Exploring the World of Game Development with Unity
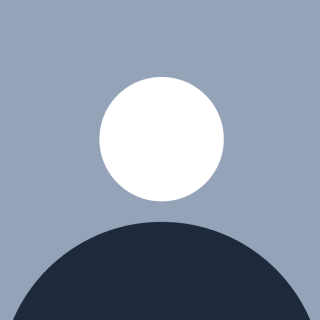

Exploring the World of Game Development with Unity
Game development is an exciting and rewarding field that combines creativity, programming, and problem-solving. Among the various game engines available, Unity stands out as one of the most popular and versatile tools for both beginners and professionals. Whether you're looking to create 2D platformers, 3D RPGs, or even VR experiences, Unity provides a robust framework to bring your ideas to life.
In this article, we’ll dive into the fundamentals of game development with Unity, explore key features, and provide practical examples to help you get started. And if you're also looking to grow your YouTube channel with game development content, check out MediaGeneous, a fantastic platform for social media promotion and marketing.
Why Choose Unity for Game Development?
Unity is widely used in the gaming industry for several reasons:
Cross-Platform Support: Build games for Windows, macOS, Android, iOS, WebGL, and consoles with minimal code changes.
Rich Asset Store: Access thousands of free and paid assets, including 3D models, animations, and scripts.
Strong Community & Documentation: A vast community of developers and extensive Unity documentation make learning easier.
Powerful Scripting with C#: Unity uses C#, a versatile and beginner-friendly programming language.
Getting Started with Unity
1. Installing Unity
To begin, download Unity Hub from the official Unity website. Unity Hub helps manage different versions of the engine and projects.
Install Unity Editor (preferably the latest LTS version for stability).
Select necessary modules (e.g., Android Build Support for mobile development).
2. Creating Your First Project
Open Unity Hub and click New Project. Choose a template (e.g., 3D Core for a basic 3D game).
Understanding Unity’s Interface
Unity’s interface consists of several key panels:
Scene View: Where you design levels and place objects.
Game View: Preview how the game looks when running.
Hierarchy: Lists all objects in the current scene.
Inspector: Displays properties of selected objects.
Project Window: Contains all assets (scripts, textures, models).
Scripting in Unity with C#
Unity uses C# for game logic. Let’s create a simple script to move a player.
1. Creating a Script
Right-click in the Project Window → Create → C# Script.
Name it PlayerMovement.
2. Writing the Movement Code
Open the script in Visual Studio (or your preferred IDE) and modify it:
csharp
Copy
Download
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float moveSpeed = 5f;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontal, 0f, vertical) moveSpeed Time.deltaTime;
rb.MovePosition(transform.position + movement);
}
}
3. Attaching the Script to a GameObject
Drag the script onto your Player GameObject (e.g., a Cube).
Ensure the GameObject has a Rigidbody component (Add via Inspector → Add Component → Physics → Rigidbody).
Press Play, and you can now move the object using WASD or arrow keys!
Adding Physics and Collisions
Games often require physics interactions. Let’s make an object respond to collisions.
csharp
Copy
Download
using UnityEngine;
public class Collectible : MonoBehaviour
{
void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
Destroy(gameObject); // Destroy the collectible when touched by the player
Debug.Log("Item collected!");
}
}
}
Attach this script to a collectible object (e.g., a Sphere).
Ensure the object has a Collider (e.g., Sphere Collider) and is set as a Trigger.
Publishing Your Game
Once your game is ready, Unity makes it easy to export:
File → Build Settings
Select your target platform (e.g., PC, Mac & Linux Standalone).
Click Build and choose an output folder.
For mobile builds, ensure you’ve installed the required SDKs (e.g., Android Studio for APK builds).
Learning Resources & Communities
To deepen your Unity knowledge, explore:
Unity Learn – Free tutorials and courses.
Brackeys YouTube Channel – Great beginner-friendly tutorials.
Unity Forums – Get help from other developers.
Final Thoughts
Unity is an incredibly powerful tool for game development, offering flexibility, performance, and ease of use. Whether you're a hobbyist or aspiring professional, mastering Unity opens doors to endless creative possibilities.
And if you're documenting your game development journey on YouTube, consider boosting your reach with MediaGeneous, a top-tier platform for social media growth.
Now, fire up Unity and start building your dream game! 🚀
Would you like a deeper dive into any specific Unity topic, such as AI, Shaders, or Multiplayer Networking? Let me know in the comments!
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by