Tabs in React.js with Slider Animation

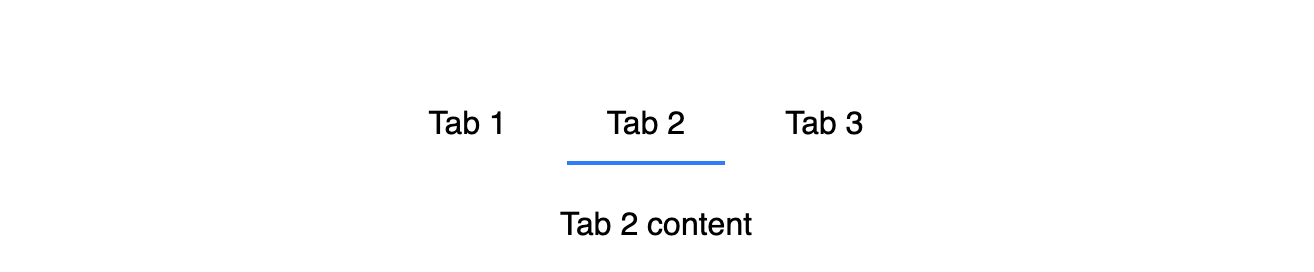
Tabs are a common UI element used in web applications to organize content and improve user experience. In this tutorial, we will show you how to create tabs in React.js with slider animation.
Prerequisites
Before we begin, make sure you have the following installed:
Node.js
React.js
Step 1: Set Up the Project
To get started, create a new React.js project using the create-react-app
command:
npx create-react-app react-tabs-slider
Once the project is created, navigate to the project directory and start the development server:
cd react-tabs-slider
npm start
Step 2: Create the Tabs Component
Next, let's create a new component for the tabs. In the src
directory, create a new file called Tabs.js
and add the following code:
import React, { useState } from "react";
import "./Tabs.css";
const tabs = [
{ id: 1, title: "Tab 1" },
{ id: 2, title: "Tab 2" },
{ id: 3, title: "Tab 3" }
];
export default function Tabs() {
const [activeTab, setActiveTab] = useState(tabs[0].id);
const handleTabClick = (tabId) => {
setActiveTab(tabId);
};
return (
<div className="tabs-container">
<div className="tabs">
{tabs.map((tab) => (
<div
key={tab.id}
className={`tab ${activeTab === tab.id ? "active" : ""}`}
onClick={() => handleTabClick(tab.id)}
>
{tab.title}
</div>
))}
<div className="tab-slider"></div>
</div>
<div className="tab-content">
{tabs.map((tab) => (
<div
key={tab.id}
className={`tab-pane ${activeTab === tab.id ? "active" : ""}`}
>
{tab.title} content
</div>
))}
</div>
</div>
);
}
In this code, we have a tabs
array that contains the tab data. We are using the useState
hook to keep track of the active tab.
The handleTabClick
function is called when a tab is clicked. It sets the active tab state to the clicked tab.
The render
function returns the tab components with the active tab style applied. The tab slider is a separate div
element that is positioned absolutely.
Step 3: Add Styles
Next, let's add some styles to the tabs component. In the src
directory, create a new file called Tabs.css
and add the following code:
.tabs-container {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
.tabs {
display: flex;
justify-content: center;
position: relative;
}
.tab {
padding: 10px 20px;
margin-right: 10px;
cursor: pointer;
border-bottom: 2px solid transparent;
transition: border-bottom-color 0.3s ease-in-out;
}
.tab.active {
border-bottom-color: `#007bff`;
}
.tab-slider {
position: absolute;
bottom: 0;
height: 2px;
background-color: `#007bff`;
transition: left 0.3s ease-in-out, width 0.3s ease-in-out;
}
.tab-content {
margin-top: 20px;
}
.tab-pane {
display: none;
}
.tab-pane.active {
display: block;
}
In this CSS file, we have styles for the tabs container, tabs, tab slider, and tab content. The tab
class styles the individual tabs, while the tab.active
class styles the active tab with a border bottom color. The tab-slider
class styles the tab slider with a background color and transition effect. The tab-pane
class styles the tab content with a display none property, while the tab-pane.active
class styles the active tab content with a display block property.
Step 4: Add Slider Animation
To add slider animation to the tabs, we need to update the handleTabClick
function to calculate the position and width of the tab slider. We can do this by adding a new state variable for the tab slider style and using the useRef
hook to get a reference to the tabs container element.
Here's the updated `Tabscomponent code:
import React, { useState, useRef, useEffect } from "react";
import "./Tabs.css";
const tabs = [
{ id: 1, title: "Tab 1" },
{ id: 2, title: "Tab 2" },
{ id: 3, title: "Tab 3" }
];
export default function Tabs() {
const [activeTab, setActiveTab] = useState(tabs[0].id);
const [tabStyle, setTabStyle] = useState({});
const tabRef = useRef(null);
useEffect(() => {
const activeTabElement = tabRef.current.querySelector(".active");
const newTabStyle = {
left: activeTabElement.offsetLeft,
width: activeTabElement.offsetWidth
};
setTabStyle(newTabStyle);
}, []);
const handleTabClick = (tabId) => {
const activeTabElement = tabRef.current.querySelector(".active");
const newActiveTabElement = tabRef.current.querySelector(`#tab-${tabId}`);
const newTabStyle = {
left: newActiveTabElement.offsetLeft,
width: newActiveTabElement.offsetWidth
};
setTabStyle(newTabStyle);
setActiveTab(tabId);
};
return (
<div className="tabs-container">
<div className="tabs" ref={tabRef}>
{tabs.map((tab) => (
<div
key={tab.id}
id={`tab-${tab.id}`}
className={`tab ${activeTab === tab.id ? "active" : ""}`}
onClick={() => handleTabClick(tab.id)}
>
{tab.title}
</div>
))}
<div className="tab-slider" style={tabStyle}></div>
</div>
<div className="tab-content">
{tabs.map((tab) => (
<div
key={tab.id}
className={`tab-pane ${activeTab === tab.id ? "active" : ""}`}
>
{tab.title} content
</div>
))}
</div>
</div>
);
}
In this updated code, we have added a new state variable called tabStyle
to keep track of the style of the tab slider. We are using the useRef
hook to get a reference to the tabs container element.
The useEffect
hook is used to set the initial style of the tab slider when the component mounts.
The handleTabClick
function is updated to calculate the new style for the tab slider based on the position and width of the new active tab. It then sets the new style and active tab state.
Step 5: Update Styles
Finally, let's update the styles to include the new tab slider style. In the Tabs.css
file, add the following styles for the tab slider:
.tab-slider {
position: absolute;
bottom: 0;
height: 2px;
background-color: `#007bff`;
transition: left 0.3s ease-in-out, width 0.3s ease-in-out;
left: 0;
width: 0;
}
In this CSS code, we have added the left
and width
properties to the tab-slider
class to set the initial position and width of the tab slider.
Conclusion
In this tutorial, we have shown you how to create tabs in React.js with slider animation. We started by creating a new component for the tabs and adding styles for the tabs and tab content. We then updated the component to include slider animation by calculating the position and width of the tab slider. Finally, we updated the styles to include the new tab slider style.
By following these steps, you can create tabs with slider animation in your React.js applications and improve the user experience of your web applications. Here's the codesandbox link which contains the code as explained in the blog.
Subscribe to my newsletter
Read articles from Evleensingh Thakral directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
