How To Test GraphQL API Endpoints with Platformatic Using Hoppscotch

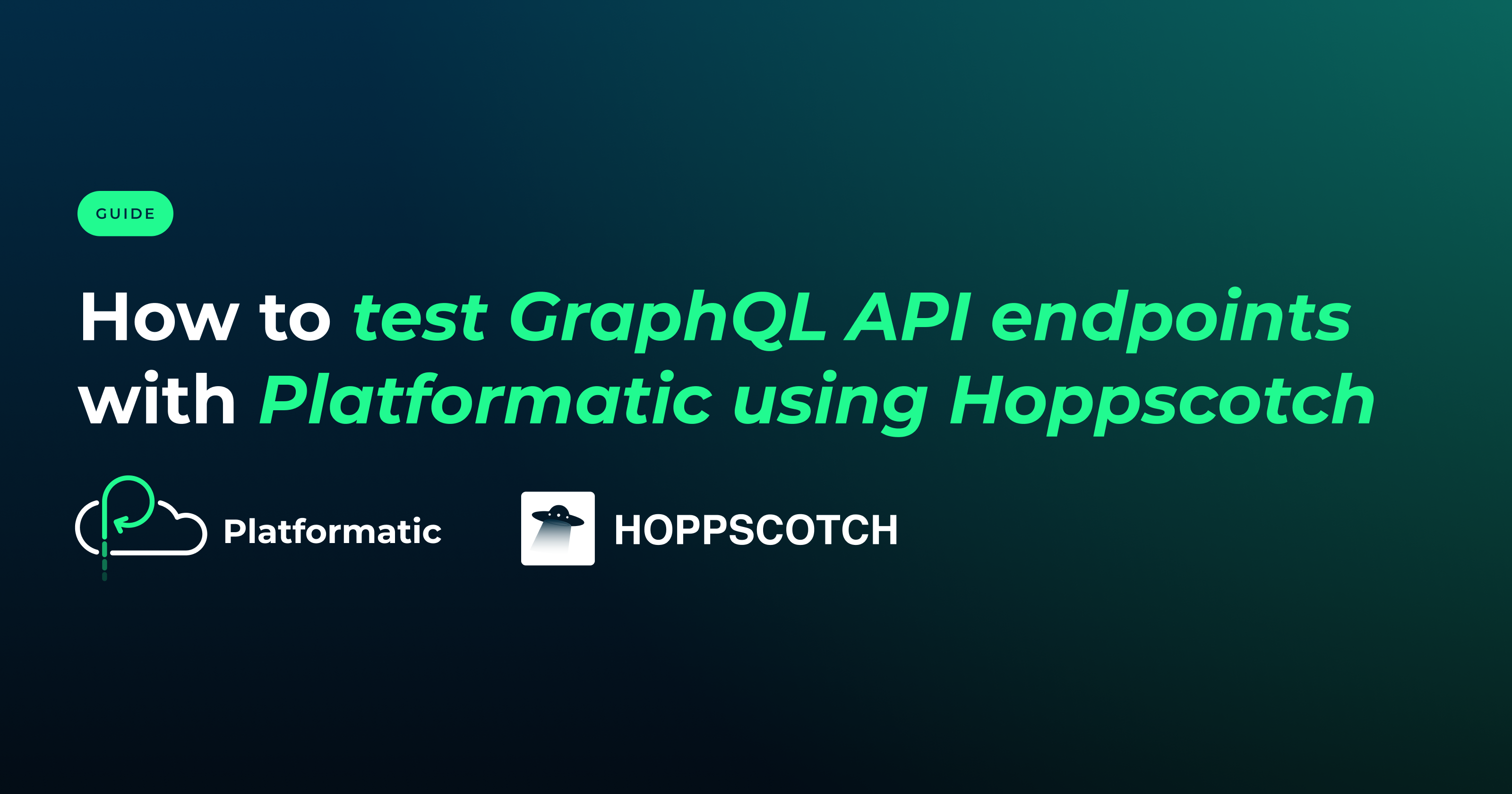
Testing is an important aspect of any development process in modern-day software development. In backend development, testing endpoints is just as important as writing out the code needed to build them, ensuring that each endpoint validates requests and sends appropriate success or error responses.
Platformatic DB can help you simplify building REST API and GraphQL APIs, enabling you to automatically map them from the tables in our database.
Hoppscotch, on the other hand, is an API development web app that simplifies the process of testing, documenting, and managing APIs. It provides an intuitive UI to send HTTP requests, view responses, and analyze API behaviour. Hoppscotch simplifies testing REST APIs and GraphQL APIs, and works with real-time applications like Socket.io and WebSockets.
In this tutorial, we will build a simple GraphQL API for a notes app API. We will also learn how to write queries and mutations to test our API using Hoppscotch.
Prerequisites
To follow this tutorial, you must have a basic knowledge of Javascript and SQL databases.
You also need to have the following installed on your PC
Node.js >= v18.8.0 or >= v19.0.0
npm v7 or later
A code editor (we will be using VS Code)
Setting Up Platformatic DB
To set up Platformatic DB using the automatic CLI method:
1. Create a folder named graphql-api and open the folder in the code editor
2. Open your terminal in your code editor and input the code below to start the Platformatic Creator wizard
npm create platformatic@latest
3. After running the command in the terminal, you will be prompted with an interactive command-line tool. For this tutorial, select these options:
Hello, <Your Name>, welcome to Platformatic 0.29.0!
Let's start by creating a new project.
? Which kind of project do you want to create? => DB
? Where would you like to create your project? => .
? What database do you want to use? => SQLite
? Do you want to use the connection string "sqlite://./db.sqlite"? => y
? Do you want to create default migrations? => yes
? Do you want to create a plugin? => yes
? Do you want to use TypeScript? => no
? What port do you want to use? => 3042
? Do you want to run npm install? => yes
? Do you want to apply migrations? => yes
? Do you want to generate types? => yes
? Do you want to create the github action to deploy this application to Platformatic Cloud dynamic workspace? => no
? Do you want to create the github action to deploy this application to Platformatic Cloud static workspace? => no
All done! Please open the project directory and check the README.
Integrating our Schema to Platformatic DB
Having set up our Platformatic DB, we will now proceed to integrate our note app schema into Platformatic DB and build our endpoints.
To integrate the schemas into our Platformatic DB:
1. Paste the schema below in the 001.db.sql file in the migrations folder
CREATE TABLE users (
id INTEGER PRIMARY KEY,
username VARCHAR(255) NOT NULL,
created_at DATETIME DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE notes (
id INTEGER PRIMARY KEY,
user_id INTEGER,
title VARCHAR(255) NOT NULL,
content TEXT,
created_at DATETIME DEFAULT CURRENT_TIMESTAMP,
updated_at DATETIME DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES users(id) ON DELETE CASCADE
);
2. Run the command below in your terminal
npx plt db migrations apply -t 0
The above command deletes the existing db.sqlite file.
3. Run the command below to apply your new migrations. This command also creates a new db.sqlite file, regenerates the global.d.ts file and automatically generates your schema and Entities in the global.d.ts file.
npm run migrate
4. You should have the output below in your terminal
5. Run the command below to start your server on port 3042.
npm start
6 . You should have the output below in your terminal:
To view the GraphQL schema generated by Platformatic DB for our app, you can run the command below in another terminal:
npx platformatic db schema graphql
Testing The Endpoints Using Hoppscotch
Now let's test out our GraphQL API endpoints using Hoppscotch.
The first step is to allow Cross-Origin Resource Sharing from the Hoppscotch web app to our server.
Copy the variable below and paste it into the .env file:
PLT_SERVER_CORS_ORIGIN=https://hoppscotch.io/
You’ll also need to add a CORS configuration to the API configuration file; platformatic.db.json file.
{
"server": {
"logger": {
"level": "{PLT_SERVER_LOGGER_LEVEL}"
},
"hostname": "{PLT_SERVER_HOSTNAME}",
"port": "{PORT}",
"cors": {
"origin": "{PLT_SERVER_CORS_ORIGIN}"
}
},
...
}
Now, we are ready to write queries and mutations to test our graphql endpoints.
Go to the Hoppscotch app.
Signup or Login to your Hoppscotch account. You can do this by using either your Github, Google, or Microsoft accounts or email addresses.
Paste http://localhost:3042/graphql in the tab for the URL.
On the left sidebar, click the GraphQL tab to change the testing environment from REST API to GraphQL API.
Create
Now it’s time to write a query to create a new user:
mutation{
saveUser(input: {
username: "john_doe",
}){id, username}
This query creates a new User and returns the user ID and username as a response.
Paste the above query into the labelled field and click on Run. You should have the response below:
To create a new note, paste the query below into the query field:
mutation{
saveNote(input: {
userId: 1,
title: "First Note title",
content: "First Note content"
}){id, title, content, user{id, username}}
}
You can go on to create more users and notes.
Read
To Fetch users, paste the query below into the query field:
query{
users{
id, username
}
}
You can fetch notes by using the query below:
query{
notes{
id, title, content, user{id, username}
}
}
You can get notes by ID:
query{
getNoteById(id:1){
title, content, user{id, username}
}
}
Delete
You can delete a note:
mutation{
deleteNotes(where: {id: {eq: 2}}){
id
}
}
View Documentation and Schema
You can also view the schema and documentation the Platformatic DB generates in Hoppscotch.
- To view the generated documentation, click the book icon on the left sidebar and then the Connect button.
- To view the generated schemas, click on the cube icon and then the Connect button (if you have not connected to the app).
Wrapping Up
Throughout the course of this tutorial, we have seen how using Platformatic DB makes building GraphQL endpoints extremely fast. We also saw how Hoppscotch came in handy, making the process of testing our API seamless.
There are numerous GraphQL APIs you can build using the Platformatic DB. You can use Hoppscotch to test both REST API and GraphQL API. Hence, you can go on to build a REST API using Platformatic DB and test it using Hoppscotch.
If you have any questions about this tutorial, you can always reach out to us on Twitter.
Subscribe to my newsletter
Read articles from Matteo Collina directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
