Secrets to Scaling a Monolithic Application Effectively
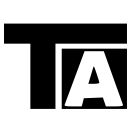
4 min read
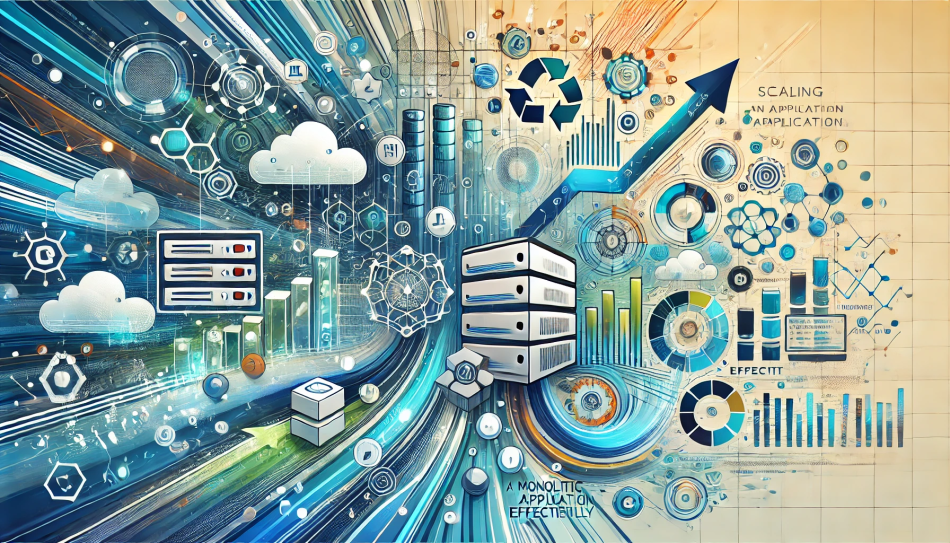
1. Understanding Monolithic Architecture Scaling
Scaling monolithic applications requires us to understand how they are structured and the challenges associated with them.
The Basics of Monolithic Architecture
A monolithic application is a single unified codebase, often built on a three-tier architecture: presentation, business logic, and data layers. Scaling involves increasing the capacity to handle more transactions and users, but it has its limitations compared to microservices.
Why Monolithic Applications Need Scaling
With user growth, system demands increase, making it essential to enhance processing capabilities. In this section, discuss typical issues, like request bottlenecks and database overload, that a monolith faces without proper scaling.
Challenges in Scaling Monoliths
Unlike microservices, a monolithic system cannot scale individual components independently. This section should explain why monoliths require more complex scaling techniques and the potential for downtime or inefficiencies.
2. Techniques for Scaling Monolithic Applications
2.1 Vertical Scaling
Vertical scaling is increasing resources such as CPU and RAM to improve performance. Here’s a basic code snippet showing how an application can be configured to use maximum available CPU resources:
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class AppScaling {
public static void main(String[] args) {
int cores = Runtime.getRuntime().availableProcessors();
ExecutorService executorService = Executors.newFixedThreadPool(cores);
// Application tasks can now utilize the maximum CPU
}
}
Explanation: This code dynamically sets the application to utilize all available CPU cores, a basic approach to vertical scaling within the application itself. However, vertical scaling often has limitations due to hardware constraints.
2.2 Horizontal Scaling
Horizontal scaling involves adding more instances of the application to distribute the load. While monolithic applications are not inherently designed for this, certain techniques, like using a load balancer, can facilitate horizontal scaling.
Example: Setting up a Load Balancer for Horizontal Scaling
In this section, discuss how to configure a load balancer with an example of using Nginx as a load balancer for multiple instances of a monolithic Java application. Include configuration code for clarity.
http {
upstream app_servers {
server app_server_1;
server app_server_2;
server app_server_3;
}
server {
location / {
proxy_pass http://app_servers;
}
}
}
Explanation: This Nginx configuration will distribute incoming traffic to multiple instances of the application, helping to balance the load across servers.
2.3 Database Scaling Techniques
Databases are often the bottleneck in a monolithic application. Cover the methods for database scaling: sharding, replication, and partitioning. An example could illustrate how to implement database replication in MySQL for redundancy and performance:
-- Master Configuration
CHANGE MASTER TO
MASTER_HOST='slave_server_ip',
MASTER_USER='replication_user',
MASTER_PASSWORD='password',
MASTER_LOG_FILE='mysql-bin.000001',
MASTER_LOG_POS=107;
START SLAVE;
Explanation: This SQL command initiates replication, helping to reduce the load on the primary database server by allowing read operations from the replicated database.
3. Best Practices in Monolithic Application Scaling
3.1 Caching for Faster Access
Caching can significantly reduce database load and increase response time. A popular caching solution for Java applications is using Redis.
import redis.clients.jedis.Jedis;
public class CacheExample {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
jedis.set("user:1000", "John Doe");
System.out.println(jedis.get("user:1000"));
}
}
Explanation: In this example, Redis caches user information, allowing quick access without repeatedly querying the database.
3.2 Implementing Asynchronous Processing
Asynchronous processing can help distribute workload more evenly, making the application more responsive to high traffic.
Example: Using Java's CompletableFuture for async processing:
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
System.out.println("Async task");
});
future.join();
}
}
Explanation: This snippet uses CompletableFuture to execute tasks asynchronously, which is useful for handling non-blocking operations within a monolithic application.
4. Conclusion
Scaling a monolithic application can seem like a daunting task, but with the right strategies, it is achievable. Whether it’s through vertical scaling, horizontal scaling, or optimization techniques such as caching and async processing, you can improve your monolithic application’s performance and scalability. If you have further questions or would like to share your experiences, feel free to leave a comment below.
Read more at : Secrets to Scaling a Monolithic Application Effectively
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
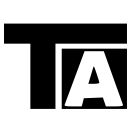
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.