Understanding Java HashSet for DSA
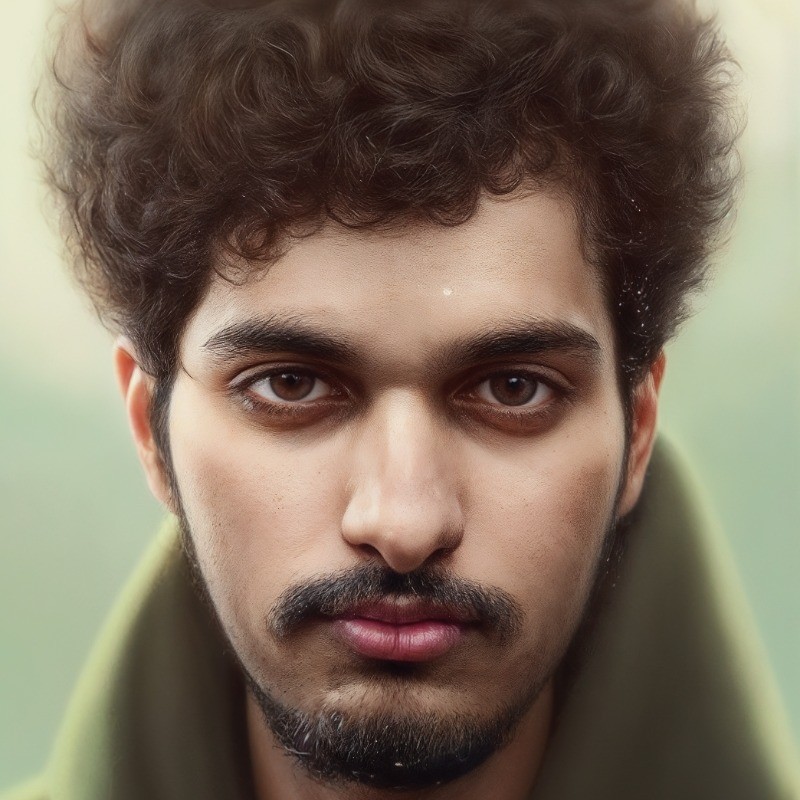
A HashSet
in Java is a part of the Java Collections Framework and provides a way to store unique elements. It is part of the java.util
package and implements the Set
interface. The HashSet
class does not allow duplicate elements, allows at most one null
element, and does not guarantee the order of its elements over time.
Key Characteristics
Unique Elements: Stores unique elements; duplicate elements are not allowed.
At Most One Null Element: Allows at most one
null
element.Unordered: Does not guarantee any specific order of the elements.
Not Synchronized: The
HashSet
itself is not synchronized. For concurrent access, useCopyOnWriteArraySet
or synchronize it externally.
Constructor
Default Constructor: This creates an empty
HashSet
with the default initial capacity (16) and the default load factor (0.75).HashSet<String> set = new HashSet<>();
Constructor with Initial Capacity: This creates an empty
HashSet
with the specified initial capacity and the default load factor (0.75).HashSet<String> set = new HashSet<>(20);
Constructor with Initial Capacity and Load Factor: This creates an empty
HashSet
with the specified initial capacity and load factor.HashSet<String> set = new HashSet<>(20, 0.8f);
Constructor with Collection: This creates a new
HashSet
with the same elements as the specifiedCollection
.List<String> list = Arrays.asList("Apple", "Banana", "Cherry"); HashSet<String> set = new HashSet<>(list);
Common Methods
add(E e): Adds the specified element to this set if it is not already present.
hashSet.add("element1");
contains(Object o): Returns
true
if this set contains the specified element.boolean contains = hashSet.contains("element1");
remove(Object o): Removes the specified element from this set if it is present.
hashSet.remove("element1");
size(): Returns the number of elements in this set.
int size = hashSet.size();
isEmpty(): Returns
true
if this set contains no elements.boolean empty = hashSet.isEmpty();
clear(): Removes all of the elements from this set.
hashSet.clear();
Iteration
HashSet
can be iterated using various methods such as for-each loop, iterators, and streams.
for (String item : hashSet) {
System.out.println(item);
}
Iterator<String> iterator = hashSet.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
hashSet.forEach(System.out::println);
Performance Considerations
Time Complexity: The average time complexity for
add
,remove
, andcontains
operations is O(1). However, in the worst case (due to hash collisions), it can be O(n).Load Factor: The load factor determines when the capacity of the
HashSet
is increased. A higher load factor means less space overhead but potentially slower access times.Initial Capacity: The initial capacity of the
HashSet
determines the number of buckets when theHashSet
is created. Choosing an appropriate initial capacity can reduce the need for resizing.
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
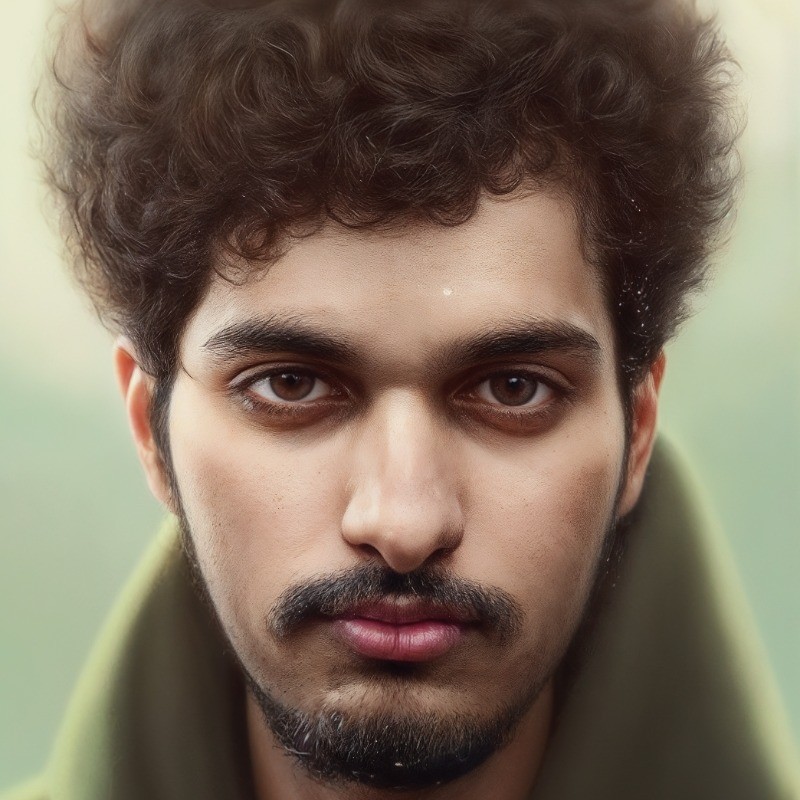
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?