Demystifying Deletion of Nodes from a Binary Search Tree (BST)
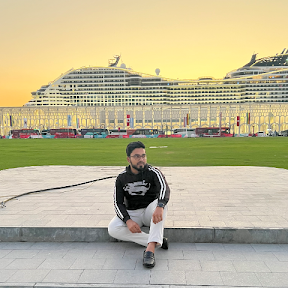
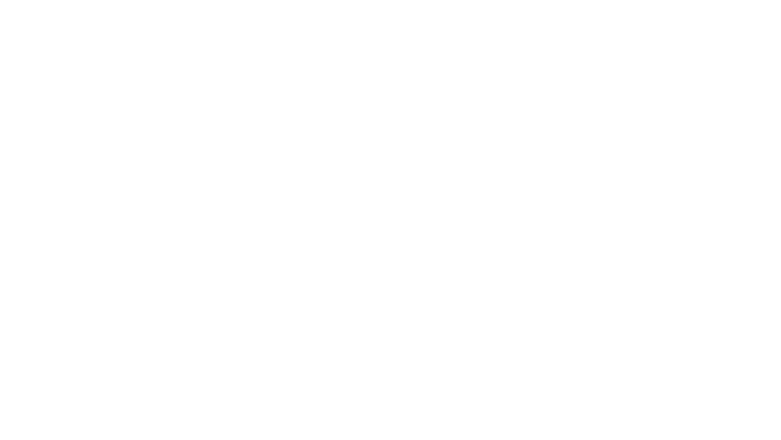
Introduction :
Binary Search Trees (BSTs) are foundational data structures in the realm of computer science, celebrated for their efficient search, insertion, and deletion operations. Their elegant design leverages a hierarchical arrangement of nodes, where each node holds a key value, and every node's left child contains a smaller key while the right child holds a larger key. This fundamental property empowers BSTs to facilitate rapid searches and ordered traversals.
While the insertion of nodes into a BST establishes its initial structure, the deletion of nodes is equally vital to maintaining the integrity and balance of the tree. Deletion operations are essential for adapting the tree to changing data dynamics, removing obsolete elements, and preventing the tree from degenerating into a less efficient structure.
In this article, we will learn the intricacies of deleting nodes from a Binary Search Tree. We will also delve into the precise steps required to identify the node to be deleted and explore the nuanced handling of different cases, ranging from nodes with zero children to nodes with one or two children.
Understanding Binary Search Trees:
A Binary Search Tree is a hierarchical data structure where each node has at most two children, commonly referred to as the left child and the right child. The nodes are organized in a manner that follows a crucial property: the key value of the node's left child is smaller than the key value of the node itself, while the key value of the right child is larger. This property serves as the guiding principle for searching and organizing elements within the tree.
The heart of a BST's efficiency lies in its adherence to the key property: the left child of a node contains a key that is smaller than the node's key, and the right child contains a larger key. This arrangement creates a natural ordering within the tree, akin to the sorted nature of an array. Consequently, as we traverse down the tree from the root to a specific node, we can rapidly narrow down our search range based on the comparison of key values, leading to efficient search operations with a time complexity of O(log n), where n is the number of nodes.
Let's visualize a simple example to grasp the structure of a Binary Search Tree. Consider the following series of integers: 70, 50, 90, 30, 60, 80, 100, 20, and 40. We will construct a BST using these values:
In this example, the root node contains the key value 70, with its left child containing 50 and its right child containing 90. The subsequent levels follow the property of smaller keys to the left and larger keys to the right. For instance, the node with key 30 has children 20 and 40, while the node with key 90 has children 80 and 100. This hierarchical arrangement allows for efficient searches and ordered traversals, showcasing the power of Binary Search Trees in organizing data.
Importance of Deletion:
Deletion plays a pivotal role in Binary Search Trees (BSTs), contributing to balance, memory management, and precise searches. Removing nodes allows the tree to adapt dynamically to changing data, preventing it from skewing and retaining optimal search efficiency. However, deletion requires cautious execution to uphold the BST's key property: the left-child-right-child relationship. Mishandling could lead to tree degradation. Thus, mastering deletion techniques is essential to sustain the reliability and effectiveness of BSTs in various applications.
Node Deletion Process in a Binary Search Tree:
The process of deleting a node from a Binary Search Tree (BST) is a delicate operation that requires meticulous consideration to preserve the tree's balance and ordering properties. The goal is to seamlessly remove a node while ensuring that the tree remains a valid BST.
The process can be broken down into several key steps:
Identifying the Node to Delete:
- The first step is to locate the node that needs to be deleted. This is achieved by traversing the tree based on the value of the node to be removed, similar to the process of searching.
Handling Different Cases:
a. Node with Zero Children:
If the node to be deleted has no children (leaf node), the operation is relatively straightforward. The parent of the node simply has its reference to the target node set to null, effectively removing the node from the tree.
This process takes constant time, denoted as O(1)
b. Node with One Child:
When the target node has only one child, the parent of the target node is linked directly to its sole child. This step effectively bypasses the node to be deleted, ensuring a seamless transition in the tree's structure.
This process takes constant time, denoted as O(1)
c. Node with Two Children:
Deleting a node with two children is more intricate. The in-order successor or predecessor of the node must be found. The in-order successor is the smallest node in the right subtree of the target node, while the in-order predecessor is the largest node in the left subtree.
The key value of the target node is then replaced with the value of the in-order successor/predecessor, effectively maintaining the ordering property.
Finally, the in-order successor/predecessor node, which now has a duplicate key value, is recursively deleted using the same process.
This process takes O(log n), where n is the number of nodes time complexity
Considerations and Challenges in Node Deletion:
Node deletion in Binary Search Trees demands careful handling due to multifaceted considerations. Preserving balance to sustain efficient search operations, managing memory allocation, and navigating duplicate key values require strategic choices. Implementing rebalancing techniques such as AVL or Red-Black trees may counterbalance tree skewing post-deletion. Special cases like root deletion necessitate meticulous restructuring. Balancing efficiency while upholding ordering principles adds complexity. Ensuring correct implementation through thorough testing validates the deletion process. Negotiating these intricacies ensures effective node deletion, contributing to the overall integrity and utility of Binary Search Trees
If you're interested in learning more about how to delete nodes from a Binary Search Tree, you can check out the deletion method in this GitHub repository. This code example shows you how to remove nodes from a BST while keeping everything organized and working correctly.
Subscribe to my newsletter
Read articles from Mohammed Ruman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
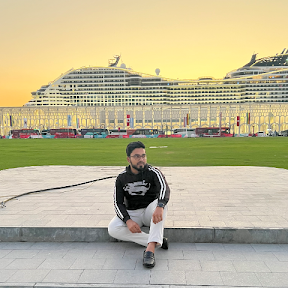
Mohammed Ruman
Mohammed Ruman
I am a back-end developer proficient in Java and Spring-boot framework. I also have working knowledge on front end technologies. I love to share my knowledge and to learn in public