Streamlining Data Management: Exploring Laravel Cascade Soft Deletes
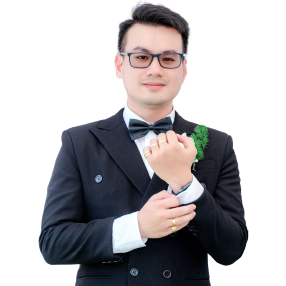

Introducing Laravel Cascade Soft Deletes: Efficient Soft Delete Management
When developing web applications with Laravel, managing related data across database tables is a critical task. For instance, when deleting a parent record, such as a blog post, you often want to remove associated child records, like comments, to keep your data clean and consistent. The laravel-cascade-soft-deletes package by Michael Dyrynda addresses this challenge effectively, especially when leveraging Laravel’s soft deletes feature.
Soft Deletes and the Challenge
Laravel’s soft delete functionality, provided by the SoftDeletes
trait, allows you to mark records as "deleted" without removing them from the database. This is invaluable for restoring data later. However, when using soft deletes, you lose the ability to automatically delete related child records through database foreign key constraints like ON DELETE CASCADE
.
The laravel-cascade-soft-deletes package bridges this gap. It enables automatic soft deletion of child records when a parent record is soft deleted, supporting cascading soft deletes for nested relationships.
Key Features
Automatic child record soft deletion: When a parent record is soft deleted, all related child records (defined in the
$cascadeDeletes
array) are also soft deleted.Cascading soft deletes: If child records also use the
CascadeSoftDeletes
trait, their children (grandchildren) will be soft deleted, creating a chain of cascading deletions.Customizable data fetching: Supports methods like
get
,cursor
,lazy
, orchunk
to optimize performance when handling large datasets.Robust error handling: The package throws a
LogicException
if the model lacks theSoftDeletes
trait or if defined$cascadeDeletes
relationships are invalid or non-existent.
Installation and Usage
Installation
Install the package via Composer:
composer require dyrynda/laravel-cascade-soft-deletes
Example Usage
Here’s how to integrate the package into a Post
model to automatically soft delete related comments:
<?php
namespace App;
use App\Comment;
use Dyrynda\Database\Support\CascadeSoftDeletes;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
use SoftDeletes, CascadeSoftDeletes;
protected $cascadeDeletes = ['comments'];
protected $dates = ['deleted_at'];
public function comments()
{
return $this->hasMany(Comment::class);
}
}
After configuration, soft deleting a post:
$post = App\Post::find($postId);
$post->delete();
This will soft delete the post and all related App\Comment
records. If the Comment
model also uses CascadeSoftDeletes
, its child records (if any) will be soft deleted as well.
Important Notes
Data restoration: Restoring a parent record does not automatically restore soft-deleted child records, as there’s no way to distinguish whether they were deleted via cascading or independently.
Performance: For relationships with large datasets, you can customize
$fetchMethod
(e.g.,chunk
orlazy
) and$chunkSize
to optimize performance.
Support and Community
If you encounter issues with the package, reach out to the author, Michael Dyrynda, via Twitter or report problems on the GitHub issue tracker. Contributions via pull requests are also welcome if you spot bugs or want to enhance the package.
Treeware - Contributing to the Environment
The laravel-cascade-soft-deletes package is part of Treeware, encouraging users to "buy a tree" if the package is used in production. This initiative supports local communities and restores wildlife habitats. Contribute at plant.treeware.earth.
Conclusion
The laravel-cascade-soft-deletes package is a powerful and user-friendly solution for managing soft deletes in Laravel applications with complex relationships. By automating the soft deletion of related records and offering flexible customization, it’s an essential tool for Laravel developers aiming to maintain clean and efficient data. Try it today and contribute to a greener planet through Treeware!
Reference: GitHub - laravel-cascade-soft-deletes
Subscribe to my newsletter
Read articles from David Nguyen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
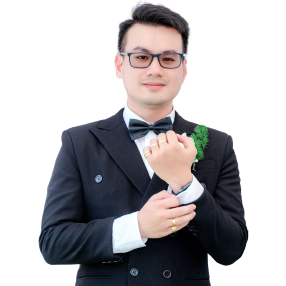
David Nguyen
David Nguyen
A passionate full-stack developer from @ePlus.DEV