Why You Should Always Explicitly Mark Client Components in Next.js

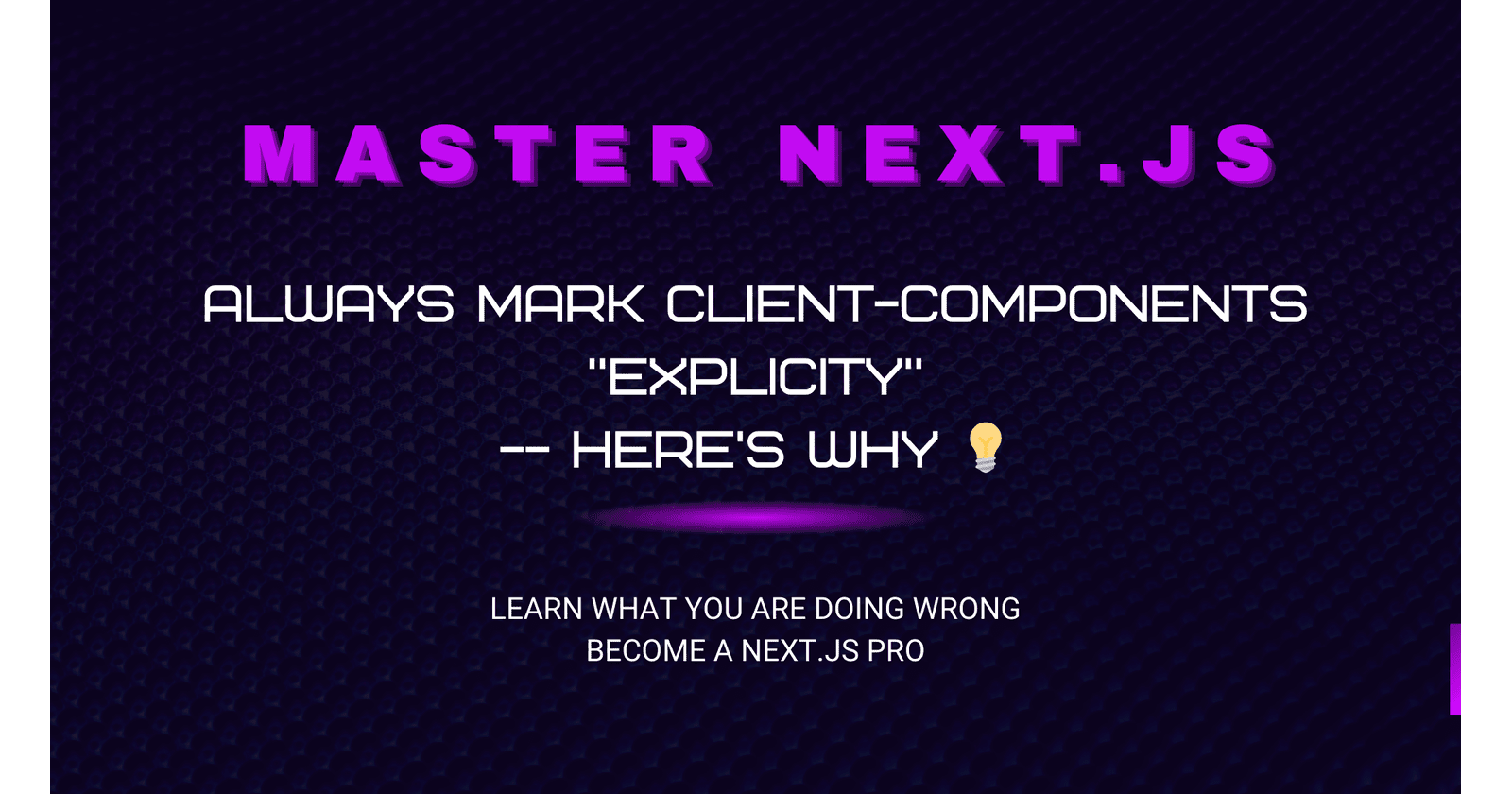
In Next.js, a common mistake developers make is assuming that a component without the use client
directive is a server component. This misunderstanding often arises when developers import components that use client-side functionality (like state or event handlers) into other client components. While this may work in the short term, it can lead to confusion and issues as the codebase grows.
In this article, we’ll dive into why it’s essential to explicitly mark components that rely on client-side features with the use client
directive — rather than relying on imports — and how failing to do so can create maintainability issues.
Understanding the use client
Directive
The use client
directive tells Next.js that a component should be treated as a client component, meaning it will be rendered on the client-side. Client components can use features like useState
, useEffect
, and event handlers that rely on the browser environment.
By default, components in Next.js are treated as server components, which are rendered on the server. These components do not have access to client-side features like state or DOM manipulation.
The Problem: Assuming a Component Is a Server Component Because It Lacks use client
It’s easy to assume that if a component doesn’t explicitly have the use client
directive at the top, it must be a server component. But this isn’t always true. Let’s break down a common scenario:
Example Scenario: Importing Client Functionality Without use client
Consider you have two components: a Sidebar
and a FavoriteButton
. The FavoriteButton
component is interactive — it manages state with useState
and listens to events like onClick
— meaning it needs to be a client component.
However, if FavoriteButton
is imported into Sidebar
, and Sidebar
is marked with the use client
directive, FavoriteButton
will automatically become a client component, even if it doesn’t have the use client
directive at the top.
Here’s an example:
Sidebar.js
(Client Component)
// components/Sidebar.js
'use client'; // Sidebar is explicitly a client component
import FavoriteButton from './FavoriteButton';
export default function Sidebar() {
return (
<div>
<h2>Sidebar</h2>
<FavoriteButton />
</div>
);
}
FavoriteButton.js
(Missing use client
directive but Uses Client-Side Features)
// components/FavoriteButton.js
import { useState } from 'react';
export default function FavoriteButton() {
const [liked, setLiked] = useState(false);
const toggleLike = () => {
setLiked((prevLiked) => !prevLiked);
};
return (
<button onClick={toggleLike}>
{liked ? 'Unlike' : 'Like'}
</button>
);
}
In this case, even though FavoriteButton.js
doesn’t have the use client
directive, it will function as a client component because it’s being used inside the Sidebar
component, which is explicitly marked as a client component.
Why This Is a Problem
At first glance, this may not seem like an issue, especially if the code works. However, as your application grows, this reliance on implicit behavior can lead to several problems:
Lack of Clarity: Future developers (or even you after some time) might not immediately understand why
FavoriteButton
is functioning as a client component. Without theuse client
directive, it can be unclear that this component relies on client-side functionality.Maintainability: As your codebase grows, it becomes harder to track which components are client components and which are server components. This can lead to confusion, especially if you’re working in a large team or revisiting the code after a long time.
Potential Bugs: If you move
FavoriteButton
to another part of your app that isn’t a client component, you could introduce bugs or break functionality because it’s no longer running on the client as expected.
Best Practice: Always Mark Client Components Explicitly
Even though FavoriteButton
works as a client component in the example above, the better practice is to explicitly mark it as a client component because it uses client-side features like useState
and event handlers.
Good Example: Explicitly Marking Client Components
FavoriteButton.js
(Explicitly Marked as a Client Component)
// components/FavoriteButton.js
'use client'; // Explicitly mark as a client component
import { useState } from 'react';
export default function FavoriteButton() {
const [liked, setLiked] = useState(false);
const toggleLike = () => {
setLiked((prevLiked) => !prevLiked);
};
return (
<button onClick={toggleLike}>
{liked ? 'Unlike' : 'Like'}
</button>
);
}
By marking FavoriteButton
as a client component explicitly, we make the code clearer and more maintainable. Anyone reading the code can immediately understand that this component relies on client-side functionality, without needing to trace how it’s being used elsewhere in the app.
Benefits of Explicitly Marking Client Components
Clarity: There’s no ambiguity about whether a component is a client or server component. It’s immediately clear from the presence of the
use client
directive.Consistency: By following this approach consistently throughout your codebase, you make the intent of your components clear and easy to understand for future developers (or your future self).
Avoiding Implicit Behavior: Relying on implicit behavior (like importing a component into a client component) can lead to subtle bugs or confusing code. Being explicit removes these potential issues.
Improved Codebase Hygiene: Clean, clear, and maintainable code is the hallmark of a well-structured application, and marking client components explicitly contributes to this.
Conclusion
Relying on imports to dictate whether a component functions as a client component is a common mistake in Next.js development. Even if a component works fine when imported into a client component, it’s a good practice to explicitly mark any component that uses client-side functionality (like state or event handlers) with the use client
directive. This approach ensures that your code is clear, maintainable, and free from potential confusion as your application grows.
By explicitly declaring client components, you reduce the chance of bugs, make your codebase more maintainable, and improve overall code clarity for yourself and future collaborators. So, the next time you write a component that relies on client-side features, remember to mark it as a client component — don't rely on implicit behavior!
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.