Understanding the Anti-Corruption Layer: A Comprehensive Guide
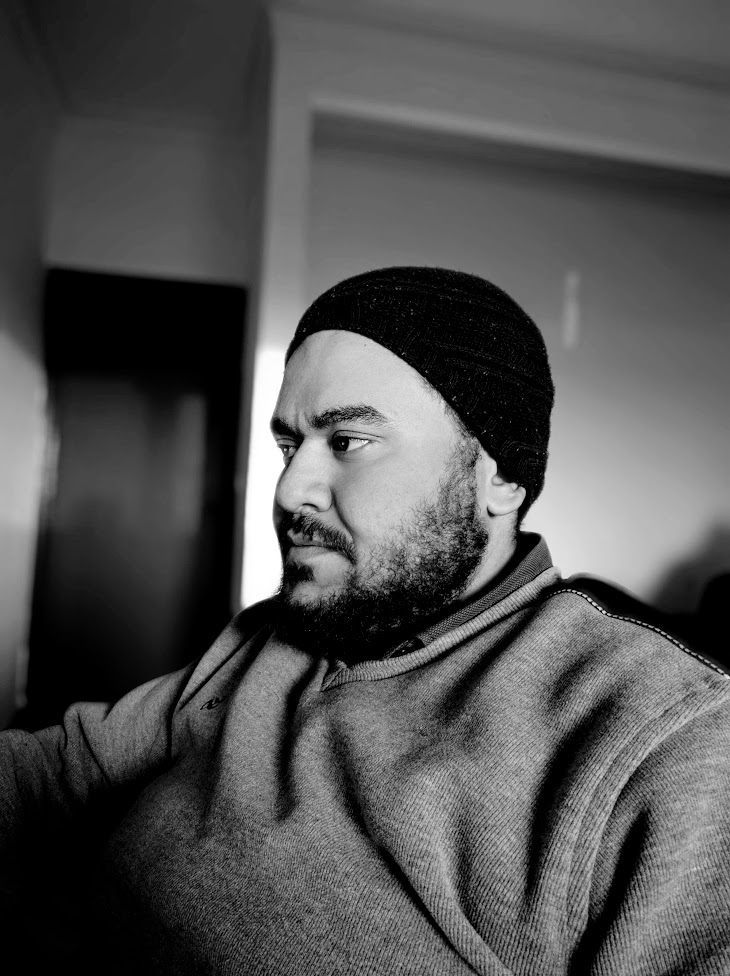
Abstract:
In complex, distributed software systems—especially those built on evolving microservices, legacy modernization initiatives, or multi-vendor integrations—ensuring domain integrity becomes a first-order architectural concern. This article offers a deep-dive, academically grounded exploration of the Anti-Corruption Layer (ACL) pattern, a core strategic pattern in Domain-Driven Design (DDD). We examine its motivation, theoretical underpinnings, structural mechanics, and practical applications, using real-world software architecture paradigms and antipattern analyses.
1. Introduction: Integration as a Source of Architectural Debt
In enterprise-grade software systems, integration is unavoidable. It arises from:
Legacy system interoperability
Third-party services and platforms (e.g., CRMs, ERPs, payment gateways)
Federated microservices owned by separate teams or organizations
However, while integration enables collaboration across systems, it also introduces semantic coupling, conceptual mismatch, and model contamination. This typically manifests as:
Codebases that reflect external design flaws
Domain logic corrupted by alien terminology
Increased effort in adapting to change
The Anti-Corruption Layer (ACL) was introduced by Eric Evans in his book Domain-Driven Design: Tackling Complexity in the Heart of Software to address this fundamental problem: how can a system integrate with another system without compromising its own model and design principles?
2. Conceptual Basis: The Philosophy Behind ACL
2.1 Ontological Independence
The central philosophical underpinning of ACL is ontological independence. Each software system (or bounded context) has its own conceptual model of the world. These models are not just data schemas but include vocabulary, assumptions, business rules, and worldviews.
To maintain ontological integrity, we must resist the temptation to conform to external definitions. ACLs allow each bounded context to remain Isolated, while enabling interaction.
"Integration should not mean submission." — Eric Evans (paraphrased)
2.2 Semantic Decoupling
The ACL pattern explicitly separates semantic layers:
The external system has its model (e.g.,
ExternalCustomer
)The internal domain has its model (e.g.,
Client
,AccountHolder
)The ACL translates, adapts, and shields
This translation prevents foreign semantics from leaking into the core business logic.
3. Structural Mechanics: What Makes an ACL?
An Anti-Corruption Layer is not a singular class or middleware component. It is a composite architectural layer with specific responsibilities.
3.1 Components of the ACL
Component | Responsibility |
Translator/Mappers | Convert external DTOs to internal value objects or entities |
Adapters | Implement interfaces used by internal services, delegating to external systems |
Facades/Gateways | Abstract external service protocol and contract |
Error Isolation | Manage error handling, logging, and fallback mechanisms |
Security Layer | Handle access tokens, credentials, and protocol security |
3.2 Logical Placement
ACLs are located at the interface between bounded contexts, or at the integration layer of your architecture. They form a boundary that isolates domain logic from technical and semantic details of external systems.
The ACL is part of your own system. It is not implemented by the external party.
4. Practical Instantiation: Implementing ACL in Modern Systems
4.1 Interface-Driven Design
An ACL should conform to internal interfaces, not external ones.
// Internal abstraction
type ClientVerificationService interface {
VerifyClient(id string) (VerificationResult, error)
}
Your ACL would implement this interface, even if the external service follows a different paradigm (e.g., REST, SOAP, gRPC).
4.2 Mapping Strategies
Use pure mapping layers (e.g., assemblers, mappers) to convert between models. Avoid passing external types across service boundaries.
func mapToInternalClient(ext ExternalCustomer) Client {
return Client{
Name: ext.FullName,
Email: normalizeEmail(ext.Email),
Status: translateStatus(ext.Code),
}
}
4.3 Protocol and Data Contract Abstraction
Use gateway or façade classes to shield the domain from transport concerns:
type ExternalAPI struct {
client *http.Client
baseURL string
}
func (api *ExternalAPI) GetCustomer(id string) (ExternalCustomerDTO, error) {
// Transport logic hidden from domain
}
5. ACL in Strategic DDD: Context Mapping
ACL fits into Strategic Design as a tool to preserve bounded context boundaries.
In a Conformist relationship, the internal model is dictated by the external. This is fast but risky.
In contrast, the ACL pattern represents a Customer-Supplier relationship, where the customer (you) builds a translator to maintain independence.
The ACL becomes a contract firewall.
This is essential when:
Integrating with legacy systems
Consuming external SaaS APIs
Bridging radically different models
6. Performance, Reliability, and Resilience Considerations
6.1 Performance Overhead
ACLs can introduce latency due to translation and abstraction, particularly if:
Protocols are non-native (e.g., SOAP in REST-based systems)
ACL performs extensive validation or transformation
Solutions:
Use in-memory caching for external calls
Offload translation where possible (e.g., pre-process via message queues)
6.2 Resilience Engineering
Use the ACL to embed robustness patterns, including:
Retry logic
Circuit breakers
Bulkheads
Fallbacks (graceful degradation)
Libraries: Hystrix (deprecated), Resilience4j, Spring Retry, or custom middleware
7. Advanced Use Case: ACL + Event-Driven Architecture
In event-based systems, ACLs can be used in two ways:
Event Translator: Consumes external events and translates them into your internal domain events
Event Producer ACL: Converts internal events into the format and semantics expected by an external consumer
This protects against:
Event schema drift
Versioning incompatibilities
Inconsistent causality assumptions
8. Pitfalls and Antipatterns
❌ 8.1 Leaky Abstractions
Allowing external DTOs or APIs to bleed into internal code undermines the ACL’s purpose.
Fix: Enforce boundaries through strict typing and dependency inversion.
❌ 8.2 Overuse in Simple Cases
Avoid using ACLs where simple data passthrough suffices. ACLs incur costs in terms of code volume and test surface area.
Fix: Apply ACLs only when the model gap justifies the complexity.
❌ 8.3 Bi-directional ACLs
Avoid building ACLs that must deeply understand both domains. This leads to tight coupling in disguise.
9. Testing and Maintenance
ACLs should be independently testable, including:
Contract tests against the external system
Unit tests for mappers and adapters
Fault injection tests to simulate partial failures
They also serve as a single point for version upgrades, e.g., when the external API moves from v1 to v2, only the ACL requires updates—not the entire domain model.
10. Conclusion: The ACL as an Intellectual and Architectural Firewall
The Anti-Corruption Layer is not just a technical construct—it is a strategic boundary, an intellectual firewall, and a manifestation of bounded context autonomy.
It empowers software teams to:
Maintain clean, expressive, and intention-revealing models
Safely consume external systems without becoming dependent on their structure
Create systems that remain internally stable, even as the external landscape evolves
"Build walls not to isolate—but to control what crosses over."
In a world increasingly defined by integration, the ACL is your shield against architectural erosion.
Subscribe to my newsletter
Read articles from Yousif Aziz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
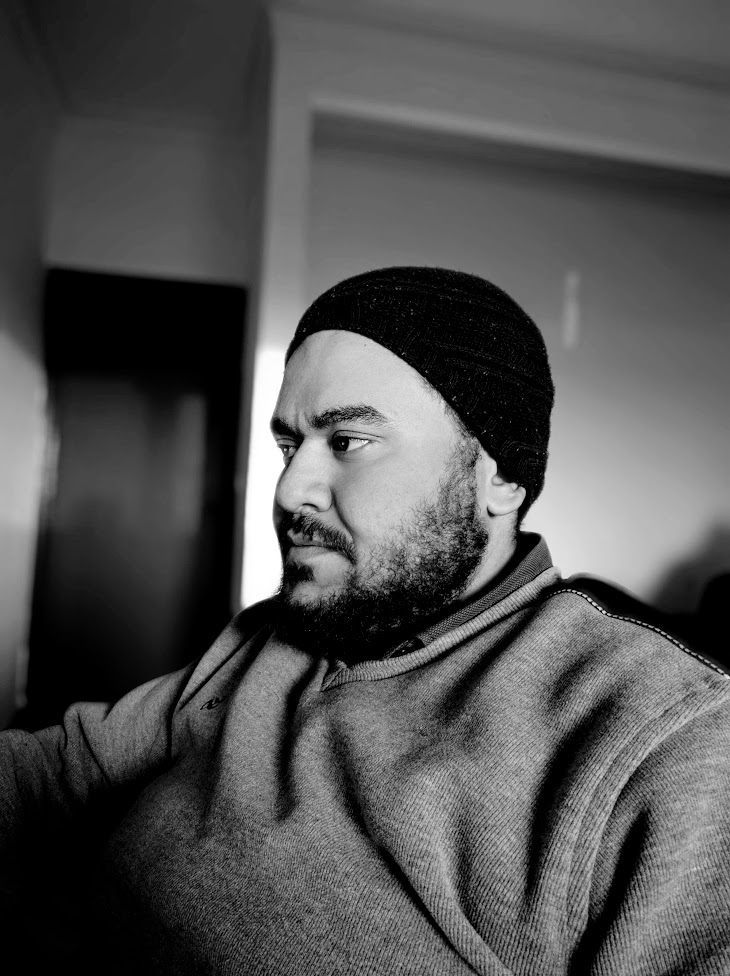
Yousif Aziz
Yousif Aziz
Mainly I've been working as a web developer for ten years, my experience in the back-end, front-end, and leadership. I also have a great experience with DevOps and Cloud Computing (AWS and Linux servers).