Why @EntityGraph Breaks Pagination in Spring Data JPA
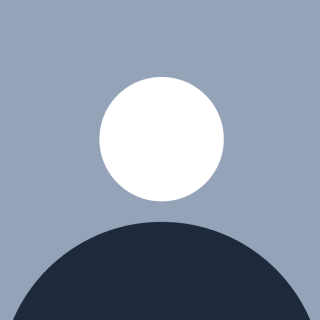
Table of contents
This is my second blog post — more of a mini blog, really — and I wanted to share a super frustrating issue I ran into recently with Spring Data JPA. I was using @EntityGraph
to avoid the N+1 problem, and it worked great — until I added pagination.
And just to make things worse, even LLMs and the GPTs of the world couldn’t help me much. The answers were vague, or plain wrong. This bug hid in plain sight until I dove into SQL logs myself.
@EntityGraph(attributePaths = {"orders"})
Page<Customer> findAll(Pageable pageable);
This looks fine, compiles, and even runs — but the results are wrong. I’d ask for 10 customers, and only get 7. And no, your query isn’t broken — it’s just Hibernate being sneaky.
The Problem: One-to-Many Joins Break Paging
If you eagerly fetch a collection (like @OneToMany) via @EntityGraph, Hibernate joins the child table. That means one parent row turns into multiple DB rows if the child list has entries.
Example:
@Entity
class Customer {
@OneToMany(mappedBy = "customer")
List<Order> orders;
}
@EntityGraph(attributePaths = {"orders"})
Page<Customer> findAll(Pageable pageable);
This produces a JOIN behind the scenes. So if Customer 1 has 3 orders, that’s 3 rows. But pagination (LIMIT 10) applies to rows, not entities — and now your results are off.
It was only after staring at the logs and breaking the query down manually that I understood what was really going on.
The Fix: Two-Step Fetch
Here’s what worked for me:
Page<Long> ids = customerRepo.findIdsOnly(pageable);
List<Customer> customers = customerRepo.findAllByIdIn(ids.getContent());
Apply @EntityGraph on the second query. Clean, correct, and doesn’t mess up paging.
TL;DR
• Don’t mix @EntityGraph and pagination directly when fetching collections.
• Hibernate joins duplicate rows and breaks paging.
• Even LLMs weren’t helpful here — SQL logs were.
• Use a two-step ID fetch instead.
Hope this saves you time. It sure cost me enough of mine.
Subscribe to my newsletter
Read articles from Shivansh Narayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by