HTTP Fundamentals: How to Easily Make HTTP Calls with Platformatic

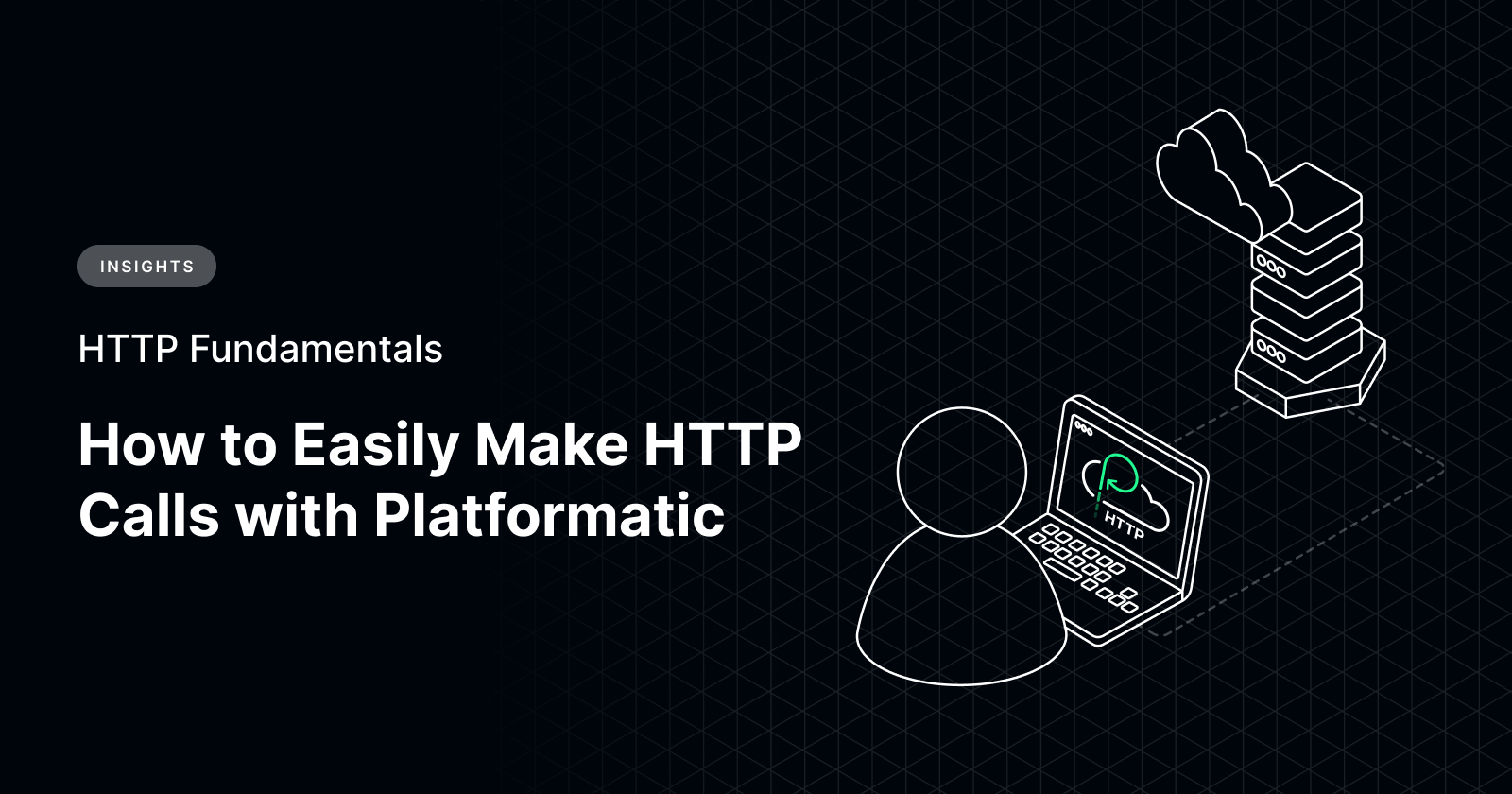
An HTTP call, also known as an HTTP request, is a mechanism applications use to communicate with servers over the Hypertext Transfer Protocol (HTTP). It involves sending a request from a client to a server and receiving a response in return. These calls are fundamental for various web-based operations, such as fetching data from an API, submitting form data, or accessing resources on a server.
Performing HTTP calls easily is crucial for application development efficiency, productivity, scalability, integration, and debugging. Simplifying the process saves time and effort, reduces cognitive load, supports scalability, enables smooth integration with third-party services, and aids in identifying and resolving issues quickly.
In this blog, we will explore using Platformatic to make HTTP calls, simply.
Getting started with Platformatic
Platformatic offers open-source tools designed to simplify the development journey for Node.js developers. We aim to enable developers to concentrate on core functionalities rather than getting caught up in time-consuming infrastructure tasks.
Developers often invest significant time in backend development, tackling tasks like establishing database connections, creating documentation, setting up local development environments, managing logs, implementing updates, configuring boilerplates, monitoring performance, and integrating API clients.
Platformatic simplifies this process by automating repetitive tasks, allowing developers to focus on building valuable application features rather than dealing with mundane infrastructure concerns.
Platformatic Client Demo
The Platformatic Client simplifies API creation for your application, seamlessly supporting Open API or GraphQL. It automatically generates type-safe clients for your API, offering clear interfaces and autocomplete support in your code editor.
You can create two types of libraries: one for local servers and another for browser-based applications, each tailored to its respective environment.
To use Platformatic Client, start by running the command below to create a Platformatic application.
npm create platformatic@latest
Then, follow the steps below:
Hello <your name>, welcome to Platformatic 1.37.1!
? What kind of project do you want to create? Application
? Where would you like to create your project? pl
✔ Installing @platformatic/runtime...
? Which kind of project do you want to create? DB
? What is the name of the service? db
? What is the connection string? sqlite://./db.sqlite
? Do you want to create default migrations? yes
? Do you want to create another service? yes
? Which kind of project do you want to create? Service
? What is the name of the service? service
? Do you want to create another service? yes
? Which kind of project do you want to create? Composer
? What is the name of the service? composer
? Do you want to create another service? no
? Which service should be exposed? composer
? Do you want to use TypeScript? no
? What port do you want to use? 3042
Your terminal should look similar to this:
Navigate into the pl folder from the terminal and start the application by running the command below in the terminal.
cd pl
npm start
The output displayed in the image below is logged to the terminal.
You can now navigate to http://localhost:3042/documentation to view your application’s documentation.
Let’s create a movie using the documentation UI:
Click on movies on the left sidebar and the movies toggle button.
Then click on the Create the movies endpoint.
On the main page, click the Test Endpoint button
Then, input the movie name as displayed in the image below.
Generate a client
To generate a Platformatic client, first, open a new terminal, then create a folder and navigate to that folder by running the commands below:
mkdir platformatic-client
cd platformatic client
Initialize a new Node project:
node init -y
As shown below, add a script tag in the package.json file to generate the front-end client.
"scripts": {
"start": "platformatic client --frontend http://localhost:3042/documentation/json",
},
Now run the command below to generate the client for the Platformatic db:
npm start
Your terminal output should look like this:
When you check the platformatic-client folder, you will see a new folder named api that has been created. The folder contains api-types.d.ts
, api.mjs, and api.openapi.json files.
Now, let’s write a client file to test the API.
First, open the package.json file and add a type key set to module.
Then, create a new file called client.mjs
and paste the code below into it:
import {dbGetMovies, setBaseUrl} from "./api/api.mjs";
setBaseUrl("http://localhost:3042");
const res = await dbGetMovies({});
console.log(res);
Now run the following command in the terminal:
node client.mjs
You should get the first movie created earlier logged to the console, as shown below:
Alternatively, we can write the code in the client.mjs file like this:
import build from "./api/api.mjs";
const api = build("http://localhost:3042");
const res = await api.dbGetMovies({});
console.log(res);
This produces the same result as the initial approach.
Provide a New Endpoint
We can create a new endpoint in the pl folder by calling one Platformatic service within another. For instance, we can create a database (DB) from the services to make all the titles uppercase.
To do this, open a new terminal, then navigate to the service folder within the pl folder.
cd pl
cd services
cd service
Within the service folder, open the package.json and include a new script tag as shown below:
"scripts": {
"prepare": "platformatic client --runtime db --name movies",
}
Run the command below in the terminal:
npm run prepare
Your terminal should display the following:
Within the service folder, a new folder named movies has been created.
This folder contains a package.json file, a movies.openapi.json file, and a movies.d.ts file, which provides the module's types.
The full path of the Open API is also added to the platformatic.json file within the service folder. This helps the service run independently– it will not require the availability of other folders.
Open the routes folder and then the root.js file within the service folder. Add this code to the file's second line:
/// <reference path="../movies/movies.d.ts" />
Then, add a new endpoint to the root file by pasting the code below into the file:
fastify.get("/titles", async (request, reply) => {
const movies = await request.movies.getMovies({});
return movies.map((movie) => movie.title.toUpperCase());
});
When you restart the server, you will notice a new endpoint, ‘/service/titles’, under the default section.
Testing the endpoint returns the movie list in capital letters, as shown in the image below.
It’s important to note that the client is tied to the request, not the server. This makes it easy to move certain security headers so the Platformatic client can be configured to convert the headers automatically. Once the security headers are defined, the Platformatic client processes them in a GET request.
In the movies type, you can see a ConfigureMovies interface, which provides a getHeaders function which extracts the headers from the request.
It is possible to have the same client for both clients we generated earlier. They will both be undici-based. To do so, create a new folder named client1 outside the pl folder. Within this folder, initialize a new node project. Edit the package.json file to include the script below:
"scripts": {
"prepare": "platformatic client http://localhost:3042/documentation/json composer",
}
Run the command below in the terminal:
npm run prepare
The image below shows the output that is logged to the terminal:
Within the client1 folder, a new folder named client has been generated. Within this client folder is a package.json file, client.cjs, client.d.ts and client.openapi.json files.
The types within the client.d.ts files are important. However, the generateClientPlugin in the client.cjs file is not always necessary unless the dev needs a client plugin for what they are building, which is project-specific. The actual client is assembled from the path in the OpenAPI.
A Platformatic Client can also function without the generator. This leverages the buildOpenAPIClient function, which is based on HTTP requests.
Let’s try this out.
Start by creating a new folder named client2 outside of the pl folder. Navigate to the folder in the terminal. Run the command below to install the @platformatic/client package, which will be used to build the client.
npm i @platformatic/client
Create a file named myClient.mjs within the client2 folder and paste the code below into the file:
import { buildOpenAPIClient } from "@platformatic/client";
const client = await buildOpenAPIClient({
url: `http://localhost:3042/documentation/json`,
});
const res = await client.dbGetMovies({});
console.log(res);
Run the command below in the terminal to execute the myClient.mjs file.
node mClient.mjs
The output logged to the terminal is shown in the image below. It works exactly as before.
Wrapping Up
Ultimately, many developers spend too much time building clients for their microservice system. This time could be better used to focus on the application's key, value-adding, and differentiating features. Platformatic Client offers developers a way to save valuable time and re-prioritize accordingly.
Want a deep dive into HTTP Clients? Watch the YouTube tutorial here.
Subscribe to my newsletter
Read articles from Matteo Collina directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
