Build a Text-to-Speech (TTS) Converter in Python
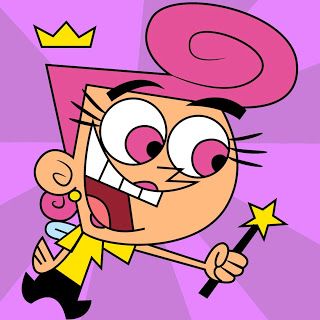

Introduction
Text-to-Speech (TTS) is the technology that converts written text into spoken words. With Python, you can build a simple TTS converter using just a few lines of code — useful for accessibility tools, audiobooks, personal assistants, or even educational apps.
In this article, you'll learn:
How TTS works
How to install and use the
pyttsx3
libraryHow to convert any string or text file into audio
How to customize the voice and speed
How to save the output as an audio file
Step 1: Install the Required Library
We'll use pyttsx3
, a Python library for offline, cross-platform text-to-speech.
Open your terminal or command prompt and run:
pip install pyttsx3
Unlike some TTS tools,
pyttsx3
doesn’t need an internet connection — it uses your system’s built-in speech engine.
Step 2: Create the Python Script
Here’s the full code — copy, paste, and run it. Explanations are provided below.
import pyttsx3 # Import the text-to-speech engine
import os # To interact with the file system
# Step 1: Initialize the TTS engine
engine = pyttsx3.init()
# Step 2: Set voice properties (optional)
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[0].id) # Change to voices[1].id for female voice if available
engine.setProperty('rate', 150) # Speed of speech (default is ~200)
# Step 3: Define your text (optionally read from a file)
text = "Hello! This is a simple text-to-speech converter built with Python."
# Optional: Load text from a .txt file
# with open("sample.txt", "r") as file:
# text = file.read()
# Step 4: Speak the text aloud
engine.say(text)
engine.runAndWait()
# Step 5: Save the speech to an audio file
engine.save_to_file(text, 'output_audio.mp3')
engine.runAndWait()
print("Speech playback complete and saved as 'output_audio.mp3'")
Step-by-Step Explanation
pyttsx3.init()
This initializes the text-to-speech engine based on your OS — using:
SAPI5 on Windows
NSSpeechSynthesizer on macOS
espeak on Linux
Set Voice and Rate
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[0].id)
engine.setProperty('rate', 150)
voices[0]
is usually the default male voice.You can try
voices[1]
for a female voice if available.rate
adjusts the speaking speed — lower = slower.
Load Text from File (Optional)
with open("sample.txt", "r") as file:
text = file.read()
This is useful if you want to read long passages, eBooks, or documents instead of hardcoding the string.
Speak the Text
engine.say(text)
engine.runAndWait()
This speaks the text out loud immediately using your system’s audio output.
Save to File
engine.save_to_file(text, 'output_audio.mp3')
Saves the speech as an MP3 (or WAV) file for later use — perfect for offline listening or sharing.
On some systems, the saved file format may be
.wav
. If.mp3
doesn’t work, try'output.wav'
.
Organizing Your Project
Here’s a simple project structure:
text_to_speech/
├── text_to_speech.py
├── sample.txt # Optional text input
├── output_audio.mp3 # Saved output
Optional Enhancements
Feature | Tool / Tip |
GUI Interface | Use tkinter |
Batch file conversion | Loop through .txt files in a folder |
Web interface | Use Flask or Streamlit |
Email audio output | Use smtplib |
Add voice selection | Allow user to choose from voices[] |
Use Cases
Reading books or articles aloud
Creating educational voice clips
Making accessible apps for the visually impaired
Generating voice alerts for automation systems
Final Testing Tips
Before using your script in a full project:
Check that your microphone/speakers are working
Ensure Python can access the TTS engine
Try different voice settings if speech doesn’t sound right
Summary
Feature | Included |
Offline TTS | Yes |
Custom voice/speed | Yes |
File input/output | Yes |
With this script, you've built a fully functional TTS converter that can speak and save text as audio, giving you a strong foundation for advanced voice-based apps.
Happy Scripting ! !
Subscribe to my newsletter
Read articles from Michelle Mukai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
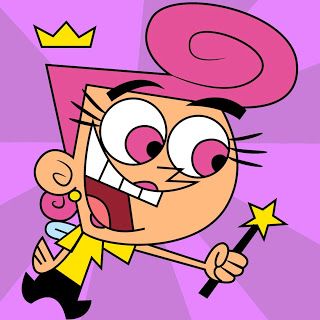