Scope in JavaScript ๐
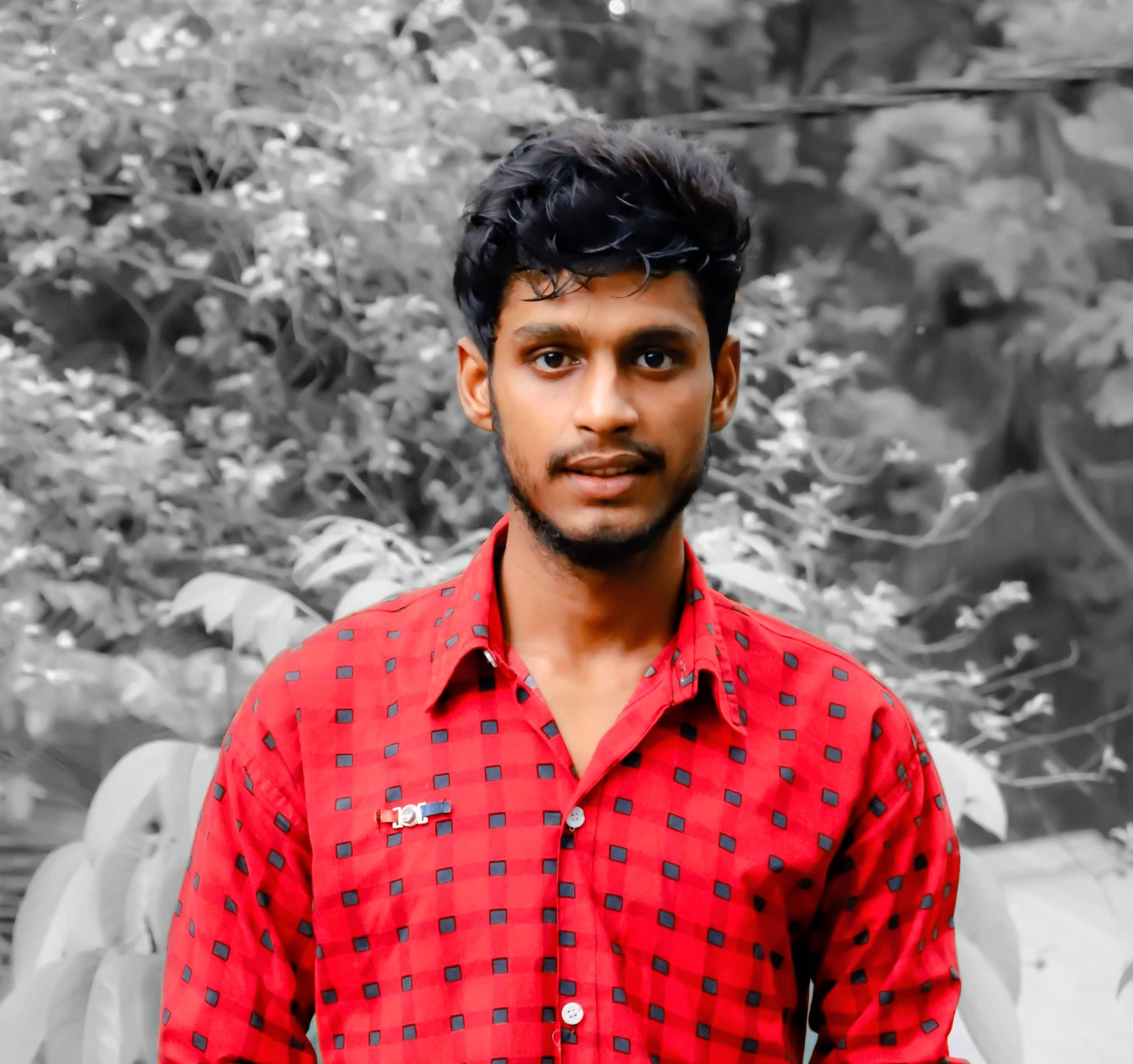
In JavaScript 3 types Scope is available.
- Global Scope.
- Block Scope.
- Functional Scope.
1. Global Scope :
In a browser, global scope is the window object. In Node.js, global object represents the global scope. To add something in global scope, you need to export it using export or module.
console.log(window);
//or
console.log(this)
Note : In global level this keyword refers to the window object.
this === window;
// output : true;
Global Variable Declare :
if we want to declare a variable in global lavel, we have to use var.
var myName = "Koushik";
we can access this variable like this:
console.log(window.myName);
//or
console.log(this.myName);
Note : If you use let and const, that variable is not global variable. for let and const variable are stored in a separate space of the memory section.
For Example :
We can not access let and const variable like this :
let myName ="Koushik Saha";
console.log(window.myName);
//or
console.log(this.myName);
2. Block Scope :
A variable when declared inside the if or switch conditions or inside for or while loops, are accessible within that particular condition or loop.
Block is used to combind multiple JavaScript statement into one group.
Every thing we write in curly bracket is block element, except var. You can say let and const are block element.
{ } ;
if () {
}
For Example :
We can not access let and const variable like this :
{
var a = 10;
let b =34;
const c = 45;
}
//we can not access b and c outside the block, try it in your system.
//but we can access a outside the block, because var is global element.
3. Functional Scope :
When a variable is declared inside a function, it is only accessible within that function and cannot be used outside that function.
Every thing inside a function are not accessible from outside, even if it is "var" declared.
You can say function is a proper block in JavaScript.
For example :
function hello(){
var a = 10;
let b = 23;
const c = 4;
}
// we can not access a, b, c from outside.
Subscribe to my newsletter
Read articles from Koushik Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
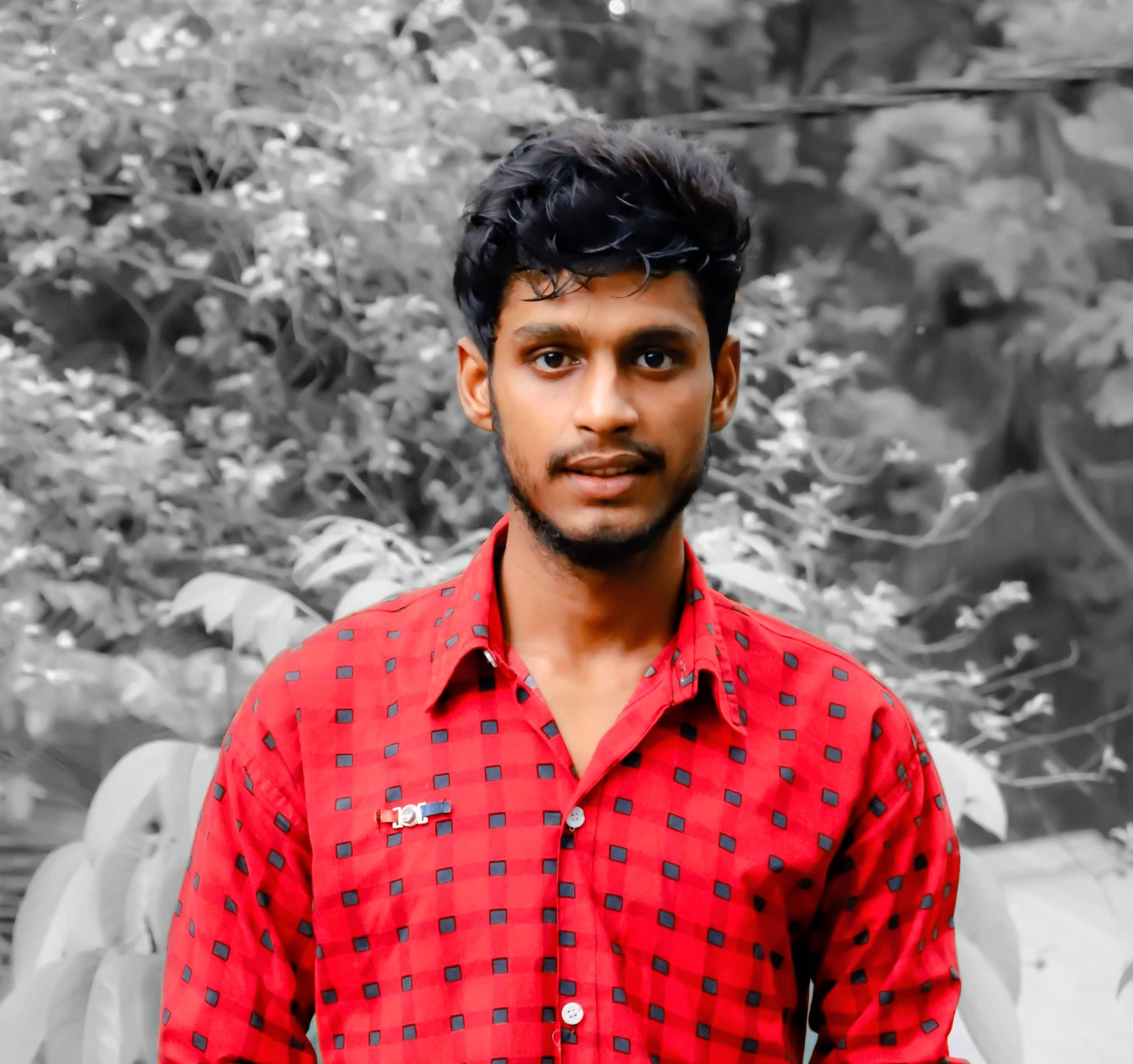
Koushik Saha
Koushik Saha
A Good Learner. And Web Developer.