Design Patterns Made Simple: Beginner's Guide
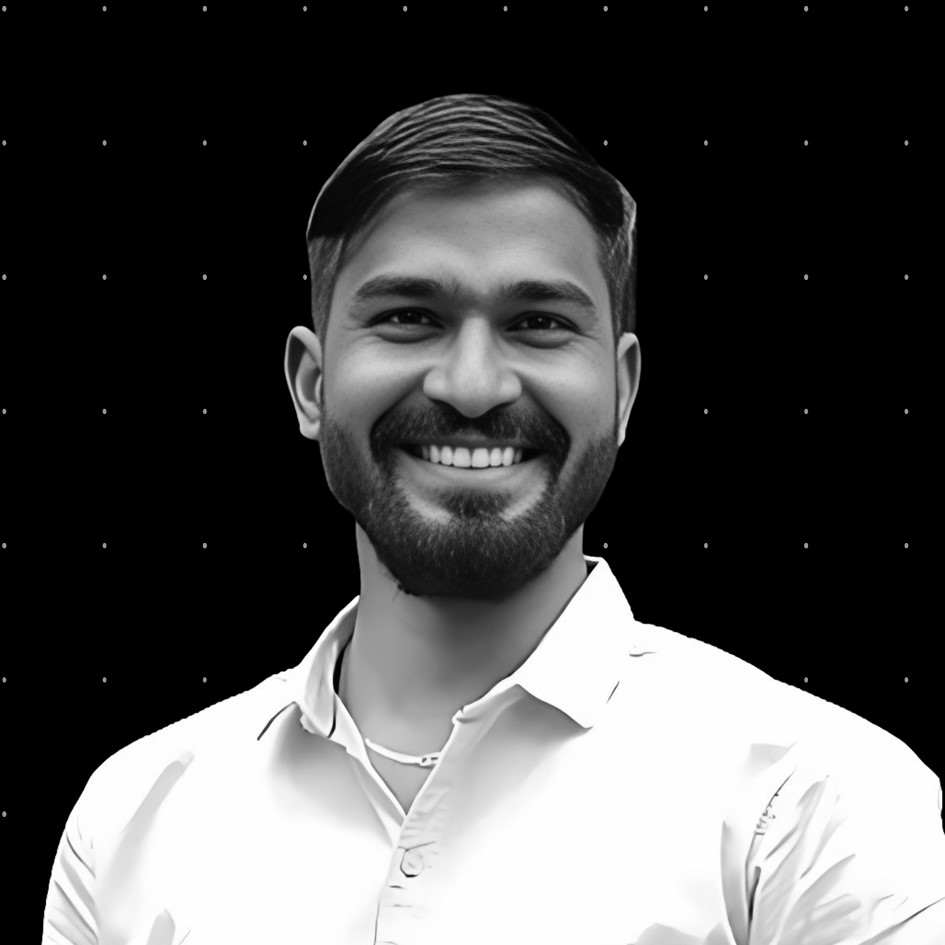

Have you ever found yourself solving a coding problem and thought, "Hmm, I’ve seen something like this before..."?
That’s where design patterns come in.
Design patterns are not magic spells or complex algorithms, they’re more like tried-and-true building blocks that experienced developers have used for years to solve common software problems. Let’s break it down.
So, What is a Design Pattern?
A design pattern is a general solution or blueprint for a commonly occurring problem in software design. It’s not a ready-made piece of code you can copy and paste. Instead, it’s a concept or a strategy - a way of approaching a problem that’s proven to work.
Design Patterns vs Algorithm
Let’s make one thing clear:
A design pattern is not an algorithm.
An algorithm is like a cooking recipe, it gives you detailed steps to prepare a dish. A design pattern is more like a cooking technique, like sauteing or braising, it helps you solve recurring problems in the kitchen, but doesn’t tell you what dish to make.
What Makes Up a Design Pattern?
Each design pattern generally includes these elements: ( in this series)
Purpose – A brief explanation of what the pattern is designed to achieve and the type of design challenge it addresses.
Reasoning – The context behind using the pattern, including examples that highlight its usefulness in solving common problems.
Layout – A visual or descriptive breakdown of how the parts of the pattern connect and work together.
Sample Code – A demonstration of how the pattern can be implemented in code, often using familiar programming languages. (we will use python)
Still Confused?
Imagine you’re running a busy coffee shop.
Every day, you face the same problems:
How do we take orders quickly?
How do we keep the counter organized?
How do we manage different drink recipes?
Over time, you develop routines to make things run smoothly:
You organize the counter with clearly labeled sections for cups, lids, and straws.
You have a set way of making each drink, so it’s quick and consistent.
You assign specific roles, one person takes orders, another makes coffee.
These routines aren’t new every time, they’re standard practices that help the coffee shop run efficiently.
In the same way, design patterns are standard solutions for common software problems. They help your code run smoothly, just like your coffee shop’s routines help keep things moving efficiently.
Why Should You Learn Design Patterns?
Here’s the truth:
You can write code for years without ever formally learning a single pattern.
And yet…
You might already be using them without knowing!
Design patterns are useful because:
They are proven solutions, tested by developers around the world.
They help you communicate with other developers using a shared vocabulary.
They teach you to think in terms of reusable, maintainable, object-oriented code.
Even if you don’t use every pattern directly, knowing them makes you a better problem-solver.
Types of Design Patterns (The Big 3)
Design patterns are grouped based on what they’re meant to solve:
1. Creational Patterns
Help you create objects in a way that’s flexible and reusable.
Think: How should I create this thing efficiently?
Examples pattern include:
Singleton
Factory Method
Builder
2. Structural Patterns
Help you build relationships between classes and objects.
Think: How should these pieces fit together?
Examples patterns include:
Adapter
Composite
Decorator
3. Behavioral Patterns
Help objects communicate and assign responsibilities effectively.
Think: Who should do what, and when?
Examples patterns include:
Observer
Strategy
Command
Final Thoughts
Design patterns aren’t something you force into your code, they’re something you reach for when a familiar problem pops up. They’re tools, not rules.
As you grow as a developer, you’ll start to recognize when and where to apply them, sometimes without even thinking about it.
In upcoming posts, we’ll explore each pattern type with real-world examples, simple code, and more analogies to keep things fun and easy to understand.
Subscribe to my newsletter
Read articles from Bhuvanesh Prasad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
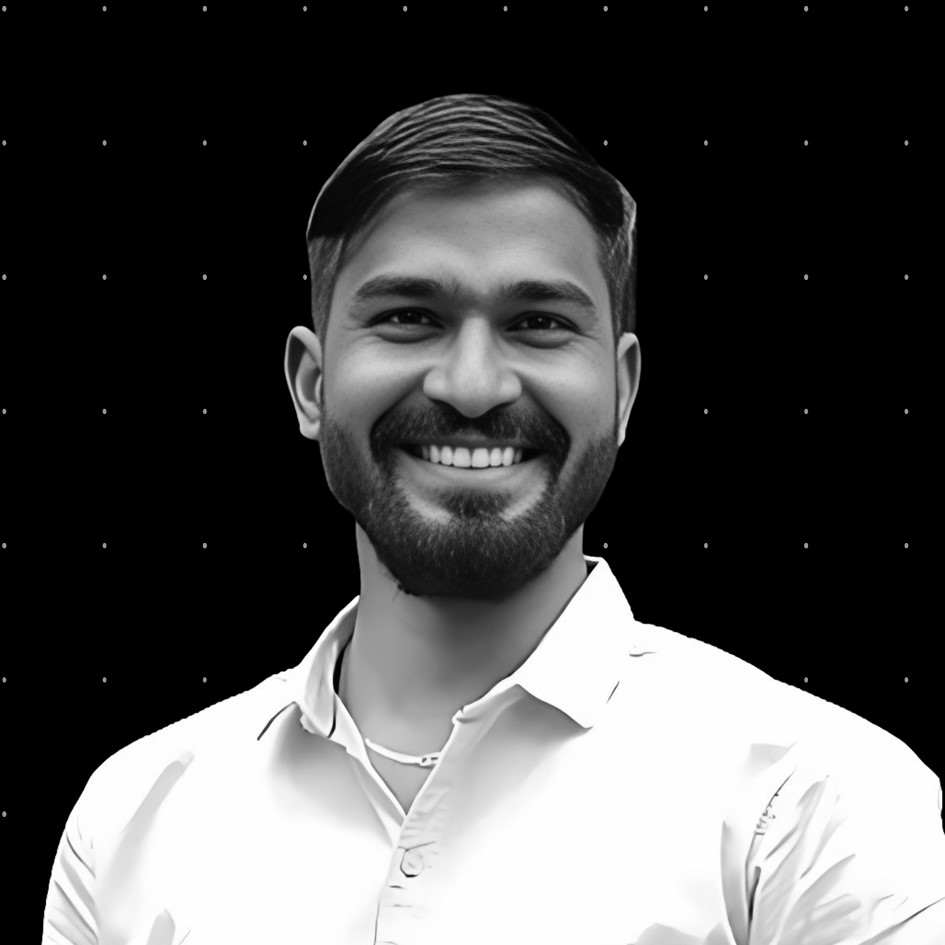