Step-by-Step Guide to Configuring Husky and Lint-Staged with ESLint and Prettier

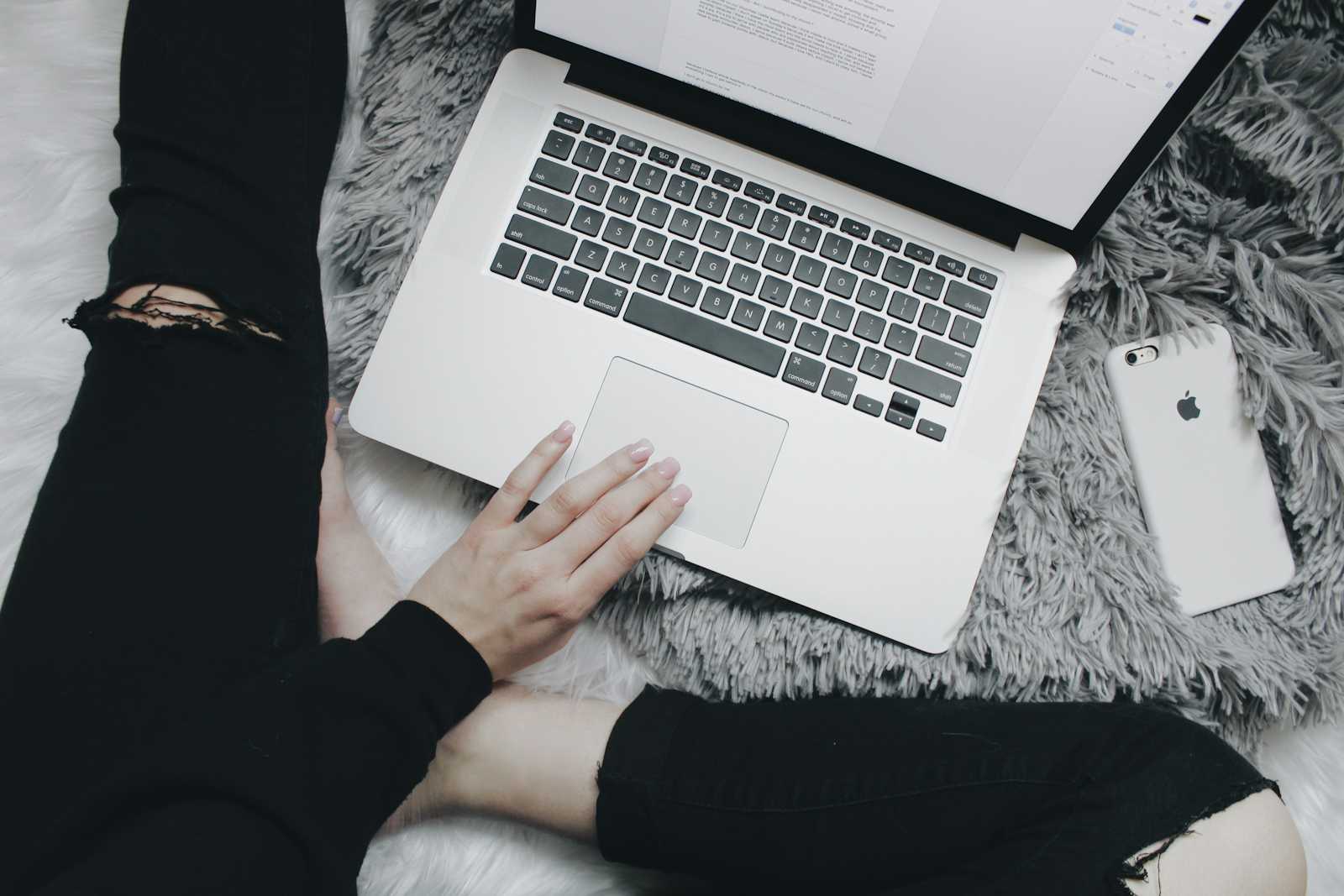
To set up Husky and lint-staged for running ESLint and Prettier automatically before every Git commit, follow these step-by-step instructions:
1. Install Husky and lint-staged
Start by installing Husky (for Git hooks) and lint-staged (to run linters only on staged files).
npm i -D husky lint-staged
2. Set Up Husky
Once installed, initialize Husky to create the husky
directory in your project.
npx husky install
This command will add the following entry to your package.json
:
{
"husky": {
"hooks": {}
}
}
It also creates a .husky/
folder where the Git hooks will reside.
3. Add a Pre-commit Hook
Use Husky to add a pre-commit hook that will trigger linting and formatting using ESLint and Prettier before each commit.
npx husky add .husky/pre-commit "npx lint-staged"
This command creates a .husky/pre-commit
file with the following content:
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npx lint-staged
4. Configure lint-staged
Next, configure lint-staged
in your package.json
to specify that ESLint and Prettier should run only on staged files. Add the following configuration:
{
"lint-staged": {
"*.{js,jsx,ts,tsx}": [
"eslint --fix",
"prettier --write"
]
}
}
This configuration ensures that ESLint will fix any linting issues, and Prettier will format files before they are committed.
5. Update Scripts in package.json
Ensure that your package.json
also includes the ESLint and Prettier scripts for linting and formatting manually:
{
"scripts": {
"lint": "eslint .",
"format": "prettier --write ."
}
}
6. Add Husky to Package Scripts
You should also add Husky to your post-install script to automatically install Husky after dependencies are installed:
{
"scripts": {
"prepare": "husky install"
}
}
7. Run Your First Commit
Now, when you make a commit, Husky will automatically run lint-staged
to check the staged files for linting and formatting issues:
git add .
git commit -m "Initial commit with Husky and lint-staged"
If ESLint or Prettier detects any issues, they will be automatically fixed before the commit is finalized.
8. Setup for TypeScript (Optional)
For TypeScript projects, make sure that your lint-staged
config accounts for .ts
and .tsx
files:
{
"lint-staged": {
"*.{js,jsx,ts,tsx}": [
"eslint --fix",
"prettier --write"
]
}
}
Recap
Install Husky and lint-staged to automate running ESLint and Prettier before every commit.
Configure lint-staged in
package.json
to target JavaScript/TypeScript files.Add a pre-commit hook using Husky to run
lint-staged
.ESLint and Prettier will ensure that your code is linted and formatted before it gets committed to Git.
By following these steps, you have an automated setup that helps maintain consistent code style across your project!
Subscribe to my newsletter
Read articles from J.A. Shezan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

J.A. Shezan
J.A. Shezan
Shezan loves technology who is currently studying Computer Science and Engineering. He codes frontend & backend of a website. He also does penetration testing on web apps.