Understanding How Top Level "use-client" Affects Next.js Performance

Table of contents
- What Does the use client Directive Do?
- The Problem: Using the use client Directive Too High
- Bad Practice: Using use client Too High Just for Small Interactivity
- Why Is This Bad?
- Good Practice: Encapsulating Interactivity into a Client Component
- ProductPage Component as a Server Component
- Why Is This Good?
- Conclusion
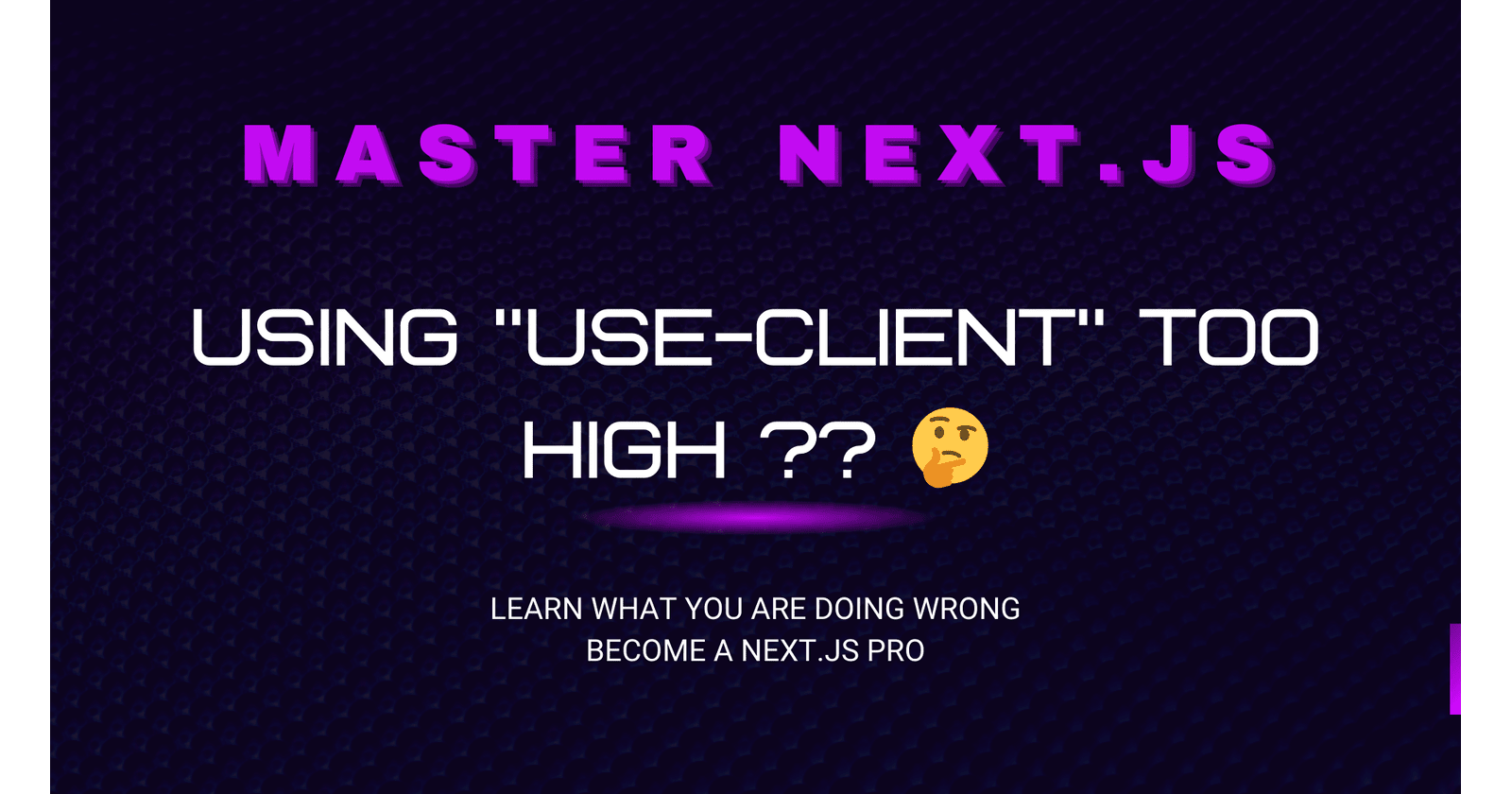
In Next.js, one of the biggest beginner mistakes — and even one made by experienced developers — is using the use client
directive too high in the component tree. This can lead to inefficient rendering, larger client bundles, and degraded performance.
In this article, we’ll break down why using the use client
directive too high is problematic, and how you can avoid this mistake to unlock the full potential of Next.js.
What Does the use client
Directive Do?
The use client
directive tells Next.js that a component should be treated as a client component. Client components run on the client-side and can use features like state management, event handling, and hooks (like useState
, useEffect
, etc.).
By default, components in Next.js are server components, meaning they are rendered on the server, providing better performance and reduced JavaScript sent to the client.
The Problem: Using the use client
Directive Too High
When developers place the use client
directive at the top level of a component (like a page), everything imported into that page and any components within that tree also become client components — even if they don’t need to be.
Bad Practice: Using use client
Too High Just for Small Interactivity
Bad Example: Marking the Whole Page as a Client Component
// pages/productPage.js
'use client'; // The whole page is now a client component
import { useState } from 'react';
export default function ProductPage() {
const [liked, setLiked] = useState(false);
const toggleLike = () => {
setLiked((prevLiked) => !prevLiked);
};
return (
<div>
<h1>Product Title</h1>
<button onClick={toggleLike}>
{liked ? 'Remove from Wishlist' : 'Add to Wishlist'}
</button>
</div>
);
}
In this example, we’ve added a simple wishlist button to a ProductPage
component. The button toggles between "Add to Wishlist" and "Remove from Wishlist" when clicked, which requires client-side interactivity.
Why Is This Bad?
The problem is that we’ve marked the entire ProductPage
as a client component ('use client'
). As a result, all content on this page, including the static product title, is rendered on the client, even though only the wishlist button actually needs to be interactive.
This means:
The entire component tree inside
ProductPage
will become a client component, increasing the bundle size unnecessarily.We lose the benefits of server-side rendering (SSR) for non-interactive parts, which could have been faster if rendered on the server.
Good Practice: Encapsulating Interactivity into a Client Component
Rather than making the entire ProductPage
a client component, we can isolate the interactive part (the wishlist button) into its own component and mark only that as a client component. The rest of the page, which doesn't need interactivity, will remain server-rendered, leading to better performance and smaller client-side bundles.
Good Example: Isolating the Wishlist Button into Its Own Client Component
// components/WishlistButton.js
'use client'; // Only this button is a client component
import { useState } from 'react';
export default function WishlistButton() {
const [liked, setLiked] = useState(false);
const toggleLike = () => {
setLiked((prevLiked) => !prevLiked);
};
return (
<button onClick={toggleLike}>
{liked ? 'Remove from Wishlist' : 'Add to Wishlist'}
</button>
);
}
Now, the WishlistButton
component is isolated and marked as a client component since it requires interactivity (state management for the wishlist functionality).
ProductPage Component as a Server Component
// pages/productPage.js
// Import the client component
import WishlistButton from '../components/WishlistButton';
export default function ProductPage() {
return (
<div>
<h1>Product Title</h1>
{/* Only the WishlistButton is a client component */}
<WishlistButton />
</div>
);
}
Why Is This Good?
In this structure, the ProductPage
component is rendered on the server, while only the WishlistButton
component is rendered on the client. This is a much more efficient approach because:
The static parts (like the product title) are server-rendered, which improves performance and reduces the JavaScript sent to the client.
The interactive part (wishlist button) is encapsulated and rendered on the client, ensuring only the necessary JavaScript for interactivity is sent.
Smaller client-side bundle: We avoid unnecessarily marking non-interactive components as client components.
By properly isolating interactivity, you get the full benefits of server-side rendering and client-side interactivity, making your app faster and more scalable.
Conclusion
In Next.js, effectively managing client and server components is crucial for building performant and scalable applications. Using the use client
directive too high in your component tree is a common mistake that can lead to unnecessary client-side rendering and bloated JavaScript bundles.
By isolating interactivity into dedicated client components, as demonstrated with the wishlist button example, you can leverage the full benefits of server-side rendering for static content while still providing rich, interactive experiences where needed. This approach ensures that your application remains fast and efficient, with a minimal amount of JavaScript sent to the client.
Avoiding the misuse of the use client
directive not only enhances performance but also aligns with best practices in Next.js development. Keep your component tree clean and optimized, and your users will enjoy a smoother, faster experience.
Stay tuned for more insights and best practices in the "You Don’t Know Next.js Series" , where i continue to uncover common pitfalls and share tips to help you become a Next.js pro.
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.