✨ Closures In JS ✨
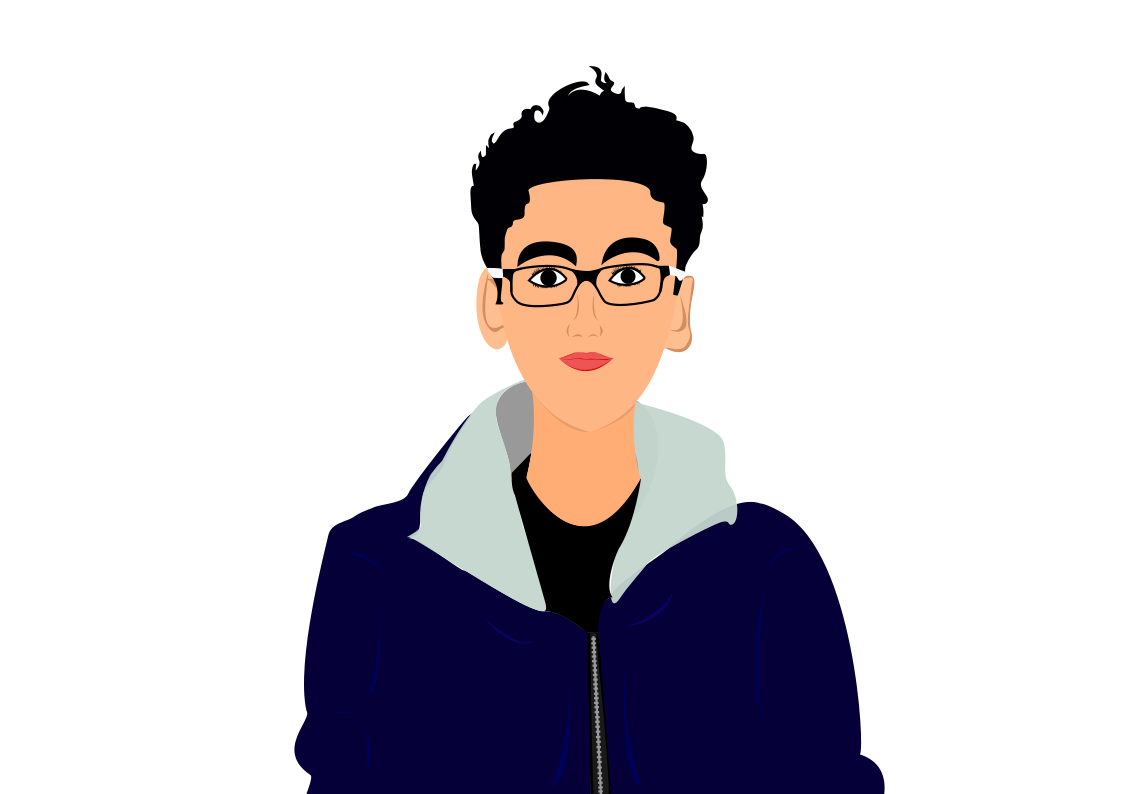
Table of contents
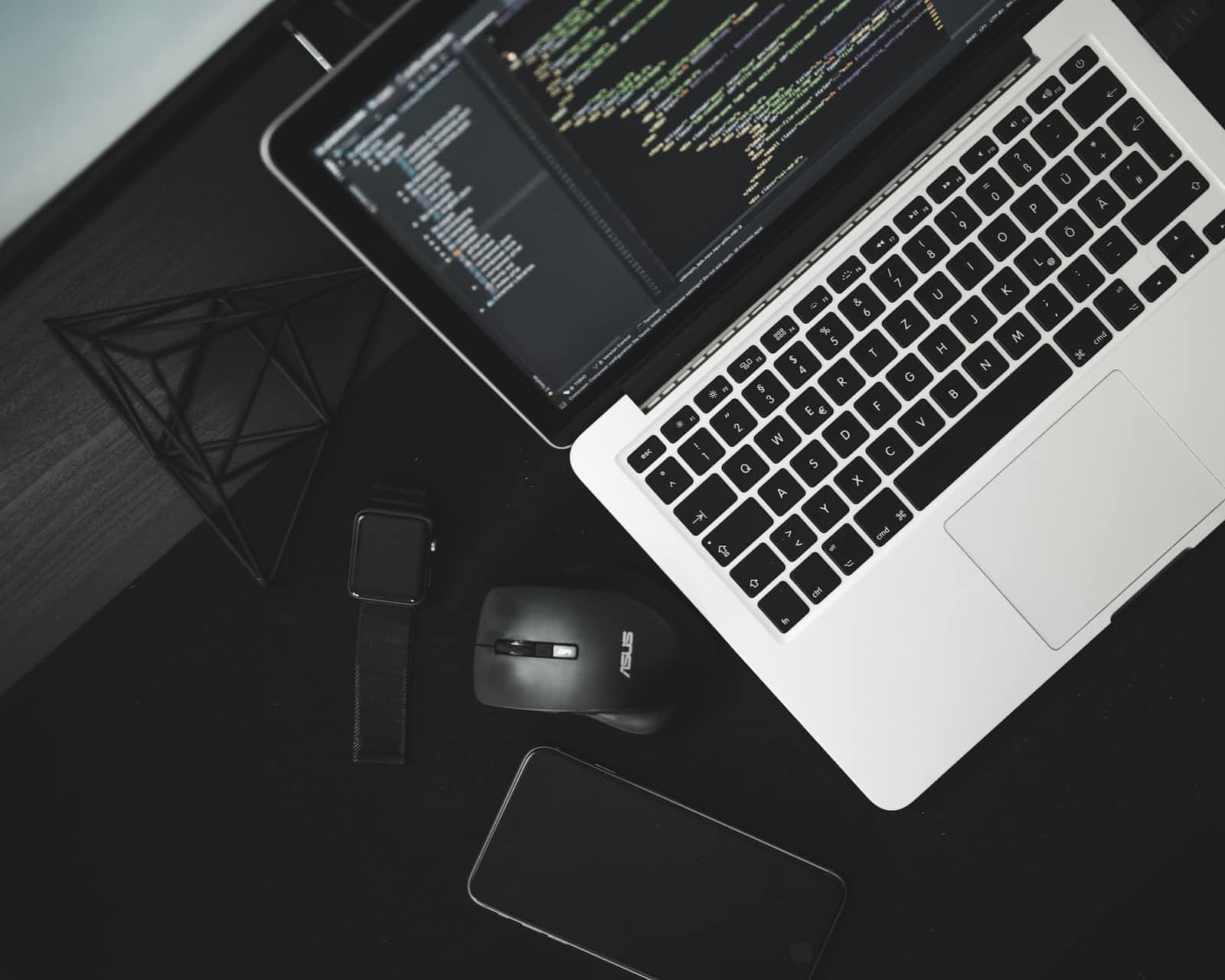
When I started learning this particular topic it messed with my head, although that happens most of the time while trying to learn something new🙈. I'm sure many of us have heard about the word Closure in JavaScript but know something and understanding it are two different tasks. This was me when I was trying to understand closures or pretty much anything.
Now that I have some grasp of the topic I'm here to showoff😂.
Introduction
Closures in JavaScript are nothing but functions that binds to their lexical environment that it was created in. I'm sure you might have heard this definition many times so, let's just break it down one by one.
- It is pretty evident from the definition that the function binds(stick together) to something. So, function is attaching itself to something called as the lexical environment it was created in.
- Lexical simple means "in order" or "in heirarchy". Whenever an execution context is created it also creates it’s lexical environment. Lexical environment is nothing but local memory and also the reference of the parents lexical environment.
So, now that it's out of the way. Let's concentrate on closures.
Consider the below example.
function x(){
var a=7;
function y(){
console.log(a);
}
y();
}
x(); // The output will be 7
So, the output of this particular snippet will be: 7
You might be wondering how because a is not present in the function y() and still giving the output as 7 it's weird isn't it? Nope, that's the beauty of JavaScript and this is where closures comes into picture.
var a was declared in line no.2 but is still available in the function y() because it's in the lexical environment of the y(). That means x was declared in the scope of y()'s parents or to simply put it the scope of function's parents(i.e. y()).
When the execution comes to line no. 4 it checks for variable a in the function y.
But a is nowhere to be found in in the function y. So, the program checks for any variable a in the lexical scope of y() or the enclosing scope where y() was created i.e from line no.2 till line no.6 and guess what it finds the variable it's looking for at line no.2 and it goes ahead and prints that particular value in the console.
Whenever a method is created they have a hidden property called as [[Environment]] this will have the reference of the lexical environment of the parent and that's how friends y() found variable a.
Mind === Blown 😂
So, it's basically closures can give us access to outer functions scope from inner functions.
Advantages of Closures
Since this is a pretty important concepts it has many advantages like currying, setTimeouts , Memoize , Functions like once, maintaining the state in async world , Iterators etc.
But one of the most important advantages is Data encapsulation or Data hiding. Let's understand it from the example given above. Suppose you have 100000 lines or more code after line no.8
function x(){
var a=7;
function y(){
console.log(a);
}
return y;
}
var answer = x();
// 100000 line or more of code
answer();//Output will still be 7
You might think when the execution reaches to the line no.100000 or more by that time the method x() will not be in the call stack but because of the thing that we are learning today the output it gives is still 7 and that is all down to Closures.
Conclusion
TL;DR
- Function that binds to the lexical environment that it was created in is called as Closure.
- Lexical environment is nothing but local memory and also the reference of the parents lexical environment.
- Closures helps in Data hiding or encapsulation among other advantages.
This was one of the most challenging and interesting topics I learned till now. Akshay Saini's video on closures Namaste JavaScript helped me a lot.
I hope you liked this explanation and it was worth your time . Feel free to comment and do reach out to me and let me know if you have any questions.
Subscribe to my newsletter
Read articles from Dhruv Vashist directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
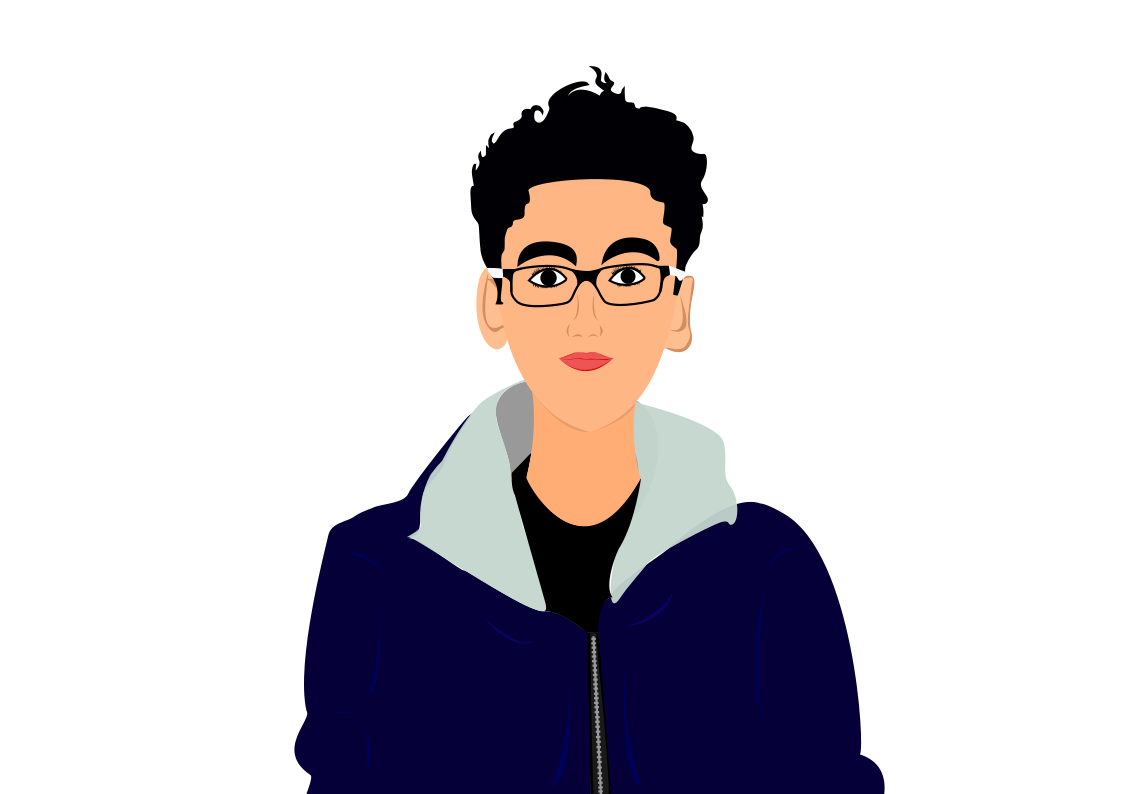
Dhruv Vashist
Dhruv Vashist
I'm a passionate software developer who thrives on the thrill of learning something new every single day. My journey is all about coding, debugging, and deploying with a sprinkle of creativity and innovation. I'm on a mission to enhance my skills and share my adventures in the tech world with you.