Setting up your JavaScript Environment (Complete Guide)
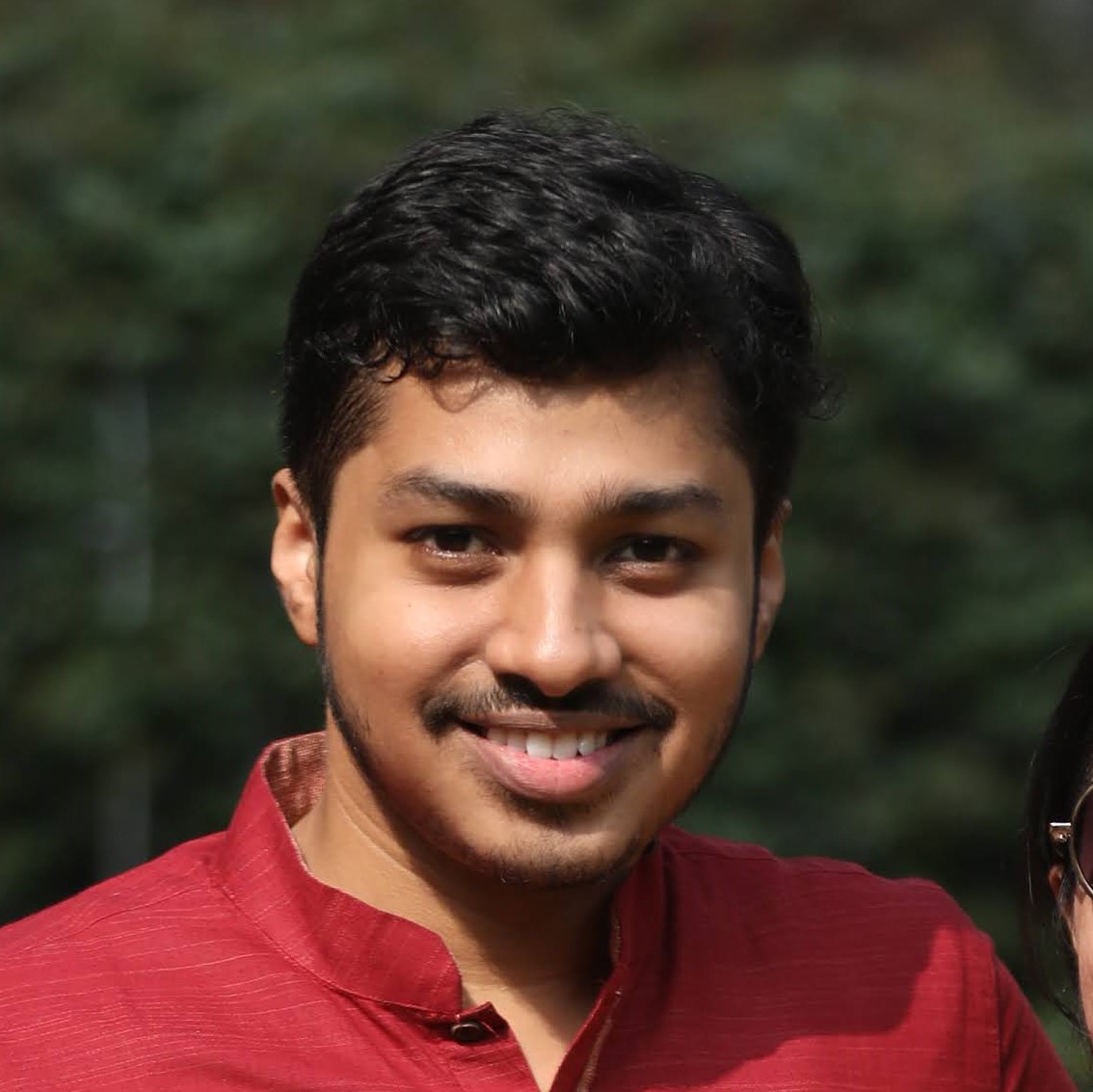

Welcome back to our JavaScript journey! In our first post, we explored why JavaScript is worth learning in 2025. Now it's time to set up your coding environment so you can start building real projects.
Don't worry—this won't involve complicated installations or technical hurdles. I'll walk you through options ranging from zero-setup solutions to professional-setup environments, so you can choose what works best for you.
Option 1: Code in the Browser (No Installation Required)
The easiest way to start coding JavaScript is directly in your browser. Here are four excellent options:
CodePen
CodePen is one of the most simple and easy to use websites which you can use to create, share, and discover front-end code snippets.
Why CodePen is great for beginners:
Zero setup required
Instant preview of your code results
Split-screen view of HTML, CSS, and JavaScript
Easy to share your creations with friends or on social media
Huge library of examples to learn from
How to get started with CodePen:
Go to codepen.io
Click "Create Pen" (you can sign up later)
You'll see three panels: HTML, CSS, and JavaScript
Focus on the JavaScript panel for now
Type your code and see the results instantly in the preview window
Try writing this in the JavaScript panel:
const message = "My first JavaScript project!";
document.body.innerHTML = `<h1>${message}</h1>`;
JSFiddle
JSFiddle is similar to CodePen but with a slightly different interface and feature set.
Why JSFiddle works well:
Clean, minimalist interface
Good documentation features
External resource integration
Version control for your code snippets
Replit
Replit is a more powerful browser-based IDE that supports multiple languages.
Why Replit may be your best choice:
Full programming environment in the browser
Terminal access
Support for npm packages (we'll cover these later in the series)
Collaborative coding features
More similar to professional development environments
CodeSandbox
CodeSandbox is another excellent browser-based option that's gained huge popularity in recent years.
Why CodeSandbox stands out:
Specializes in creating web application environments
Excellent for React, Vue, and other framework development
GitHub integration
Deployment previews
Real-time collaboration features
Professional-grade development experience in the browser
Option 2: Local Development Environment (A more Professional approach)
While browser tools are convenient, professional developers typically work on their local machines. Setting this up is surprisingly easy!
Step 1: Install a Code Editor
While there are many code editors/IDEs, Visual Studio Code (VS Code) is the most popular code editor in 2025, and my personal favourite too!
Why VS Code is awesome:
Free and powerful
Huge extension ecosystem
Excellent JavaScript support
Built-in terminal
GitHub integration
Live preview capabilities
Installation steps:
Go to code.visualstudio.com
Download the version for your operating system (Windows, Mac, or Linux)
Run the installer and follow the prompts
Launch VS Code once installed
The above steps guide you through installing VS Code, but you're free to use any code editor you prefer. Developing a JavaScript project isn't dependent on the choice of code editor.
(Optional) Recommended VS Code extensions for JavaScript beginners:
Live Server
Prettier - Code formatter
ESLint
These VS Code extensions will make your life easier while working on a project. There are many more which you can install later, but for now, I would strongly recommend you to install the above three.
To install extensions, click the Extensions icon in the sidebar (it looks like four squares), search for the extension name, and click "Install."
Step 2: Create your first project folder
Now let's create a place to store your JavaScript projects:
Create a folder called "javascript-projects" somewhere easy to find (like your Documents folder)
Inside that folder, create another folder called "project-01"
Open VS Code
Go to File > Open Folder and select your "project-01" folder
Create three new files:
index.html
script.js
styles.css
Step 3: Set up a Basic Project Structure
Let's create a minimal project structure.
Don’t worry too much if you don’t understand even a single line of code below. This is just to setup your local coding environment and get you up and running. We’ll cover everything in detail in coming blog posts in this series.
Copy this code into your index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First JavaScript Project</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>JavaScript Project</h1>
<div id="output"></div>
<script src="script.js"></script>
</body>
</html>
Now add this to your script.js
file:
// This is a JavaScript comment
const output = document.getElementById('output');
output.innerHTML = `<p>Hello from JavaScript! Today is ${new Date().toLocaleDateString()}</p>`;
// Let's add a button dynamically
const button = document.createElement('button');
button.textContent = 'Click me!';
button.addEventListener('click', function() {
alert('Button clicked! JavaScript is working!');
});
output.appendChild(button);
Finally, add some basic styling in styles.css
:
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
max-width: 800px;
margin: 0 auto;
padding: 20px;
line-height: 1.6;
}
#output {
background-color: #f4f4f4;
padding: 20px;
border-radius: 5px;
margin-top: 20px;
}
button {
background-color: #4CAF50;
color: white;
border: none;
padding: 10px 15px;
border-radius: 4px;
cursor: pointer;
margin-top: 10px;
}
button:hover {
background-color: #45a049;
}
Step 4: Run Your Project
With the Live Server extension installed in VS Code:
Right-click on your
index.html
file in the explorer panelSelect "Open with Live Server"
Your default browser will open with your project running
Any changes you make to your code will automatically refresh in the browser
Understanding Browser Developer Tools
One of the most powerful features of modern browsers is their developer tools. Let's learn the basics:
Opening Developer Tools
Chrome/Edge: Press F12 or Ctrl+Shift+I (Cmd+Option+I on Mac)
Firefox: Press F12 or Ctrl+Shift+I (Cmd+Option+I on Mac)
Safari: Press Cmd+Option+I
The Console Tab
The Console is your JavaScript playground and debugging tool. It allows you to:
See JavaScript errors and warnings
Log messages from your code
Execute JavaScript commands directly
Inspect variable values
Try typing these commands in your browser's console:
console.log("Hello from the console!");
2 + 2
document.querySelector("h1").style.color = "blue";
The Network Tab
The Network tab is crucial for understanding how your application communicates with servers. This will be especially important when we learn about AJAX, Promises, and async/await in future lessons.
What you can do in the Network tab:
Monitor all network requests (API calls, image loading, etc.)
See request/response headers and data
Check response status codes (200 for success, 404 for not found, etc.)
Measure loading times and performance
Simulate different network conditions (3G, offline mode)
To explore this feature:
Open Developer Tools and click the Network tab
Reload the page to see all resources being loaded
Click on any request to see detailed information
The Network tab will become your best friend when debugging API calls later in our series!
The Elements Tab
This shows you the HTML structure of the page and lets you:
Inspect HTML elements
See and modify CSS styles in real-time
Test different layouts and designs
The Sources Tab
This is where you can:
See all your JavaScript files
Set breakpoints for debugging
Step through code execution line by line
Watch variables as they change
Choosing the right setup for you
If you're very new to coding, I recommend starting with CodePen or JSFiddle for the first few lessons. They let you focus on learning JavaScript without worrying about file structures.
Once you're comfortable with the basics (in about a week or two), transition to the local VS Code setup. This will prepare you for more complex projects and professional development.
Next Steps
Congratulations! You now have a working JavaScript development environment. In our next post, we'll dive into JavaScript basics: variables, data types, and operations—the building blocks of all JavaScript applications.
Homework Assignment: Try modifying the example code to display your name and a custom message when the button is clicked. If you get stuck, post in the comments and I'll help you out!
What setup will you be using to follow along with this series? Let me know in the comments below!
Subscribe to my newsletter
Read articles from Shubhaw Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
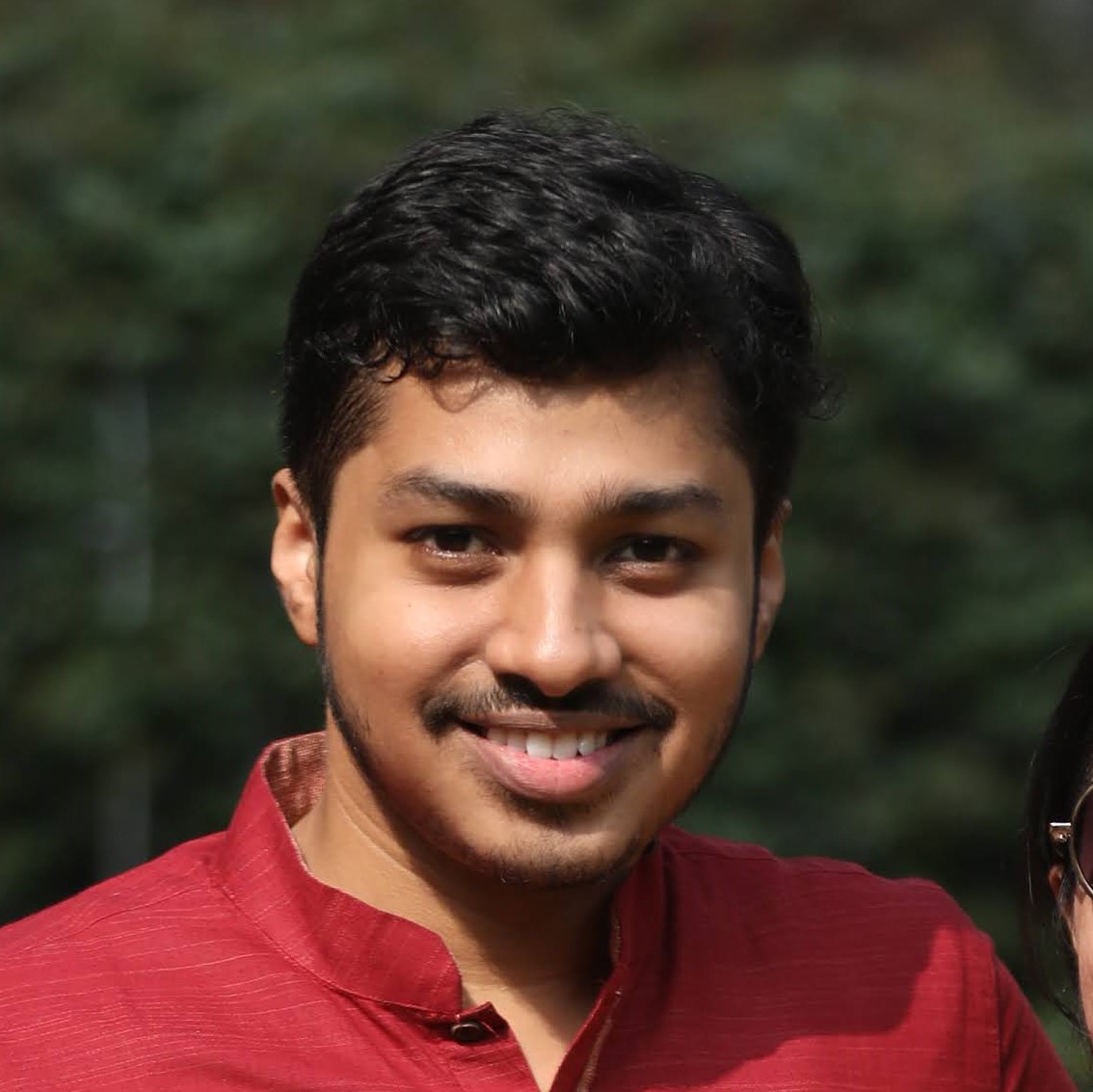
Shubhaw Kumar
Shubhaw Kumar
Software Engineer at Microsoft, India