JavaScript Basics: Variables, Data Types, and Operations
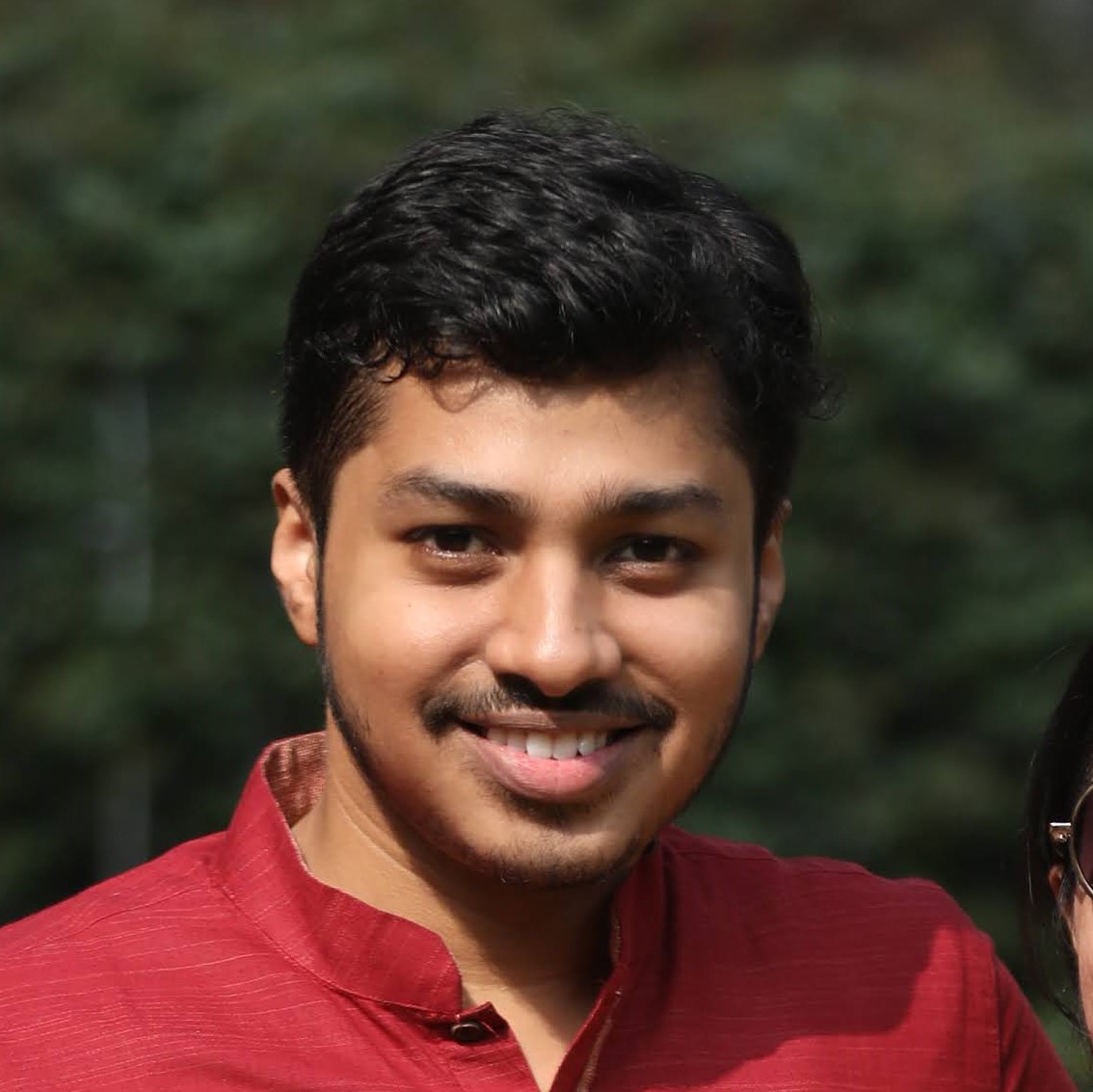

Welcome to the third post in our JavaScript journey! Now that you've set up your coding environment, it's time to learn the fundamental building blocks of JavaScript: variables, data types, and basic operations.
Think of these concepts as the alphabet and basic vocabulary of JavaScript—once you understand them, you can start forming more complex "sentences" in code.
Understanding Variables: Containers for your data
In JavaScript (and in most of the programming languages), variables are containers that store data values. Think of them as labeled boxes where you can put different things.
Declaring Variables
JavaScript gives us three ways to declare variables:
1. let
- The Standard Choice.
It should be used, when you need to change/reassign values. You can also delay the initialization.
let username = "shubhaw"; // initialization and value assignment done at the same step
let score; // not assigning any value initially
username = "learnWithShubhaw"; // reassigned value
score = 90; // assigned value now
2. const
- For values that shouldn't change
When you use const
, you cannot change the variable’s value anytime later.
const API_KEY = "abc123xyz";
const MAX_ATTEMPTS = 3;
API_KEY = "pqrs789"; // Error! Cannot reassign a constant
const TIMEOUT; // Error! Missing initializer in const declaration
3. var
- The Legacy Option
You can declare variables without initializing and can be reassigned anytime later, very similar to let
.
var message = "Hello world";
While
var
still works, it's generally better to uselet
andconst
in modern JavaScript due to their improved scoping rules. We would discuss about scoping in depth in future posts.
Variable Naming Conventions
JavaScript commonly uses camelCase for variable names (first word lowercase, then capitalize the first letter of each subsequent word):
let firstName = "Alex";
let totalItemCount = 5;
JavaScript variable names:
are case sensitive (
score
is different fromScore
)must only start with a letter, underscore (_) or dollar sign ($)
cannot be reserved keywords like
function
orreturn
Good variable names make your code easier to understand, and naming the variables is one of the most basic skill which young developers lack.
✅ Good variable names:
firstName
totalScore
isLoggedIn
❌ Bad variable names:
x
(too vague)a1
(meaningless)thingamajig
(unclear)
JavaScript Data Types: What can variables hold?
JavaScript has several built-in data types that determine what kind of data a variable can contain.
Primitive Data Types
These are the basic, immutable data types in JavaScript:
1. String - Text Values
Strings are text, enclosed in single or double quotes. Modern JavaScript also allows template literals with backticks, which enable multi-line strings and embedded expressions:
let message = "Hello, world!";
let username = 'javascriptLearner';
let greeting = `Hello, ${username}!
Welcome to JavaScript.`;
2. Number - Numeric Values
Unlike some programming languages, JavaScript has just one number type for integers and decimals:
let age = 24;
let price = 19.99;
let negativeValue = -42;
3. Boolean - True/False Values
Booleans represent logical values - true
or false
:
let isOnline = true;
let hasErrors = false;
4. Undefined
When a variable is declared but not assigned a value:
let futureValue;
console.log(futureValue); // undefined
5. Null
Represents the intentional absence of any value:
let emptyValue = null;
6. Symbol
A newer primitive type for creating unique identifiers:
let uniqueId = Symbol('id');
We won't focus much on Symbol here, but it's good to know it exists.
7. BigInt
For working with very large integers:
let hugeNumber = 9007199254740991n;
Non-Primitive (Complex) Data Types
These can hold multiple values and are mutable (can be changed):
1. Object - Collections of Properties
Objects store data in name-value pairs:
let person = {
firstName: "Alex",
age: 24,
isStudent: true,
hobbies: ["gaming", "music"]
};
// Accessing object properties
console.log(person.firstName); // "Alex"
console.log(person["age"]); // 24
2. Array - Ordered Collections
An array is a single variable storing multiple values in sequential order. Generally all the values are of same data type, but JavaScript allows mixed data types too (which can be used in complex situations).
let colors = ["red", "green", "blue"];
let mixedArray = [42, "hello", true, { name: "object" }]; // mixed data types
// Accessing array elements (zero-indexed)
console.log(colors[0]); // "red"
console.log(colors[2]); // "blue"
console.log(colors.length); // 3
Checking Data Types
You can check a variable's type using the typeof
operator:
let name = "Alex";
let age = 24;
let isOnline = true;
let person = { name: "Alex" };
let hobbies = ["coding", "gaming"];
console.log(typeof name); // "string"
console.log(typeof age); // "number"
console.log(typeof isOnline); // "boolean"
console.log(typeof person); // "object"
console.log(typeof hobbies); // "object" (arrays are objects in JavaScript)
Basic Operations in JavaScript
Now that we understand variables and data types, let's look at the operations we can perform on them.
Arithmetic Operators
These perform mathematical calculations:
let sum = 5 + 3; // 8 (Addition)
let difference = 10 - 4; // 6 (Subtraction)
let product = 7 * 6; // 42 (Multiplication)
let quotient = 20 / 5; // 4 (Division)
let remainder = 10 % 3; // 1 (Modulus (remainder))
let power = 2 ** 3; // 8 (2³) (Exponentiation)
let count = 5;
count++; // 6 (Increment)
let lives = 3;
lives--; // 2 (Decrement)
String Operations
let firstName = "Alex";
let lastName = "Johnson";
let fullName = firstName + " " + lastName; // "Alex Johnson" (String concatenation)
// Modern way using template literals
let greeting = `Hello, ${firstName}!`; // "Hello, Alex!"
// String methods
let text = "JavaScript is fun";
console.log(text.length); // 17
console.log(text.toUpperCase()); // "JAVASCRIPT IS FUN"
console.log(text.toLowerCase()); // "javascript is fun"
console.log(text.indexOf("fun")); // 14
console.log(text.includes("Java")); // true
Assignment Operators
These assign values to variables:
// Basic assignment
let x = 10;
// Compound Assignment operators
x += 5; // Same as x = x + 5
x -= 3; // Same as x = x - 3
x *= 2; // Same as x = x * 2
x /= 6; // Same as x = x / 6
Comparison Operators
These compare values and return a boolean:
// Equal to (checks value only)
console.log(5 == "5"); // true
// Strict equal to (checks value AND type)
console.log(5 === "5"); // false
// Not equal to
console.log(10 != 10); // false
// Strict not equal to
console.log(10 !== "10"); // true
// Greater than
console.log(10 > 5); // true
// Less than
console.log(5 < 10); // true
// Greater than or equal to
console.log(10 >= 10); // true
// Less than or equal to
console.log(5 <= 10); // true
Logical Operators
These combine or manipulate boolean values:
// AND (&&) - returns true if both conditions are true
console.log(true && true); // true
console.log(true && false); // false
// OR (||) - returns true if at least one condition is true
console.log(true || false); // true
console.log(false || false); // false
// NOT (!) - reverses the boolean value
console.log(!true); // false
console.log(!false); // true
Let's Put It All Together
Let's create a simple example that uses the concepts we've learned—variables, data types, and basic operations:
// Creating variables with different data types
const studentName = "Alex";
let mathScore = 85;
let englishScore = 92;
let isHonorStudent = false;
// Array to store all scores
let allScores = [mathScore, englishScore];
// Object to store student information
let student = {
name: studentName,
grade: "A",
subjects: 2
};
// Performing operations
let totalPoints = mathScore + englishScore; // Addition
let averageScore = totalPoints / 2; // Division
// Check if student qualifies for honor roll
if (averageScore >= 90) {
isHonorStudent = true;
student.grade = "A+";
}
// Display results using template literals
console.log(`Student: ${student.name}`);
console.log(`Total Points: ${totalPoints}`);
console.log(`Average Score: ${averageScore}`);
console.log(`Honor Student: ${isHonorStudent}`);
console.log(`Final Grade: ${student.grade}`);
Coming Up Next
In our next blog post, we'll dive into control flow: making decisions and repeating actions with conditionals and loops. These tools will allow us to create more dynamic and responsive JavaScript programs.
What part of JavaScript basics did you find most interesting? Do you have questions about any of these concepts? Let me know in the comments below!
Subscribe to my newsletter
Read articles from Shubhaw Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
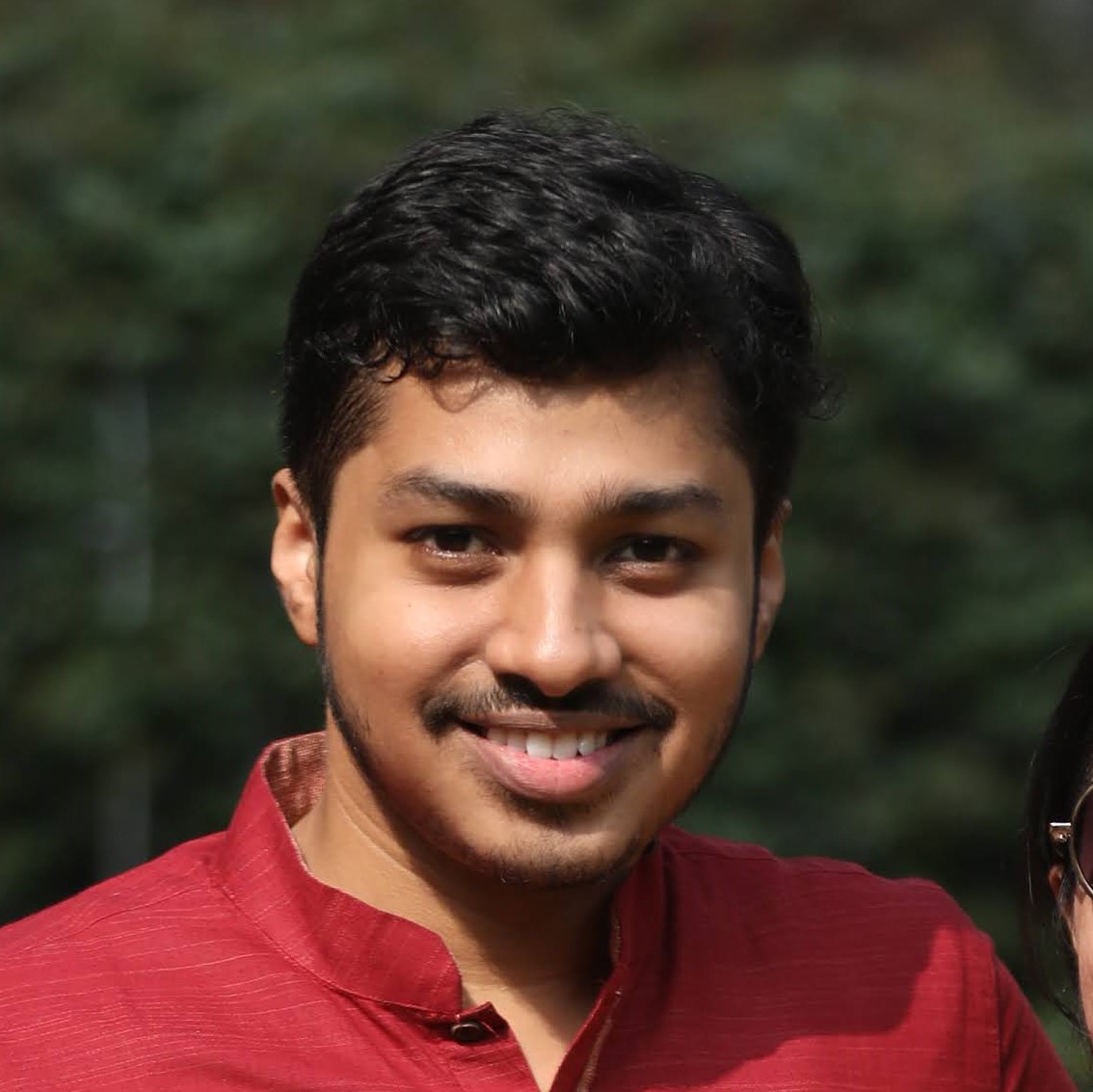
Shubhaw Kumar
Shubhaw Kumar
Software Engineer at Microsoft, India