Domain-Driven Design: Bridging Complexity with Clarity in Modern Software Systems
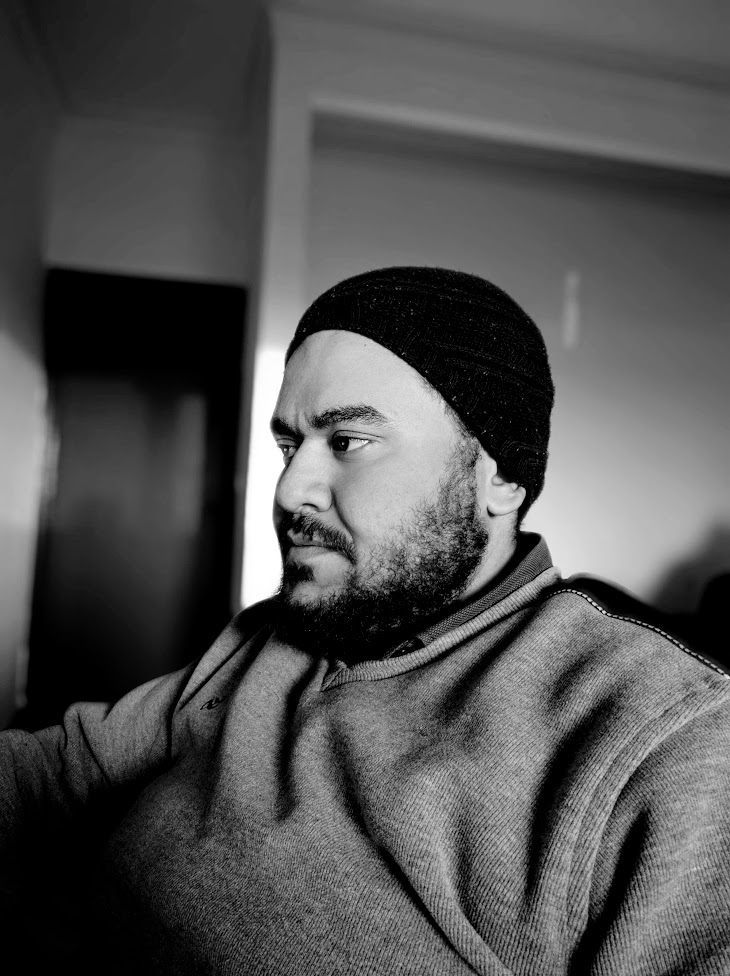
In an age where software complexity is scaling faster than ever, driven by distributed systems, evolving business models, and demanding delivery cycles, there’s a growing need for strategies that go beyond writing code. Domain-Driven Design (DDD) is not a pattern or a framework. It’s a mindset. A discipline. A lens through which software engineers, architects, and stakeholders can view the chaos of requirements and bring order to it.
🧭 Introduction: The Crisis of Complexity
Software is rarely built in a vacuum. Every system we develop serves a purpose that lives outside the realm of code: automating financial systems, supporting logistics networks, managing healthcare workflows, or orchestrating microservices in a distributed architecture.
However, as systems grow, complexity arises—not always from the technology stack, but often from a misalignment between the software and the business domain it aims to serve. This misalignment leads to:
• Fragile codebases
• Miscommunication between developers and domain experts
• Slow adaptation to changing requirements
• Architectural erosion over time
Enter Domain-Driven Design (DDD)—an approach pioneered by Eric Evans in his seminal book “Domain-Driven Design: Tackling Complexity in the Heart of Software.”
📚 1. The Philosophical Foundation of DDD
At its core, DDD is based on a simple premise:
The design of a software system should be guided by the domain it models.
This is not a trivial statement. Let’s unpack the components:
➤ What is the Domain?
The domain is the sphere of knowledge and activity around which the application logic revolves. For a banking system, the domain involves accounts, transactions, interest calculations, and regulations.
➤ Who Are Domain Experts?
Domain experts are not developers. They are the stakeholders who live and breathe the problem space: accountants, logistics managers, doctors, or loan officers. In DDD, they become first-class collaborators.
➤ What Does “Driven” Mean?
It means the domain drives the design—not the database, not the framework, and not the technology trends. The idea is to shape the codebase to reflect the real-world model in a precise, expressive, and evolving way.
🏛️ 2. The Building Blocks of DDD
Let’s explore the key building blocks that form the foundation of DDD.
2.1 Ubiquitous Language
A ubiquitous language is a common, rigorous vocabulary shared by developers and domain experts.
• It is embedded in code: class names, method names, and modules should mirror real-world terminology.
• It eliminates translation layers between business and development.
🧩 Example: In an insurance domain, “PolicyHolder” and “Premium” are better terms than “User” or “Cost.”
2.2 Bounded Context
One of DDD’s most powerful concepts. A bounded context defines a clear boundary within which a particular model is defined and applicable.
• Inside the context, terms have a consistent meaning.
• Outside the context, the same term might mean something different.
📌 In e-commerce:
• In the Sales context, a “Customer” is a buyer.
• In the Support context, a “Customer” is a help-desk ticket submitter.
2.3 Aggregates
An aggregate is a cluster of domain objects treated as a single unit for data changes.
• One entity is the aggregate root (e.g., Order), and the other entities are internal (OrderLine).
• External systems only interact with the root.
Rules:
• All updates must go through the aggregate root.
• Aggregates are transaction boundaries.
2.4 Entities and Value Objects
• Entities have identity (Customer, Account).
• Value Objects are immutable and defined by their values, not identity (Money, Address, DateRange).
2.5 Domain Services
When behavior doesn’t fit naturally within a single entity or value object, it becomes a domain service.
• Stateless
• Operates over multiple entities or aggregates
🔧 Example: TransferService.transfer(from, to, amount)
🧪 3. Tactical Design: Applying DDD in Real Code
Layered Architecture
DDD traditionally uses a layered architecture:
1. Domain Layer – Pure business logic, aggregates, entities, services
2. Application Layer – Use cases, workflows, orchestration
3. Infrastructure Layer – Repositories, messaging, databases
4. Interface Layer – Controllers, APIs, UI
✅ Pro Tip: Keep the domain layer free from infrastructure concerns.
Repository Pattern
Used to abstract data access. A repository provides a collection-like interface to aggregates.
type OrderRepository interface {
Save(order Order)
FindById(orderID string) (Order, error)
}
🛠️ 4. Strategic Design: Aligning Teams and Models
DDD shines in large-scale systems and microservices, where not everything can or should use a single model.
Context Mapping
When multiple bounded contexts interact, we need to define context maps to govern their relationships.
• Shared Kernel – Shared code between two teams
• Customer-Supplier – One context depends on another
• Conformist – A context conforms to another’s model
• Anticorruption Layer (ACL) – Protects the domain from influence of external models
💡 An ACL acts as a translator to keep your domain clean and independent.
💼 5. Real-World Application Scenarios
E-Commerce Platform
• Bounded Contexts: Checkout, Inventory, Pricing, Payment, Order Fulfillment
• Aggregate Example: Order → Contains OrderLines, tracks status
• Domain Events: OrderConfirmed, PaymentFailed, InventoryReserved
Banking System
• Bounded Contexts: Account Management, Fraud Detection, Loan Processing
• Aggregates: Account, Transaction
• Value Objects: Money, InterestRate
🧬 6. Common Pitfalls in DDD Adoption
Despite its strengths, DDD is not a silver bullet. Here are some traps to avoid:
• Over-modeling: Not every system needs full DDD treatment.
• Ignoring Ubiquitous Language: Teams still speak in “tech talk.”
• Lack of Domain Expert Involvement: DDD without business input is just technical modeling.
• Accidental Complexity: DDD should tame complexity, not create new abstractions for the sake of it.
🧭 7. DDD in the Age of Microservices and Event-Driven Systems
In distributed systems, DDD becomes indispensable:
• Each microservice = One bounded context
• Domain events become the medium of communication
• Aggregates enforce consistency within a service
• Eventual consistency is modeled explicitly across services
🌐 Example: When OrderPlaced is emitted, downstream services like Inventory and Payment can react asynchronously.
📘 8. DDD and Modern Tools & Practices
• CQRS (Command Query Responsibility Segregation)
• Commands modify state (write model)
• Queries read state (read model)
• Event Sourcing
• State is derived from a sequence of events
• Hexagonal Architecture
• Core logic at the center, ports/adapters at the edges
🎯 9. When to Use DDD (and When Not To)
✅ Use DDD when:
• The domain is complex and rapidly evolving
• Business rules are central to success
• You have access to domain experts
• Multiple teams are working on different parts of the system
❌ Avoid DDD when:
• The domain is CRUD-heavy and simple
• No access to domain experts
• Small monolithic apps with little business logic
🧠 10. Final Thoughts: DDD as an Ongoing Conversation
Domain-Driven Design is not a checklist. It’s a continuous journey of refining models, improving communication, and aligning code with real-world concepts. It encourages collaboration, not just among developers, but across the business.
In the end, DDD isn’t about writing better code—it’s about building better understanding.
“Software that matters, starts with a model that matters.”
— Eric Evans
🏷️ Suggested Tags for Blog
Would you like this converted to a downloadable PDF or published to a documentation platform?
Subscribe to my newsletter
Read articles from Yousif Aziz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
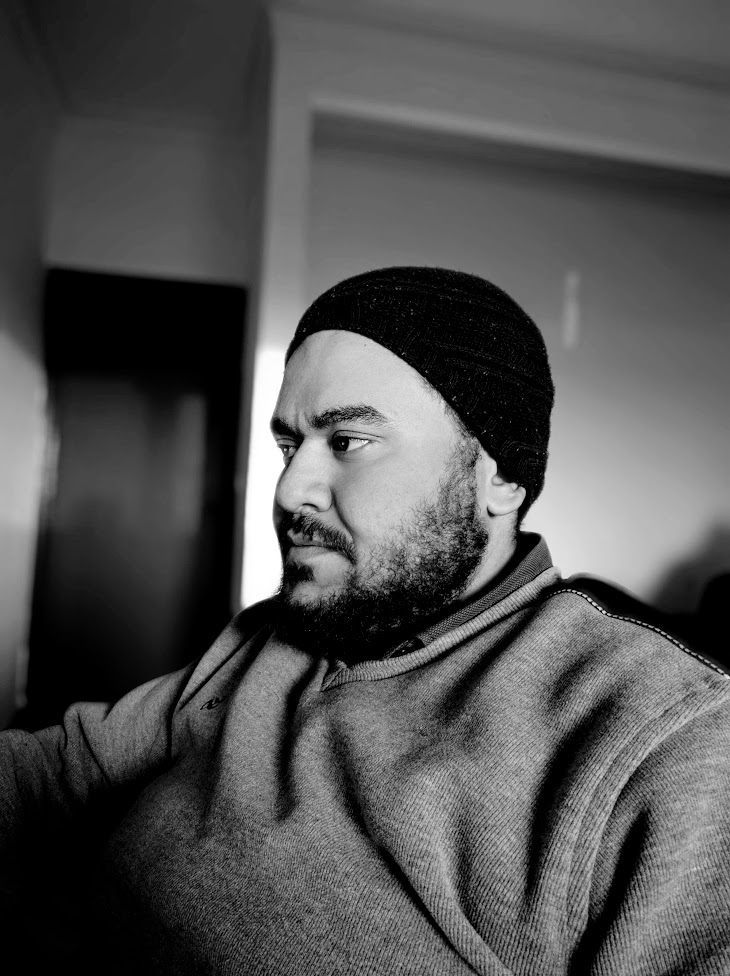
Yousif Aziz
Yousif Aziz
Mainly I've been working as a web developer for ten years, my experience in the back-end, front-end, and leadership. I also have a great experience with DevOps and Cloud Computing (AWS and Linux servers).