Mastering Full Stack Development: A Beginner's Roadmap to Success ( 2025 )
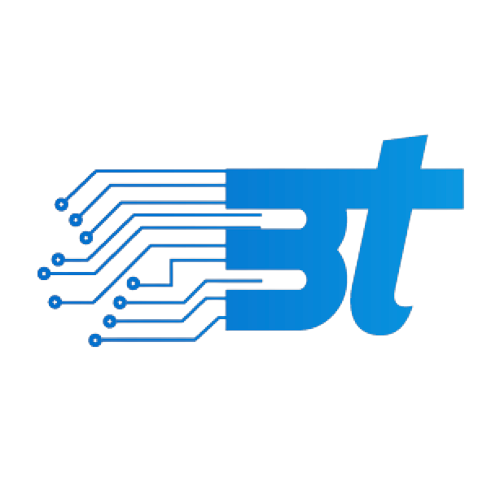
Table of contents
- Phase 1: Laying the Foundation - The Web Essentials
- Phase 2: Adding Interactivity - JavaScript Fundamentals
- Phase 3: Diving into Backend - The Server-Side Logic
- Phase 4: Connecting Frontend and Backend - Building Full Stack Applications
- Phase 5: Essential Developer Tools and Best Practices
- Continuous Learning and Next Steps (Beyond 2025)

Becoming a full-stack developer in 2025 might seem like a monumental task when you're starting from scratch. But fear not! This meticulously crafted roadmap breaks down the journey into digestible, step-by-step phases, designed for absolute beginners. We'll guide you through the essential technologies, provide learning resources, suggest practical projects, and ensure you're equipped with the knowledge to not only learn but also thrive in the world of full-stack development.
Our Goal: To empower you with a clear, actionable path to becoming a proficient full-stack developer by 2025.
Why This Roadmap is Different:
Beginner-Centric: Assumes absolutely no prior coding experience.
Step-by-Step with Visuals: Integrates image suggestions to enhance understanding.
SEO Optimized: Targets relevant keywords to help you and others find this valuable resource.
2025 Ready: Focuses on modern and in-demand technologies.
Project-Based Learning: Emphasizes practical application of knowledge.
Let's Get Started!
Phase 1: Laying the Foundation - The Web Essentials
Before diving into complex frameworks and backend logic, it's crucial to understand the fundamental building blocks of the web.
Step 1: Understanding How the Web Works
At its core, the web operates on a client-server model. Your web browser (the client) sends requests to a remote computer (the server), which then sends back the information you see (webpages, images, etc.). Understanding this basic interaction is fundamental.
Key Concepts:
Client: Your web browser (Chrome, Firefox, Safari, etc.).
Server: A computer hosting website files and applications.
HTTP/HTTPS: Protocols for communication between clients and servers.
Domain Name: The website address (e.g., https://www.google.com/search?q=google.com).
Hosting: Where website files are stored on a server.
Step 2: Mastering HTML - The Structure
(Image Suggestion: A code snippet of a basic HTML document with highlighted tags like <html>
, <head>
, <body>
, <h1>
, <p>
. Next to it, a simple rendered webpage showing the heading and paragraph.)
HTML (HyperText Markup Language) is the standard markup language for 1 creating web pages. It provides the structure and meaning to the content displayed in your browser. Think of it as the skeleton of a website.
What You'll Learn:
Basic HTML Structure:
<html>
,<head>
,<title>
,<body>
.Essential Tags: Headings (
<h1>
to<h6>
), paragraphs (<p>
), images (<img>
), links (<a>
), lists (<ul>
,<ol>
,<li>
), divs (<div>
), spans (<span>
).Semantic HTML: Using tags that convey meaning (e.g.,
<article>
,<nav>
,<aside>
,<header>
,<footer>
). This is crucial for SEO and accessibility.HTML Forms: Creating interactive forms for user input (
<form>
,<input>
,<textarea>
,<button>
).HTML Tables: Structuring tabular data (
<table>
,<tr>
,<td>
,<th>
).
Learning Resources:
MDN Web Docs (Mozilla Developer Network): Comprehensive and reliable documentation.
freeCodeCamp: Interactive HTML and CSS courses.
W3Schools: Easy-to-understand tutorials and examples.
Practice:
- Create simple static web pages: your personal profile, a recipe website, a basic product landing page.
Step 3: Styling with CSS - Making it Beautiful
CSS (Cascading Style Sheets) is used to style the visual presentation of your HTML content. It controls colors, fonts, layout, responsiveness, and more.
What You'll Learn:
CSS Selectors: Targeting specific HTML elements to apply styles (e.g., element selectors, class selectors, ID selectors).
Basic CSS Properties:
color
,font-family
,font-size
,background-color
,margin
,padding
,border
.The Box Model: Understanding how elements are structured with content, padding, border, and margin.
Layout Techniques:
Flexbox: A powerful layout module for creating one-dimensional layouts.
CSS Grid: A two-dimensional layout system for more complex page structures.
Responsive Design: Using media queries to adapt your website's layout to different screen sizes (desktops, tablets, mobiles).
Learning Resources:
MDN Web Docs (Mozilla Developer Network): CSS section.
freeCodeCamp: Responsive Web Design certification.
CSS-Tricks: Articles, tutorials, and guides on advanced CSS techniques.
Flexbox Froggy & Grid Garden: Interactive games to learn Flexbox and CSS Grid.
Practice:
- Style the HTML pages you created in Step 2. Experiment with different layouts and designs.
Phase 2: Adding Interactivity - JavaScript Fundamentals
JavaScript (JS) is a powerful programming language that adds interactivity and dynamic behavior to your web pages. It allows you to manipulate HTML and CSS, handle user events, make asynchronous requests, and much more.
What You'll Learn:
JavaScript Basics: Variables, data types, operators, control flow (if/else statements, loops), functions.
DOM (Document Object Model) Manipulation: How JavaScript interacts with the HTML structure to dynamically change content, styles, and attributes.
Events: Handling user interactions like clicks, mouse movements, and form submissions.
Asynchronous JavaScript: Understanding concepts like
setTimeout
,setInterval
, Promises, andasync/await
for handling operations that take time.Working with APIs (Application Programming Interfaces): Fetching data from external sources and integrating it into your website.
Learning Resources:
JavaScript.info: A detailed and well-structured JavaScript tutorial.
freeCodeCamp: JavaScript Algorithms and Data Structures certification.
Codecademy: Interactive JavaScript courses.
The Net Ninja (YouTube): Excellent JavaScript tutorials.
Practice:
Build interactive elements: a simple calculator, a to-do list application, a basic quiz.
Fetch data from a public API (e.g., a weather API) and display it on your page.
Phase 3: Diving into Backend - The Server-Side Logic
The backend is the engine room of your web applications. It handles data storage, user authentication, business logic, and serves the frontend.
Step 1: Choosing a Backend Language and Framework - JavaScript with Node.js and Express.js
For beginners already familiar with JavaScript, a natural progression is to use JavaScript on the backend with Node.js. Express.js is a popular and minimalist web application framework for Node.js, making it easy to build APIs and server-side applications.
What You'll Learn:
Node.js Fundamentals: Understanding the Node.js runtime environment, event loop, and non-blocking I/O.
NPM (Node Package Manager): Managing and using third-party libraries and packages.
Express.js Basics: Setting up an Express server, defining routes, handling requests and responses.
Middleware: Functions that execute during the request-response cycle.
Building RESTful APIs: Designing and implementing APIs that follow REST (Representational State Transfer) principles. Common HTTP methods (GET, POST, PUT, DELETE) and status codes.
Learning Resources:
Node.js Official Documentation: Comprehensive documentation.
Express.js Official Documentation: Clear and concise guides.
Traversy Media (YouTube): Excellent Node.js and Express.js tutorials.
The Net Ninja (YouTube): More in-depth Node.js and Express.js courses.
Practice:
Build a simple API that returns static data.
Create an API to manage a list of items (e.g., books, tasks) with CRUD operations.
Step 2: Databases - Storing and Retrieving Data
Databases are essential for storing and managing the data your application uses. For beginners, a relational database is a good starting point.
๐ ๏ธ Discover Utilshub โ Your Go-To Hub for Developer Tools, Tutorials & Automation!
Boost your productivity with practical guides, coding resources, and real-world automation tips.
๐ Visit now: https://www.utilshub.com/
Choosing a Relational Database: PostgreSQL or MySQL
PostgreSQL: A powerful, open-source relational database known for its extensibility and adherence to standards.
MySQL: Another popular open-source relational database widely used in web applications.
What You'll Learn (for your chosen database):
SQL (Structured Query Language): The standard language for interacting with relational databases. Learn
CREATE TABLE
,INSERT
,SELECT
,UPDATE
,DELETE
statements,WHERE
clauses,JOIN
operations, and basic database design principles.Database Setup and Management: Installing and configuring your chosen database.
Connecting Node.js/Express.js to the Database: Using libraries (e.g.,
pg
for PostgreSQL,mysql2
for MySQL) to interact with your database from your backend code.ORM (Object-Relational Mapper) Concepts: Understanding how ORMs (like Sequelize or Prisma for Node.js) can simplify database interactions by mapping database tables to JavaScript objects.
Learning Resources:
SQLBolt: Interactive lessons to learn SQL basics.
Codecademy: Learn SQL course.
Official Documentation for PostgreSQL/MySQL: In-depth information.
Documentation for your chosen Node.js database library (pg, mysql2) and ORM (Sequelize, Prisma).
Practice:
Design a database schema for your API project (e.g., for your list of items).
Create tables and insert sample data using SQL.
Implement API endpoints in your Node.js/Express.js application to perform CRUD operations on the database.
Phase 4: Connecting Frontend and Backend - Building Full Stack Applications
This is where the magic happens! You'll learn how to integrate your frontend and backend to create complete web applications.
Step 1: Making API Calls from the Frontend
(Image Suggestion: A code snippet showing JavaScript code using the fetch
API or Axios
to make a GET request to a backend API endpoint.)
Your frontend (HTML, CSS, and JavaScript) needs to communicate with your backend API to fetch and send data.
What You'll Learn:
fetch
API (built-in to browsers): Making HTTP requests from JavaScript.Axios
(a popular promise-based HTTP client): Often preferred for its features and ease of use.Handling API Responses: Processing data received from the backend (usually in JSON format).
Updating the UI Based on API Data: Dynamically displaying data fetched from the backend on your web pages.
Practice:
Modify your frontend projects to fetch data from the backend APIs you built in Phase 3 and display it.
Implement forms on the frontend that send data to your backend API (e.g., creating a new item).
Step 2: Frontend Frameworks/Libraries - Enhancing User Experience (Introduction to React)
While you can build complex frontends with vanilla JavaScript, using a frontend framework or library can significantly improve development efficiency and create more interactive and maintainable applications. React is a highly popular and in-demand JavaScript library for building user interfaces.
Why React for Beginners (After Mastering Core JS):
Component-Based Architecture: Breaks down the UI into reusable components.
Virtual DOM: Improves performance by efficiently updating the actual DOM.
Large and Active Community: Extensive resources and support.
What You'll Learn (Basics of React):
JSX (JavaScript XML): A syntax extension that allows you to write HTML-like structures in your JavaScript code.
Components: Functional and class components.
Props (Properties): Passing data from parent to child components.
State: Managing data within a component that can change over time.
Event Handling: Handling user interactions within React components.
Basic React Hooks:
useState
anduseEffect
for managing state and side effects in functional components.
Learning Resources:
Official React Documentation: A great starting point.
freeCodeCamp: Front End Development Libraries certification (focuses on React and Redux).
The Net Ninja (YouTube): Excellent React tutorials for beginners.
Scrimba: Interactive React courses.
Practice:
Rebuild some of your earlier frontend projects using React components.
Create simple React applications that fetch and display data from your backend API.
Phase 5: Essential Developer Tools and Best Practices
(Image Suggestion: Logos of Git, GitHub, VS Code, and a browser's developer tools window.)
To be a productive full-stack developer, you need to be familiar with essential tools and adopt good practices.
Step 1: Version Control with Git and GitHub
Git: A distributed version control system that tracks changes in your code over time.
GitHub: A web-based platform for hosting Git repositories, facilitating collaboration.
What You'll Learn:
Basic Git Commands:
git init
,git add
,git commit
,git push
,git pull
,git clone
,git status
,git log
.Branching and Merging: Managing different versions of your code.
Collaborating on GitHub: Creating repositories, making pull requests, and resolving merge conflicts.
Learning Resources:
Git Handbook: Comprehensive documentation.
GitHub Learning Lab: Interactive tutorials on Git and GitHub.
Atlassian Git Tutorials: Clear explanations and examples.
Practice:
Use Git for all your projects.
Create a GitHub account and host your code there.
Collaborate on a small project with another learner (if possible).
Step 2: Integrated Development Environment (IDE) - VS Code
screenshot of VS Code with a code file open, highlighting features like syntax highlighting and integrated terminal.
An IDE provides a comfortable and efficient environment for writing and managing your code. Visual Studio Code (VS Code) is a free, popular, and highly extensible IDE.
Key Features to Learn:
Syntax Highlighting: Makes code easier to read.
Code Completion (IntelliSense): Suggests code as you type.
Integrated Terminal: Allows you to run command-line tools directly within the IDE.
Debugging Tools: Helps you identify and fix errors in your code.
Extensions: Enhance VS Code's functionality for specific languages and frameworks.
Learning Resources:
VS Code Documentation: Official documentation.
VS Code Tips and Tricks (YouTube): Many helpful tutorials.
Practice:
Use VS Code for all your coding projects.
Explore different extensions that can improve your workflow.
Step 3: Browser Developer Tools
(Image Suggestion: A screenshot of a browser's developer tools window (e.g., Chrome DevTools), highlighting the Elements, Console, Network, and Sources tabs.)
Modern browsers come with built-in developer tools that are invaluable for debugging and inspecting your web applications.
Key Tabs to Understand:
Elements: Inspecting and modifying the HTML and CSS of a webpage.
Console: Viewing JavaScript errors, logs, and running JavaScript code.
Network: Monitoring HTTP requests and responses.
Sources: Debugging your JavaScript code.
Learning Resources:
Browser-Specific Documentation (e.g., Chrome DevTools, Firefox Developer Tools): Detailed guides.
Google Developers (YouTube): Videos on using Chrome DevTools effectively.
Practice:
- Use the developer tools to inspect websites, debug JavaScript errors, and analyze network requests for your own projects.
Step 4: Deployment - Making Your Application Live
(Image Suggestion: A simplified diagram showing code being pushed from GitHub to a hosting platform (like Netlify or Heroku), which then makes the application accessible online.)
Deployment is the process of making your web application accessible to users on the internet.
Beginner-Friendly Deployment Platforms:
Frontend (Static Sites & React Apps):
Netlify: Easy deployment with automatic builds from Git repositories.
Vercel: Another popular platform for deploying modern web applications.
GitHub Pages: Free hosting for static websites directly from your GitHub repository.
Backend (Node.js/Express APIs):
Heroku: A platform-as-a-service (PaaS) that simplifies deploying and managing web applications.
Render: Another user-friendly PaaS option.
What You'll Learn (Basic Deployment):
Setting up an account on a deployment platform.
Connecting your Git repository to the platform.
Understanding build processes (if required).
Deploying your frontend and backend applications.
Practice:
- Deploy your full-stack blog application (from Phase 3/4) to Netlify/Vercel (frontend) and Heroku/Render (backend).
Continuous Learning and Next Steps (Beyond 2025)
The tech landscape is constantly evolving. To stay relevant, embrace continuous learning:
Explore More Frontend Frameworks/Libraries: Vue.js, Angular.
Deepen Your Backend Knowledge: Learn about authentication and authorization, testing (unit, integration, end-to-end), security best practices.
Explore Databases Further: NoSQL databases (e.g., MongoDB), database scaling.
Learn About Containerization: Docker and Kubernetes.
Cloud Platforms: AWS, Google Cloud, Azure.
Stay Updated: Follow tech blogs, listen to podcasts, attend webinars and conferences.
Build More Projects: The best way to learn is by doing. Work on personal projects and contribute to open source.
Network with Other Developers: Join online communities (Stack Overflow, Reddit, Discord) and attend local meetups.
SEO Optimization Notes:
Keywords: This content strategically uses keywords like "full stack developer roadmap," "absolute beginners," "2025," "step by step," "HTML," "CSS," "JavaScript," "Node.js," "Express.js," "React," "Git," "GitHub," "deployment," and more.
Headings and Subheadings: The content is well-structured with clear headings (H1, H2, H3) to improve readability and SEO.
Internal Linking: (While not explicitly done here as this is a standalone piece) In a blog format, you would link to other relevant articles on your site.
Image Alt Text: Remember to add descriptive alt text to all images you use.
Comprehensive Content: The in-depth nature of this guide aims to provide significant value to readers, which Google favors.
Conclusion:
The journey to becoming a full-stack developer as an absolute beginner in 2025 is challenging but achievable. By following this step-by-step roadmap, focusing on consistent learning and practice, and leveraging the vast resources available, you can build a strong foundation and embark on a successful career in web development. Stay curious, keep building, and never stop learning! Good luck!
๐ How to Use Python to Automate Daily Tasks โ A Step-by-Step Tutorial
Master automation basics with Python in this beginner-friendly guide. Start saving time today!
#Python #Automation #Utilshub #Beginners
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
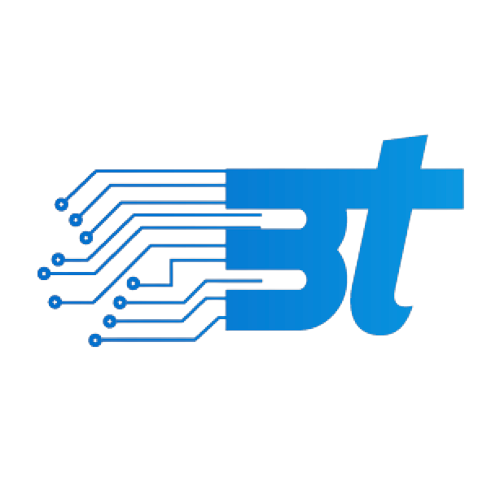
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.