Mastering Exception Handling and Custom Exceptions in Python: A Comprehensive Guide

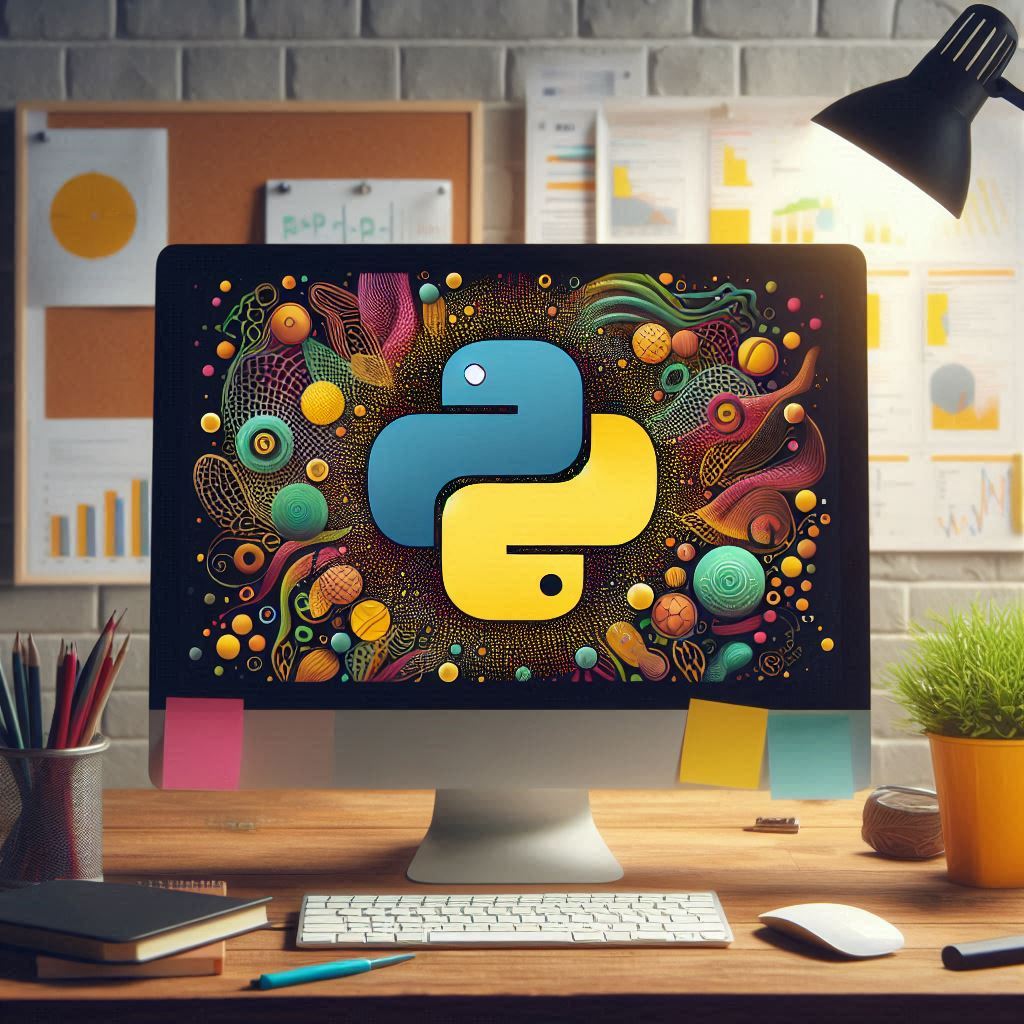
Exception handling is a crucial aspect of writing robust and fault-tolerant Python code. It allows you to manage errors gracefully and ensure that your program can handle unexpected situations without crashing. This article will cover the basics of exception handling, introduce custom exceptions, and provide detailed examples to help you understand how to use these concepts effectively.
What is Exception Handling?
In Python, exceptions are used to signal errors that occur during the execution of a program. Exception handling allows you to respond to these errors and manage them in a way that maintains the program's stability.
Basic Structure of Exception Handling
The basic structure of exception handling in Python involves the try
, except
, else
, and finally
blocks.
1. try
Block:
Contains the code that might raise an exception.
If an exception occurs, the rest of the
try
block is skipped.
2. except
Block:
Catches and handles specific exceptions.
You can have multiple
except
blocks to handle different exceptions.
3. else
Block (Optional):
Runs if no exceptions are raised in the
try
block.Useful for code that should execute only when no errors occur.
4. finally
Block (Optional):
Always executes, regardless of whether an exception occurred.
Ideal for cleanup actions like closing files or releasing resources.
Basic Examples
Example 1: Handling Specific Exceptions
try:
value = int(input("Enter a number: "))
print(f"You entered {value}")
except ValueError:
print("That's not a valid number!")
Explanation: In this example, if the user inputs something that is not a number, a ValueError
is raised, and the program prints an error message.
Example 2: Handling Multiple Exceptions
try:
file = open("non_existent_file.txt", "r")
except FileNotFoundError:
print("File not found.")
except IOError:
print("An I/O error occurred.")
Explanation: This example handles two different types of errors. If the file is not found, a FileNotFoundError
is caught. If there is an I/O error, an IOError
is caught.
Example 3: Using else
and finally
try:
number = int(input("Enter a number: "))
result = 10 / number
except ZeroDivisionError:
print("Cannot divide by zero.")
except ValueError:
print("Invalid input. Please enter a number.")
else:
print(f"Result is {result}")
finally:
print("This will always run.")
Explanation: Here, if no exceptions are raised, the else
block executes and prints the result. The finally
block always runs, ensuring that cleanup code is executed.
Custom Exceptions
Custom exceptions allow you to define your own error types that can be used to handle specific conditions in your program. They provide a way to create more meaningful and context-specific error messages.
Creating a Custom Exception
To create a custom exception, you define a new class that inherits from the built-in Exception
class.
Example: Custom Exception Class
class MyCustomError(Exception):
def __init__(self, message):
self.message = message
super().__init__(self.message)
def risky_operation():
raise MyCustomError("Something went wrong in the operation!")
try:
risky_operation()
except MyCustomError as e:
print(f"Caught an error: {e}")
Explanation: In this example, MyCustomError
is a custom exception that takes a message as an argument. The risky_operation
function raises this exception, and it is caught and handled in the try
block.
Why Use super().__init__(self.message)
?
When creating a custom exception, it’s important to understand why you should call super().__init__(self.message)
in the __init__
method.
Initialization of the Base Class: Calling
super().__init__(self.message)
ensures that the baseException
class is properly initialized with the error message. This allows the custom exception to inherit all the functionality of theException
class, including the ability to store and retrieve the error message.Consistency with Built-in Exceptions: By using
super()
, you ensure that your custom exception behaves consistently with built-in exceptions, which also use the__init__
method of theException
class to initialize the error message.Custom Attributes and Methods: While initializing the base class, you can also define custom attributes or methods specific to your exception. This makes your exceptions more informative and tailored to your application’s needs.
Example: Custom Exception with Additional Attributes
class ValidationError(Exception):
def __init__(self, message, field_name):
super().__init__(message)
self.field_name = field_name
def __str__(self):
return f"{self.field_name} - {self.message}"
def validate_input(value):
if not value:
raise ValidationError("This field cannot be empty.", "Input")
try:
validate_input("")
except ValidationError as e:
print(f"Validation failed: {e}")
Explanation: ValidationError
includes an additional attribute field_name
, which helps identify which field caused the error. The __str__
method is overridden to provide a more detailed error message.
Real-World Use Cases for Custom Exceptions
Handling User Input:
- Use custom exceptions to validate and handle errors related to user input, such as empty fields or invalid formats.
File Operations:
- Manage errors related to missing files, read/write permissions, or file corruption with custom exceptions.
Network Operations:
- Handle network-related exceptions like connection timeouts or server errors using custom exceptions.
Domain-Specific Errors:
- Represent specific error conditions relevant to your application domain, such as transaction failures in a banking application.
Interview Questions and Answers
What is the purpose of the
finally
block in exception handling?- Answer: The
finally
block is used to execute code that must run regardless of whether an exception occurred. This is useful for cleanup actions, such as closing files or releasing resources.
- Answer: The
How can you catch multiple exceptions in a single
except
block?- Answer: You can catch multiple exceptions by specifying them as a tuple in the
except
clause, like so:except (ExceptionType1, ExceptionType2) as e:
.
- Answer: You can catch multiple exceptions by specifying them as a tuple in the
What is the difference between
Exception
andBaseException
in Python?- Answer:
BaseException
is the base class for all built-in exceptions, including system-exiting exceptions.Exception
is the base class for all non-system-exiting exceptions. Most user-defined exceptions should inherit fromException
.
- Answer:
Can you re-raise an exception after catching it? How?
- Answer: Yes, you can re-raise an exception using the
raise
statement without arguments. This will re-raise the last exception caught.
- Answer: Yes, you can re-raise an exception using the
What happens if you don't handle an exception?
- Answer: If an exception is not handled, it will propagate up the call stack. If it reaches the top level of the program without being caught, it will cause the program to terminate.
Why is it important to call
super().__init__(self.message)
in a custom exception?- Answer: Calling
super().__init__(self.message)
ensures that the baseException
class is properly initialized with the error message. This allows the custom exception to inherit all the functionality of theException
class and ensures consistency with built-in exceptions.
- Answer: Calling
The above article provides a comprehensive overview of exception handling and custom exceptions in Python. Understanding these concepts will help you write more robust code and handle errors effectively in your applications.
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!