OAuth or JWT? Everything Developers Need to Know in 2025
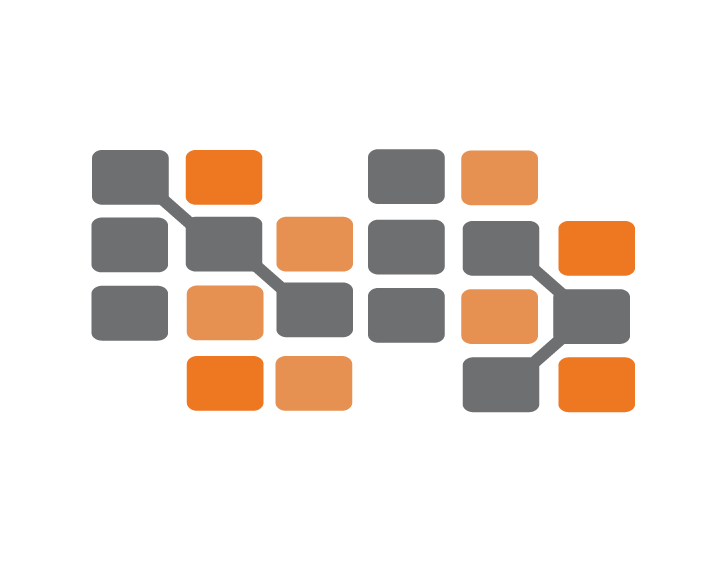
Table of contents

https://www.nilebits.com/blog/2025/05/oauth-jwt-everything-need-know-2025/
In contemporary software development, authorization and authentication are essential elements. OAuth and JWT (JSON Web Tokens) are two of the most widely used protocols and standards available to developers today. Despite their frequent usage together, they have distinct functions and cannot be substituted for one another. This article gives a thorough side-by-side analysis of OAuth and JWT, explaining how each functions, when to use it, and why it's important for contemporary application security.
Whether you're developing a microservices architecture, a mobile backend, or a SaaS product, understanding the key differences and practical implementations of OAuth and JWT will help you design more secure and scalable systems.
What is OAuth?
OAuth (Open Authorization) is an open standard protocol for authorization, commonly used to grant third-party applications delegated access to resources without exposing user credentials.
Key Features
Delegated access (authorization without sharing passwords)
Token-based access (Access Tokens)
Supports multiple grant types (Authorization Code, Client Credentials, Password, etc.)
Often used in conjunction with OpenID Connect for authentication
Common Use Case
Allowing a user to log in to your application using their Google or Facebook account.
What is JWT?
JWT (JSON Web Token) is an open standard (RFC 7519) for securely transmitting information between parties as a JSON object. It is commonly used for authentication and information exchange.
Key Features
Self-contained and stateless
Base64-encoded JSON object with a signature
Supports HMAC or RSA encryption
Commonly used in stateless authentication mechanisms
Common Use Case
Storing user session data in a signed token to avoid database lookups for each request.
OAuth and JWT: Are They the Same?
No. OAuth is a protocol, and JWT is a token format.
OAuth can use JWT as the format for access tokens, but it doesn’t have to. Similarly, JWTs can be used independently from OAuth for custom authentication systems.
When to Use OAuth
Use OAuth when:
You need delegated authorization
You’re exposing APIs to third-party developers
You require scope-based access to resources
You need to separate authentication (OpenID Connect) and authorization
When to Use JWT
Use JWT when:
You want stateless session management
You want to embed user claims directly in a token
You need fast, scalable authentication
You’re implementing Single Sign-On (SSO)
OAuth vs. JWT: Tabular Comparison
Feature | OAuth | JWT |
Definition | Authorization protocol | Token format for authentication |
Purpose | Delegated access to APIs | Transmitting identity and claims |
Stateless | Can be stateful or stateless | Stateless |
Token Format | Varies (can be JWT, opaque, etc.) | Always JWT format |
Used For | Authorization | Authentication and Information exchange |
Token Storage | Server or client | Client |
Revocation | Requires state (e.g., token blacklist) | Difficult (requires token expiration) |
OAuth with JWT: How They Work Together
In most modern systems, OAuth 2.0 issues JWTs as access tokens. This combines the delegation power of OAuth with the stateless nature of JWT.
Example Flow:
User logs in via Google (OAuth)
Google issues an access token (JWT)
Your app validates and parses the JWT
User is granted access based on the token's claims
Real-World Example: Using OAuth with JWT in Node.js
Here's a basic example using Node.js, Express, and passport.js with Google OAuth and JWT.
1. Install Required Packages
npm install express passport passport-google-oauth20 jsonwebtoken dotenv
2. OAuth Strategy Setup
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth20').Strategy;
const jwt = require('jsonwebtoken');
passport.use(new GoogleStrategy({
clientID: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
callbackURL: "/auth/google/callback"
}, (accessToken, refreshToken, profile, done) => {
const token = jwt.sign({
id: profile.id,
name: profile.displayName
}, process.env.JWT_SECRET, { expiresIn: '1h' });
return done(null, token);
}));
3. Express App Integration
const express = require('express');
const passport = require('passport');
require('./auth');
const app = express();
app.get('/auth/google', passport.authenticate('google', {
scope: ['profile']
}));
app.get('/auth/google/callback',
passport.authenticate('google', { session: false }),
(req, res) => {
res.send(`Your token: ${req.user}`);
}
);
app.listen(3000, () => console.log('Server running on http://localhost:3000'));
JWT Verification in API Gateway
const jwt = require('jsonwebtoken');
function verifyJWT(req, res, next) {
const token = req.headers.authorization?.split(' ')[1];
if (!token) return res.sendStatus(403);
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
req.user = decoded;
next();
} catch {
res.sendStatus(401);
}
}
Security Considerations
JWT
Always use HTTPS to prevent token interception
Set short expiration times
Avoid storing sensitive data in the token
OAuth
Always validate redirect URIs
Implement proper token revocation
Consider using PKCE for public clients
References
Conclusion
Despite frequently being used interchangeably, OAuth and JWT have different but complimentary functions. JWT offers a scalable, self-contained method of transmitting identification, whereas OAuth gives safe delegated access. They serve as the foundation for contemporary authentication systems.
Understanding their roles, strengths, and limitations is crucial for any developer building secure, scalable, and performant applications. Armed with this knowledge, you're better equipped to design systems that are both user-friendly and secure.
https://www.nilebits.com/blog/2025/05/oauth-jwt-everything-need-know-2025/
Subscribe to my newsletter
Read articles from Nile Bits directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
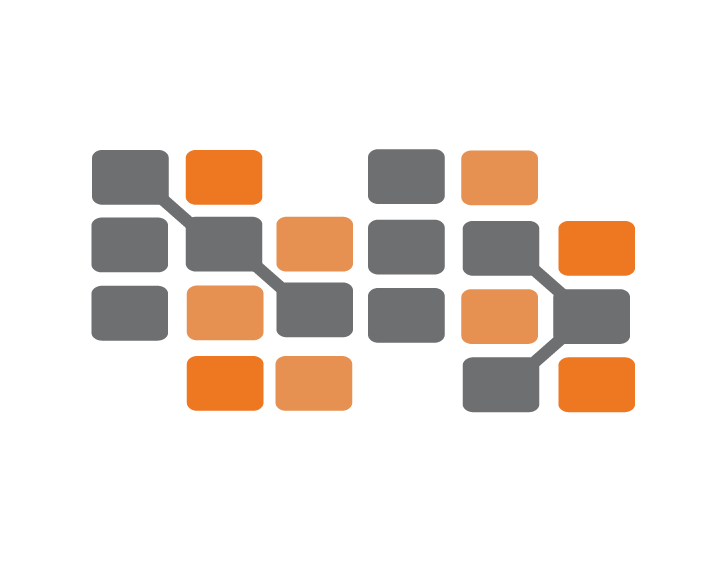
Nile Bits
Nile Bits
Nile Bits is a software company, focusing on outsourcing software development and custom software solutions. Our outsourcing software services and solutions are designed with a focus on secure, scalable, expandable and reliable business systems. Via our low cost, high quality and reliable outsourcing software services, we provide to our clients value for money and therefore client satisfaction.