Subjugating Chaos: Exception Handling in Python with Try-Except Blocks and Custom Exceptions

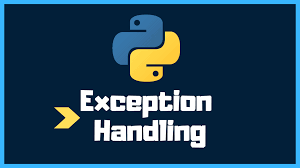
Errors and exceptions are inevitable companions in the world of programming. Python equips you with robust mechanisms to handle these disruptions gracefully, preventing program crashes and ensuring a smooth user experience. This article delves into exception handling using try-except blocks and custom exceptions, empowering you to write resilient and user-friendly Python code.
Understanding Exceptions: When Things Go Wrong
Exceptions are events that disrupt the normal flow of your program's execution. They can arise due to various reasons, such as:
User input errors: The user enters invalid data (e.g., trying to convert a string to a number).
File operations: Issues like trying to open a non-existent file or attempting to write to a read-only file.
Network errors: Network connectivity problems during communication attempts.
The Try-Except Block: A Safety Net for Your Code
The try-except
block is the cornerstone of exception handling in Python. It allows you to define a code block (the try
clause) that might raise an exception and specify how to handle that exception (the except
clause). Here's the basic structure:
try:
# Code that might raise an exception
except ExceptionType:
# Code to handle the exception
Catching Specific Exceptions:
You can specify the exact type of exception you want to catch within the except
clause:
try:
# Code that might raise a ValueError
except ValueError:
print("Error: Invalid input. Please enter a number.")
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
The else
Clause: Code for Normal Execution
The else
clause is optional and allows you to specify code that executes only if no exception occurs within the try
block:
try:
result = divide(num1, num2)
except ZeroDivisionError:
print("Error: Division by zero.")
else:
print("Result:", result)
Introducing Custom Exceptions: Tailored Error Handling
While Python provides built-in exceptions, you can create your own custom exceptions to handle specific errors in your application. Here's how:
class InvalidAgeException(Exception):
def __init__(self, message):
self.message = message
def validate_age(age):
if age < 18:
raise InvalidAgeException("Age must be 18 or older.")
try:
validate_age(15)
except InvalidAgeException as e:
print("Error:", e.message)
In this example, InvalidAgeException
is a custom exception class that inherits from the Exception
class. It allows you to raise a more specific error message when encountering an invalid age.
Benefits of Exception Handling:
Improved Program Stability: Exception handling prevents program crashes and allows for graceful recovery from errors.
Enhanced User Experience: By providing informative error messages, you guide users on how to rectify the issue.
Modular and Maintainable Code: Separating error handling concerns improves code readability and maintainability.
Remember:
Use specific exception types in
except
clauses whenever possible for more precise error handling.Don't abuse bare
except
clauses (catching all exceptions withexcept:
) as it can mask underlying issues.Custom exceptions add clarity and context to your error handling.
Therefore by mastering exception handling with try-except blocks and custom exceptions, you write robust and user-friendly Python code. Embrace these techniques to create resilient applications that can handle unexpected situations gracefully, ensuring a smooth experience for both you and your users.
Subscribe to my newsletter
Read articles from David Oshare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Oshare
David Oshare
I am a Python developer with 2 years of experience. I love building things with code and sharing that knowledge with others. Looking to collaborate and keep learning!