A Beginner's Guide to Binary Trees
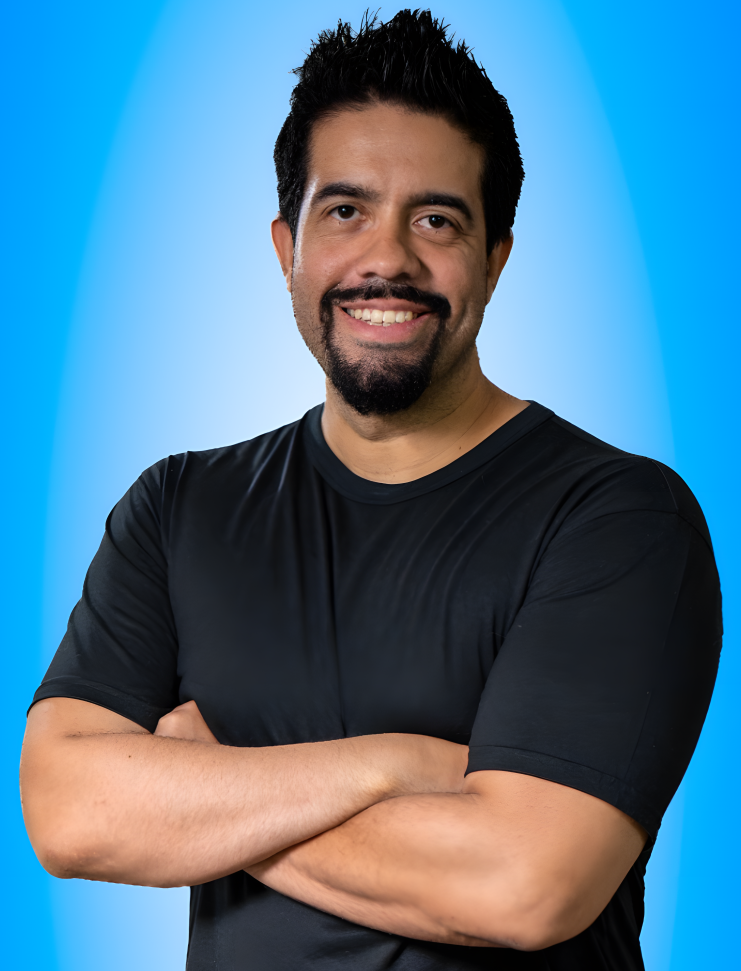
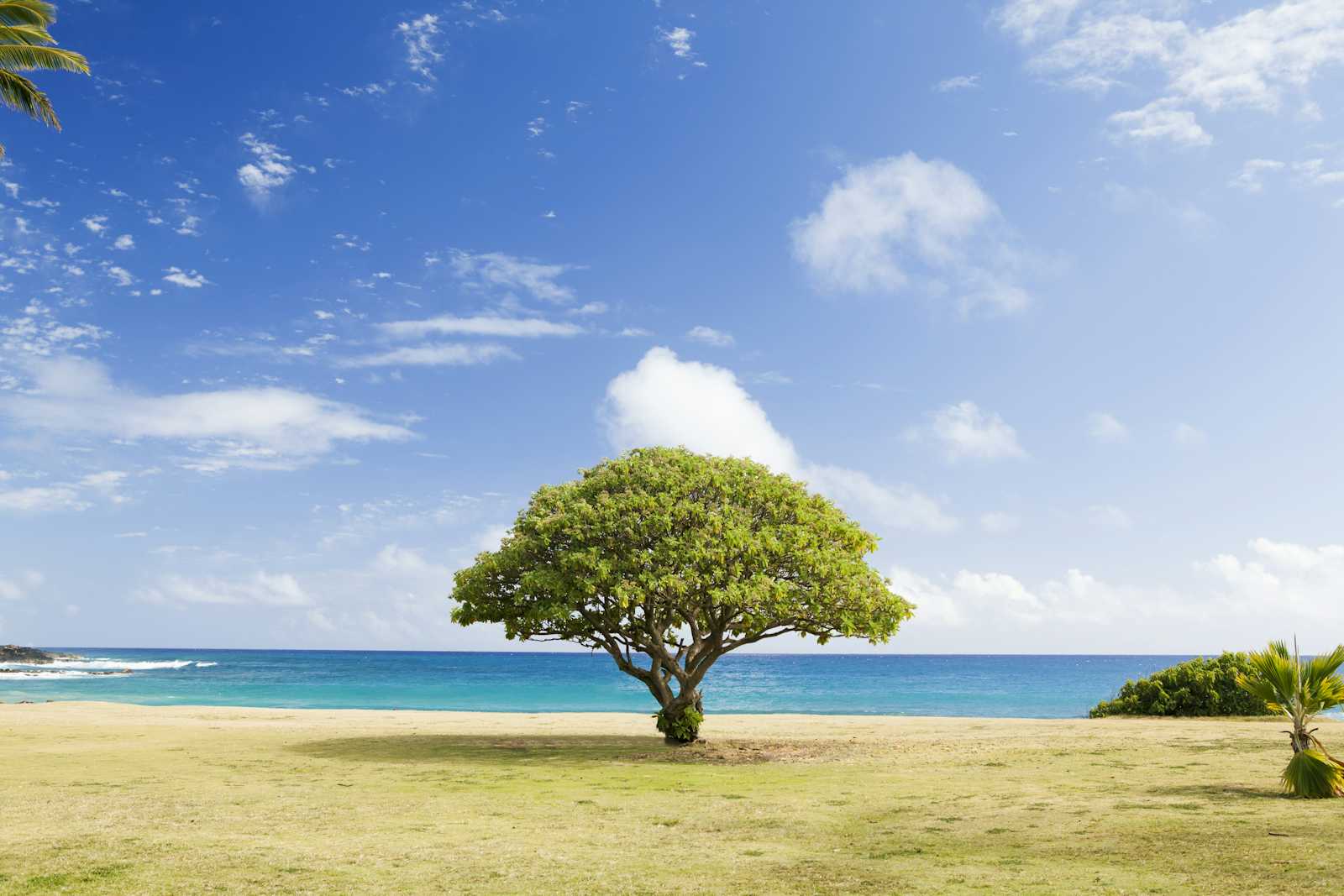
Introduction
Conversation
Dialog
Jason: Chad is our guest today and he's going to share his knowledge about binary trees. Thanks for coming, Chad.
Chad: Thanks.
Jason: So, welcome aboard.
Chad: A binary tree
is a fundamental data structure in computer science where each node
has at most
two children, referred to as the left child and the right child. The topmost node is called the root
, and nodes without children are called leaves
. The structure and properties of binary trees make them ideal for efficient searching, sorting, and managing hierarchical data.
Traversal methods
, such as preorder
, inorder
, and postorder
, are essential for accessing and manipulating binary trees. In preorder traversal, the root node is visited first, followed by the left subtree
, and then the right subtree. Inorder traversal visits the left subtree first, then the root node, and finally the right subtree, which is useful for retrieving data
in a sorted manner. Postorder traversal processes the left subtree, the right subtree, and the root node last.
Balancing
a binary tree ensures optimal performance by maintaining the tree's height, minimizing the time complexity for insertion, deletion, and search operations. Understanding binary trees and their traversal methods is crucial for solving complex computational problems efficiently.
Vocabulary
root | Leaf | node | at most |
preorder | Traversal Method | binary tree | subtree |
inorder | retrieve data | postorder | balance a binary tree |
Binary Tree
Explanation: A data structure in which each node has at most two children, called the left child and the right child.
Sample Sentences:
"A binary tree is used for efficient searching and sorting in computer science."
"Understanding the structure of a binary tree is fundamental for many algorithms."
Translation: Árvore binária
Node
Explanation: A basic unit of a data structure, such as a linked list or tree, containing data and links to other nodes.
Sample Sentences:
"Each node in a binary tree contains a value and links to its children."
"The root node is the topmost node in a binary tree."
Translation: Nó
At Most
Explanation: No more than; used to indicate the maximum number or limit.
Sample Sentences:
"In a binary tree, each node has at most two children."
"The function can process at most 100 elements at a time."
Translation: No máximo
Root
Explanation: The topmost node in a tree data structure from which all other nodes descend.
Sample Sentences:
"The root of the binary tree is the starting point for any traversal."
"If the root node is null, the binary tree is empty."
Translation: Raiz
Leaf (Leaves)
Explanation: Nodes in a tree data structure that do not have any children.
Sample Sentences:
"Leaves are the nodes at the bottom of a binary tree."
"A binary tree with many leaves is often deeper and more complex."
Translation: Folha (Folhas)
Preorder
Explanation: A type of tree traversal where the root node is visited first, followed by the left subtree, then the right subtree.
Sample Sentences:
"In preorder traversal, the root node is processed before its subtrees."
"Preorder traversal is useful for creating a copy of the tree."
Translation: Pré-ordem
Inorder
Explanation: A type of tree traversal where the left subtree is visited first, then the root node, followed by the right subtree.
Sample Sentences:
"Inorder traversal results in nodes being visited in ascending order."
"Inorder traversal is often used for binary search trees."
Translation: Em ordem
Postorder
Explanation: A type of tree traversal where the left subtree is visited first, then the right subtree, and the root node last.
Sample Sentences:
"Postorder traversal is used to delete the tree from the bottom up."
"In postorder traversal, the root node is the last to be processed."
Translation: Pós-ordem
Retrieve Data
Explanation: To access and obtain data stored in a data structure.
Sample Sentences:
"Inorder traversal is a way to retrieve data from a binary tree in sorted order."
"Efficient algorithms are necessary to retrieve data quickly from large datasets."
Translation: Recuperar dados
Subtree
Explanation: A smaller tree within a larger tree, consisting of a node and all its descendants.
Sample Sentences:
"Each node in a binary tree can be considered the root of a subtree."
"Balancing a binary tree often involves rearranging its subtrees."
Translation: Subárvore
Balance (a binary tree)
Explanation: The process of rearranging nodes in a binary tree to ensure that it remains balanced, minimizing its height.
Sample Sentences:
"Balancing a binary tree improves its performance for search operations."
"Several algorithms can be used to balance a binary tree automatically."
Translation: Balancear (uma árvore binária)
Traversal Method
Explanation: A technique used to visit all the nodes in a tree data structure in a specific order.
Sample Sentences:
"Traversal methods like preorder, inorder, and postorder are essential for accessing the nodes in a binary tree."
"Choosing the right traversal method depends on the specific task you need to accomplish with the binary tree."
Translation: Método de travessia
Exercises
Initial role play
root | Leaf | node | at most |
preorder | Traversal Method | binary tree | subtree |
inorder | retrieve data | postorder | balance a binary tree |
Final role play
root | Leaf | node | at most |
preorder | Traversal Method | binary tree | subtree |
inorder | retrieve data | postorder | balance a binary tree |
Personal experience
Homework
Fill-in-the-blanks
root | Leaf | node | at most |
preorder | Traversal Method | binary tree | subtree |
inorder | retrieve data | postorder | balance a binary tree |
A _______ _______ is used to visit all the nodes in a binary tree in a specific order.
Depending on the _______ _______, the nodes are accessed differently to achieve various tasks.
A _______ _______ is a data structure where each node has _______ two children.
Understanding a _______ _______ is crucial for efficient searching and sorting algorithms.
Each _______ in a binary tree contains a value and links to its children.
The root _______ is the topmost element in a binary tree.
In a binary tree, each node can have _______ _______ two children.
The function processes _______ _______ 100 elements at a time.
The _______ node is the starting point for any traversal in a binary tree.
If the _______ node is null, the binary tree is considered empty.
_______ are the nodes at the bottom of a binary tree that do not have any children.
A binary tree with many _______ is often deeper and more complex.
In _______ traversal, the root node is visited first, followed by the left subtree, then the right subtree.
_______ traversal is useful for creating a copy of the tree.
In _______ traversal, the left subtree is visited first, then the root node, followed by the right subtree.
_______ traversal results in nodes being visited in ascending order.
In _______ traversal, the left subtree is visited first, then the right subtree, and the root node last.
_______ traversal is used to delete the tree from the bottom up.
_______ _______ from a binary tree can be efficiently done using different traversal methods.
Efficient algorithms are necessary to _______ _______ quickly from large datasets.
Each node in a binary tree can be considered the root of a _______.
Balancing a binary tree often involves rearranging its _______.
_______ _______ _______ improves its performance for search operations.
Several algorithms can be used to _______ _______ _______ automatically.
Reading comprehension
What is a binary tree, and how many children can each node have in a binary tree?
What are the names given to the topmost node and the nodes without children in a binary tree?
Describe the three traversal methods mentioned in the text and explain their order of node visits.
Why is balancing a binary tree important, and what does it help to optimize?
How does inorder traversal differ from preorder and postorder traversal in terms of the order in which nodes are visited?
Produce
Create a new dialog or text with the following words:
root | Leaf | node | at most |
preorder | Traversal Method | binary tree | subtree |
inorder | retrieve data | postorder | balance a binary tree |
Subscribe to my newsletter
Read articles from Alexandre Calaça directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
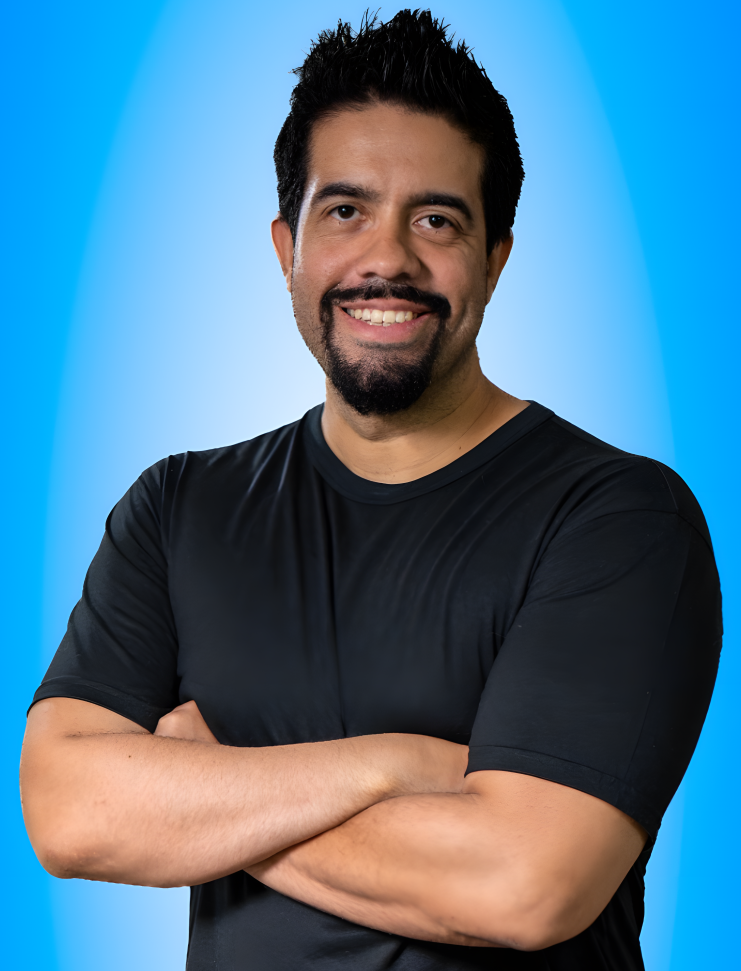
Alexandre Calaça
Alexandre Calaça
I'm a passionate software developer.