A Technical Guide to Test Mock Data: Levels, Tools, and Best Practices
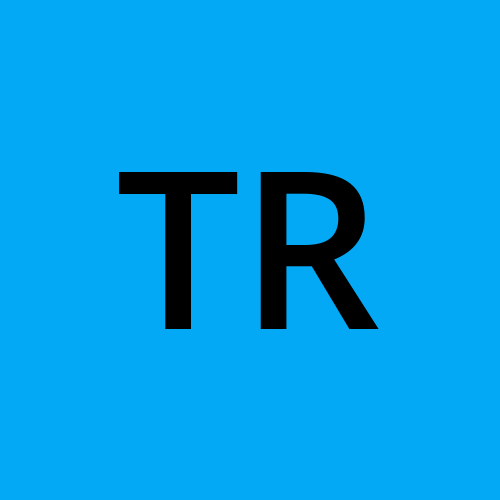

Mock data is the backbone of modern software development and testing. It allows developers to simulate real-world scenarios without relying on production data, ensuring security, efficiency, and reliability. Whether you’re testing APIs, building UIs, or stress-testing databases, mock data helps you isolate components, accelerate development, and catch bugs early.
In this blog, we’ll cover:
- Why mock data matters (with real-world examples from Tesla, Netflix, and more)
- Different levels of mock data (from foo/bar
to synthetic AI-generated datasets)
- Best tools for generating mock data (Mockaroo, Faker, JSONPlaceholder)
- Code samples in Python & JavaScript (executable examples)
- Common pitfalls & how to avoid them
Why Mock Data is Essential for Developers
Real-World Example: Tesla’s Self-Driving AI
Tesla trains its autonomous driving algorithms with massive amounts of labelled mock data. Instead of waiting for real-world accidents, Tesla simulates edge cases (e.g., pedestrians suddenly crossing) using synthetic data. This helps improve safety without risking lives
Key Benefits for Developers
No Dependency on Live APIs – Frontend devs can build UIs before the backend is ready.
Data Privacy Compliance – Avoid GDPR/HIPAA violations by never using real PII.
Faster Debugging – Reproduce bugs with controlled datasets.
Performance Testing – Simulate 10,000 users hitting your API without crashing prod.
Levels of Mock Data (From Simple to Production-Grade)
Level 1: Static Mock Data (foo/bar
Placeholders)
Use Case: Quick unit tests.
# Python Example: Hardcoded user data
user = {
"id": 1,
"name": "Test User",
"email": "test@example.com"
}
✅ Pros: Simple, fast.
❌ Cons: Not scalable, lacks realism.
Best Practices & Tips
Keep it minimal. Only mock the fields your unit under test actually needs.
Group your fixtures. Store them in a
/tests/fixtures/
folder for re-use across test suites.Version-pin schema. If you change your real schema, bump a “fixture version” so stale mocks break fast.
Level 2: Dynamic Mock Data (Faker.js, Mockaroo)
Use Case: Integration tests, demo environments.
// JavaScript Example: Faker.js for realistic fake data
import { faker } from '@faker-js/faker';
const mockUser = {
id: faker.string.uuid(),
name: faker.person.fullName(),
email: faker.internet.email()
};
console.log(mockUser);
Tools & Techniques
Faker libraries:
JavaScript: @faker-js/faker
Python: Faker
Ruby: faker
Mock servers:
Mockaroo for CSV/JSON exports
JSON Server for spinning up a fake REST API
Seeding:
- Always pass a fixed seed in CI (e.g. faker.seed(1234)) so CI failures are reproducible.
Level 3: Sanitized Production Data
Use Case: Performance testing, security audits.
-- SQL Example: Anonymized production data
SELECT
user_id,
CONCAT('user_', id, '@example.com') AS email, -- Masked PII
'***' AS password_hash
FROM production_users;
✅ Pros: Realistic, maintains referential integrity.
❌ Cons: Requires strict governance to avoid leaks.
Governance & Workflow
Anonymization pipeline:
Use tools like Aircloak Insights or write ETL-scripts to strip or hash PII.Subset sampling:
Don’t pull the entire production table—sample 1–5% uniformly or by stratified key to preserve distributions without bloat.Audit logs:
Track which team member pulled which snapshot and when; enforce retention policies.
Best Tools for Generating Mock Data
1. Mockaroo (Web-Based, Customizable Datasets)
Supports CSV, JSON, SQL exports.
REST API mocking (simulate backend responses).
# Python Example: Generate 100 fake users via Mockaroo API
import requests
API_KEY = "YOUR_API_KEY"
response = requests.get(f"https://api.mockaroo.com/api/users?count=100&key={API_KEY}")
users = response.json()
📌 Use Case: Load testing, prototyping 56.
2. Faker.js (Programmatic Fake Data)
// JavaScript Example: Generate fake medical records
import { faker } from '@faker-js/faker';
const patient = {
id: faker.string.uuid(),
diagnosis: faker.helpers.arrayElement(['COVID-19', 'Diabetes', 'Hypertension']),
lastVisit: faker.date.past()
};
📌 Use Case: Frontend dev, demo data 210.
3. JSONPlaceholder (Free Fake REST API)
# Example: Fetch mock posts
curl https://jsonplaceholder.typicode.com/posts/1
📌 Use Case: API testing, tutorials 910.
Advanced Mocking: Stateful APIs & AI-Generated Data
Example: Netflix’s Recommendation System
Netflix uses synthetic user behavior data to test recommendation algorithms before deploying them. This avoids spoiling real user experiences with untested models.
Mocking a Stateful API (Python + Flask)
from flask import Flask, jsonify
app = Flask(__name__)
users_db = []
@app.route('/users', methods=['POST'])
def add_user():
new_user = {"id": len(users_db) + 1, "name": "Mock User"}
users_db.append(new_user)
return jsonify(new_user), 201
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(users_db)
📌 Use Case: Full-stack testing without a backend.
Common Pitfalls & How to Avoid Them
Pitfall | Solution |
Mock data is too simplistic | Use tools like Faker for realism. |
Hardcoded data breaks tests | Use builders (e.g., PersonBuilder pattern) 2. |
Ignoring edge cases | Generate outliers (e.g. age: -1 , empty arrays). |
Mock != Real API behavior | Contract testing (Pact, Swagger). |
Conclusion
Mock data is not just a testing tool—it’s a development accelerator. By leveraging tools like Mockaroo, Faker, and JSONPlaceholder, developers can:
- Build much faster (no backend dependencies).
- Stay compliant (avoid PII risks).
- Find Bugs sooner (simulate edge cases).
FAQ
What is mock data?
Mock data is synthetic or anonymized data used in place of real production data for testing, development, and prototyping. It helps developers:
✅ Test APIs without hitting live servers.
✅ Build UIs before the backend is ready.
✅ Avoid exposing sensitive information (PII).When should I use mock data?
Unit/Integration Testing → Simple static mocks (
foo/bar
).UI Development → Dynamic fake data (Faker.js).
Performance Testing → Large-scale synthetic datasets (Mockaroo).
Security Testing → Sanitized production data (masked PII).
What’s the difference between mock data and real data?
| Mock Data | Real Data | | --- | --- | | Generated artificially | Comes from actual users | | Safe for testing (no PII) | May contain sensitive info | | Can simulate edge cases | Limited to real-world scenarios |
Subscribe to my newsletter
Read articles from Tvisha raji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
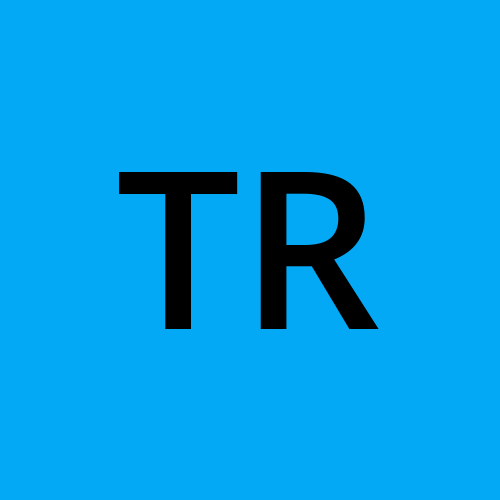