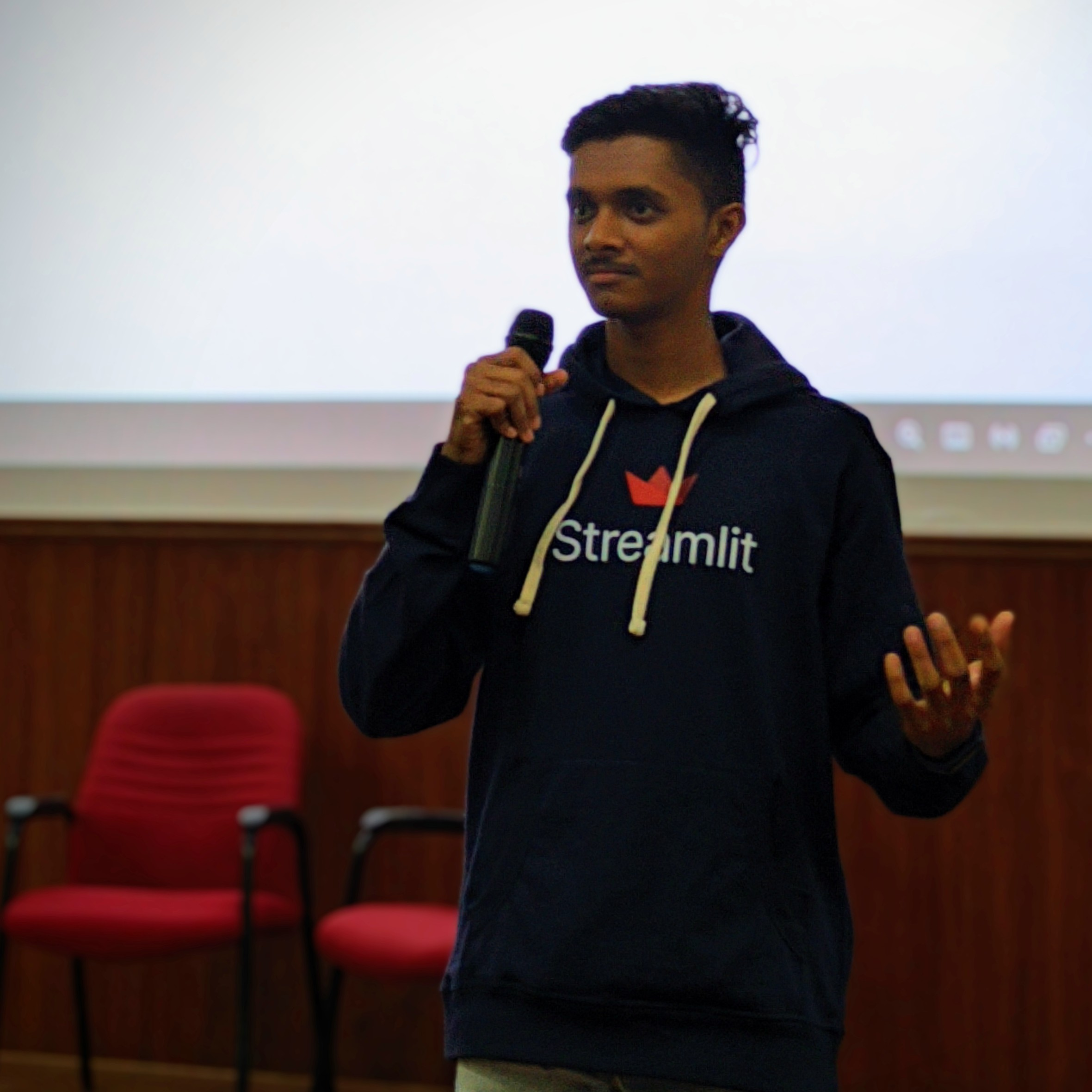
Shravan
Shravan
As a junior student at M S Ramaiah Institute of Technology, I am pursuing a degree in CSE (Spl. AI & ML). My passion for coding started a few years ago, and I have been constantly striving to expand my knowledge and skills, working with a variety of technologies. Although you can find a few of my projects online, some are private too 😉. My goal is to make a meaningful impact by contributing to open-source projects and creating practical solutions that can save time for others. In addition to my technical pursuits, I share my thoughts and knowledge on technology through my blog and enjoy helping others with common tech challenges.