Think Client Components Only Run on the Client Side? Think Again

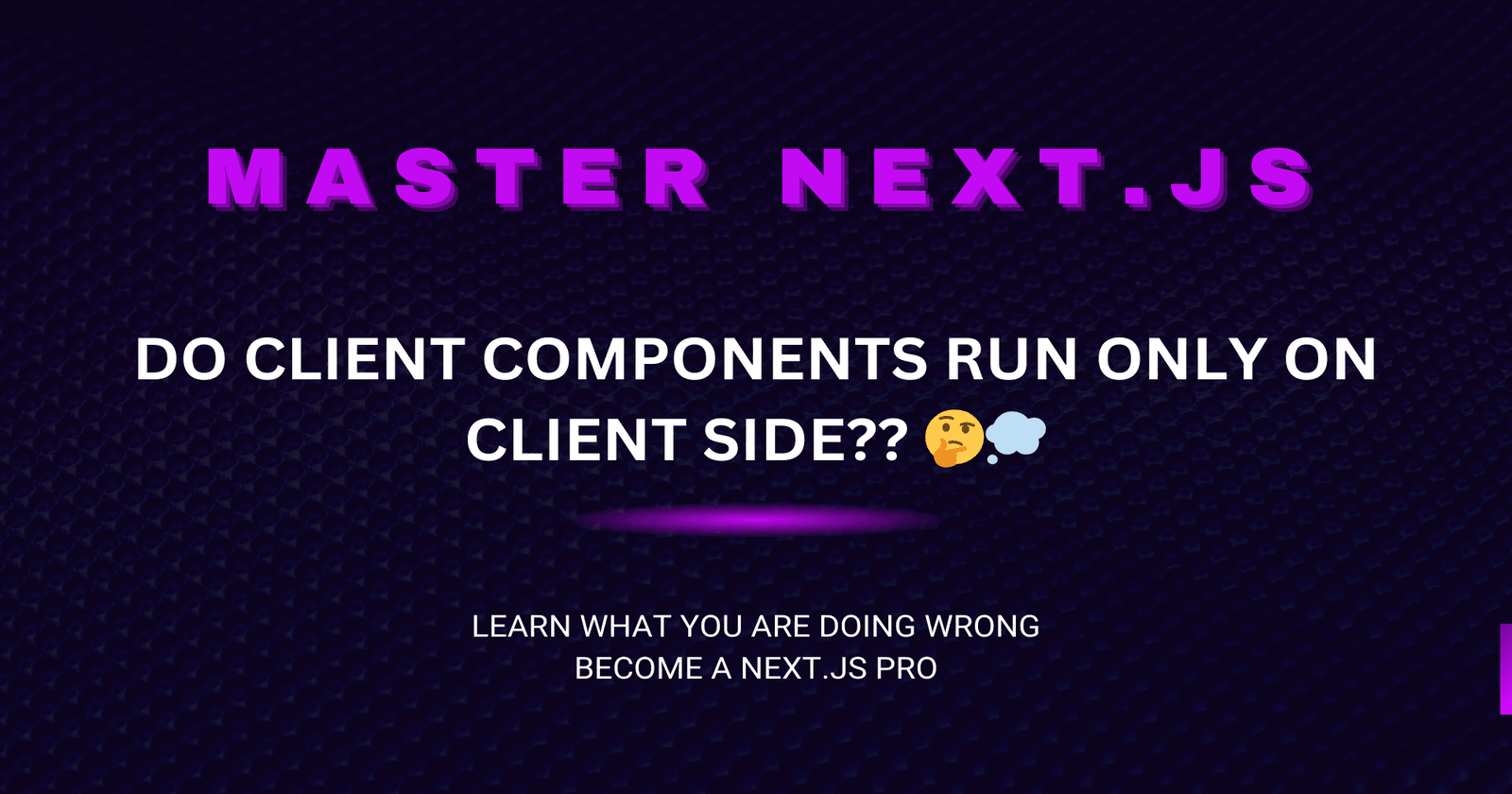
In Next.js, there’s often a misconception that client components run only on the client, as their name might suggest. However, this isn't entirely true. In reality, client components in Next.js execute both on the server during the initial page render and on the client after hydration. Understanding this dual behavior is essential for writing efficient, secure code, especially in server-rendered applications.
Server Components vs. Client Components
Before diving into client components, let's briefly clarify the behavior of server components. As expected, server components run exclusively on the server. When you add a console.log
statement to a server component, you'll only see the output in the server terminal, never in the browser console. This makes sense since server components are responsible for rendering static content or fetching data from the server, which is then sent to the client.
Client components, however, behave differently.
Client Components Execute on Both Server and Client
A common misconception is that client components run only on the client side. But when you add a console.log
statement inside a client component and request a page in Next.js, you’ll notice that the console.log
appears in both the server terminal and the browser console. This can be confusing, so let's understand why this happens.
The Server-Side Render (SSR) Process
When you request a page in Next.js, the framework tries to server-side render the entire page, including both server and client components. The goal here is to send a fully rendered HTML page to the client for better performance and SEO. This means that even client components get rendered on the server during the initial request, which triggers any code inside them—like console.log
.
The Role of the RSC (React Server Component) Payload
An important piece of this process is the RSC payload. When the server renders the page, including client components, it doesn’t just send the static HTML. Along with the HTML, the server sends an RSC payload to the client.
This RSC payload contains the necessary metadata and instructions that React needs to handle the client-side rendering and hydration of client components. It includes the following:
Component structure: Information about the components rendered on the server, including client components that need interactivity.
State and Props: Details about the state and props that were passed to these components, so the client can recreate the same environment.
Instructions for Hydration: Data that instructs React on how to "hydrate" client components on the client side. Hydration is the process where React binds the JavaScript logic to the static HTML.
The RSC payload is crucial because it provides the client with everything it needs to take over from the server-rendered HTML and manage the UI's interactivity. Without it, React wouldn't be able to properly hydrate the client components or ensure seamless transitions between server-side rendering and client-side interaction.
Hydration: React Taking Over on the Client
After the server has sent the rendered HTML along with the RSC payload, React performs a process called hydration. Hydration is React's way of taking over the static HTML and making it interactive. For example, when a user clicks a button or interacts with a form, React needs to manage the state changes and DOM updates.
During hydration, client components are re-executed on the client side to add the interactivity needed by React. This second execution triggers the console.log
in the browser, which is why you see the console.log
statement twice—once on the server (during SSR) and once on the client (during hydration).
Key Takeaways:
Server components only run on the server, and their code never executes on the client.
Client components run twice—first on the server during SSR and again on the client during hydration.
The RSC payload plays a crucial role by providing instructions to the client to handle hydration, which allows React to make the HTML interactive on the client side.
Hydration is React's process of making static HTML interactive, and it's a key reason why client components execute on both the server and the client during the initial render.
Why Does This Matter?
Understanding that client components run on both the server and client during the initial render is essential for optimizing your application and maintaining security. Here’s why:
Performance Considerations: When client components are executed on the server during the initial render, they can add unnecessary overhead, especially if they include complex logic or heavy computations that aren’t needed server-side. This can slow down the server-rendering process, affecting Time to First Byte (TTFB) and overall page performance. Consider simplifying client component logic that gets executed server-side, or limit their usage in SSR-heavy pages.
Data Handling and Privacy: When client components run on the server, any data they use, even if temporarily, can potentially be exposed in logs or during network transfers. Be cautious about fetching or manipulating sensitive user data in client components during SSR. For example, avoid using client-side logging, error handling, or any process involving confidential information, as this data might unintentionally be exposed during the server-side execution of client components.
Unintentional Server Execution: Developers often assume client components are isolated to the client, which could lead to writing code that’s inefficient or exposes unnecessary information to the server. Be aware of this dual rendering behavior when writing client-side components and ensure that only relevant logic is executed both server- and client-side.
Conclusion
In Next.js, client components are more versatile than their name suggests. While server components run strictly on the server, client components have a dual lifecycle: they run on the server during SSR and again on the client during hydration. The RSC payload plays an integral role by ensuring that the client has the necessary instructions for hydration, which allows React to take over and make the HTML interactive. Understanding this behavior, along with the role of the RSC payload, is crucial for optimizing performance and avoiding potential security risks in your applications.
By mastering the nuances of how Next.js handles server and client components, you can build more efficient, secure, and interactive applications.
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.