Ways to Implement the Backend for Frontend (BFF) Pattern for Modern Applications
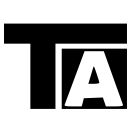
5 min read
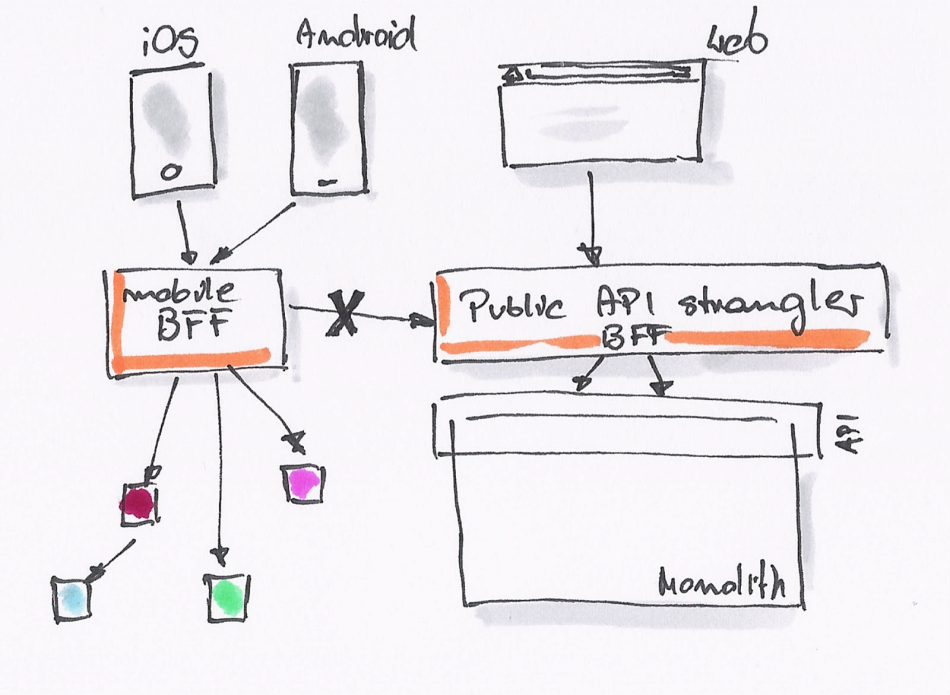
1. What is the Backend for Frontend (BFF) Pattern?
The BFF pattern is a design concept where each frontend (whether mobile, web, or other platforms) interacts with its own tailored backend service. This backend aggregates data from various microservices and presents it in the format the frontend expects. The aim is to decouple the frontend from the complexity of microservices, improving performance and providing flexibility for frontend teams.
1.1. Key Features of BFF
Frontend-Specific Logic: Each frontend (mobile app, web client, etc.) gets its own backend that understands its needs.
Data Aggregation: The BFF aggregates and transforms data from multiple sources into a single response for the frontend.
Performance Optimization: Reducing unnecessary data for the frontend, only returning what's needed.
Here’s a basic architecture showing how BFF works:
[Frontend] --> [Backend for Frontend (BFF)] --> [Microservices]
[Mobile App] --> [Backend for Frontend (BFF)] --> [Microservices]
Each frontend interacts with its respective BFF layer, which in turn communicates with microservices or external APIs.
1.2. Why Use the BFF Pattern?
The BFF pattern addresses several challenges faced when frontend and backend teams scale:
- Frontend Optimization: Different frontends (mobile vs. web) have different needs. The BFF ensures each frontend gets exactly what it requires, optimizing network traffic.
- Backend Simplicity: The complexity of backend systems is hidden from the frontend, simplifying their development.
- Separation of Concerns: By separating frontends from backend microservices, teams can independently evolve their services.
1.3. Best Practices for Implementing the BFF Pattern
Separation of Logic: Ensure that the business logic remains in the core microservices and that the BFF focuses solely on data transformation and aggregation.
Security Considerations: Always secure the BFF by implementing authentication and authorization. Since the BFF is an intermediary between the frontend and backend, it must ensure proper handling of sensitive data.
Rate Limiting and Caching: Optimize performance by implementing caching strategies at the BFF layer and ensuring that the backend services aren't overwhelmed by repeated requests.
Consistency Across Frontends: Although each BFF is tailored for a specific frontend, ensure that there is consistency in how core services are accessed and how data is formatted.
1.4. Example: Implementing BFF in a Node.js Environment
Let's consider a Node.js-based BFF implementation that aggregates data from two microservices: one for user information and another for user orders.
Backend for Frontend Code Example:
const express = require('express');
const axios = require('axios');
const app = express();
const PORT = 3000;
// User service API
const userService = 'http://user-service.local/api/users';
// Order service API
const orderService = 'http://order-service.local/api/orders';
// BFF endpoint for frontend
app.get('/bff/user-data', async (req, res) => {
try {
// Fetch user data from two microservices
const [userInfo, userOrders] = await Promise.all([
axios.get(${userService}/${req.query.userId}
),
axios.get(${orderService}/${req.query.userId}
)
]);
// Aggregate and send the response to the frontend
res.json({
user: userInfo.data,
orders: userOrders.data
});
} catch (error) {
console.error('Error fetching data from services', error);
res.status(500).json({ error: 'Internal Server Error' });
}
});
app.listen(PORT, () => {
console.log(BFF running on port ${PORT}
);
});
Explanation:
- The BFF exposes a single /bff/user-data endpoint for the frontend.
- It fetches data from two microservices (user-service and order-service) simultaneously using axios.
- The data is aggregated and returned in a format expected by the frontend, ensuring a simplified response.
2. Key Considerations When Implementing BFF
2.1. Security
Since the BFF is an intermediary between frontends and sensitive backend services, security is critical. Consider using:
- OAuth2 or JWT for securing the communication between the frontend and the BFF.
- SSL/TLS to encrypt traffic between the frontend, BFF, and backend services.
For example, adding a JWT token verification middleware in Express:
const jwt = require('jsonwebtoken');
app.use((req, res, next) => {
const token = req.headers['authorization'];
if (!token) {
return res.status(403).send('Token missing');
}
jwt.verify(token, 'secret-key', (err, decoded) => {
if (err) {
return res.status(401).send('Unauthorized');
}
req.user = decoded;
next();
});
});
2.2. Scalability
To ensure that your BFF can scale effectively:
- Horizontal Scaling: Deploy multiple instances of the BFF to handle increased load.
- Load Balancing: Distribute requests across multiple instances using load balancers like NGINX or AWS ALB.
- Service Discovery: Implement service discovery tools like Consul or Eureka to dynamically locate microservices when they scale.
2.3. Managing Dependencies Between BFF and Microservices
It’s crucial that BFF services do not become tightly coupled to backend microservices. If microservice APIs change frequently, it can cause a ripple effect on BFF services. Consider:
- API Versioning: Adopt versioning strategies to avoid breaking changes.
- Fallback Strategies: Use fallback or default responses when a backend service is unavailable.
2.4. Monitoring and Observability
For maintaining the health and performance of your BFF, integrate monitoring solutions like Prometheus or Grafana, and log requests for observability.
Here’s an example of integrating Prometheus with a Node.js BFF:
const promClient = require('prom-client');
const collectDefaultMetrics = promClient.collectDefaultMetrics;
// Enable collection of default metrics
collectDefaultMetrics();
// Expose metrics endpoint for Prometheus scraping
app.get('/metrics', async (req, res) => {
res.set('Content-Type', promClient.register.contentType);
res.end(await promClient.register.metrics());
});
3. Conclusion
The Backend for Frontend (BFF) pattern is an effective way to streamline communication between frontend applications and backend microservices. By creating a dedicated backend for each frontend, you ensure that frontend developers can optimize their APIs without being overwhelmed by backend complexities. This article has explored the BFF pattern, its benefits, and best practices, providing real-world code examples for a better understanding. If you have any questions or need further clarifications, feel free to leave a comment below!
Read more at : Ways to Implement the Backend for Frontend (BFF) Pattern for Modern Applications
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
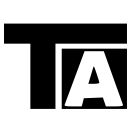
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.