Mongodb aggregation methods
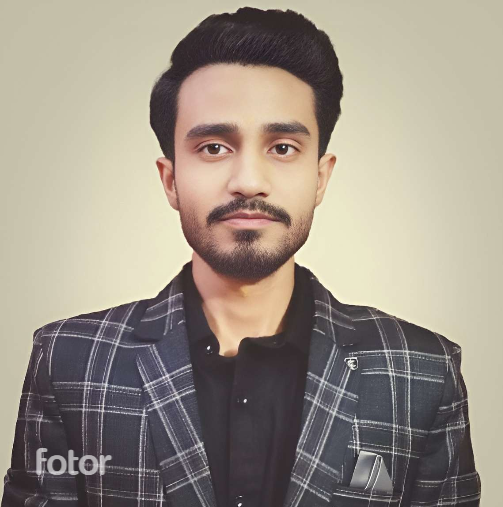
Let’s go through each aggregation stage example again, this time with sample collections and expected outputs.
Sample Collection
Assume we have the following sample products
collection:
[
{ "_id": 1, "name": "Product A", "category": "Electronics", "price": 100, "status": "active", "tags": ["new", "sale"] },
{ "_id": 2, "name": "Product B", "category": "Electronics", "price": 200, "status": "inactive", "tags": ["popular"] },
{ "_id": 3, "name": "Product C", "category": "Books", "price": 50, "status": "active", "tags": ["bestseller", "new"] },
{ "_id": 4, "name": "Product D", "category": "Books", "price": 80, "status": "active", "tags": ["new"] },
{ "_id": 5, "name": "Product E", "category": "Clothing", "price": 30, "status": "inactive", "tags": ["sale"] }
]
1. $match
Query: Filter documents where status
is "active"
.
const result = await collection.aggregate([{ $match: { status: "active" } }]).toArray();
Output:
[
{ "_id": 1, "name": "Product A", "category": "Electronics", "price": 100, "status": "active", "tags": ["new", "sale"] },
{ "_id": 3, "name": "Product C", "category": "Books", "price": 50, "status": "active", "tags": ["bestseller", "new"] },
{ "_id": 4, "name": "Product D", "category": "Books", "price": 80, "status": "active", "tags": ["new"] }
]
2. $group
Query: Group by category
and calculate the total count.
const result = await collection.aggregate([
{ $group: { _id: "$category", total: { $sum: 1 } } }
]).toArray();
Output:
[
{ "_id": "Electronics", "total": 2 },
{ "_id": "Books", "total": 2 },
{ "_id": "Clothing", "total": 1 }
]
3. $sort
Query: Sort by price
in descending order.
const result = await collection.aggregate([{ $sort: { price: -1 } }]).toArray();
Output:
[
{ "_id": 2, "name": "Product B", "category": "Electronics", "price": 200, "status": "inactive", "tags": ["popular"] },
{ "_id": 1, "name": "Product A", "category": "Electronics", "price": 100, "status": "active", "tags": ["new", "sale"] },
{ "_id": 4, "name": "Product D", "category": "Books", "price": 80, "status": "active", "tags": ["new"] },
{ "_id": 3, "name": "Product C", "category": "Books", "price": 50, "status": "active", "tags": ["bestseller", "new"] },
{ "_id": 5, "name": "Product E", "category": "Clothing", "price": 30, "status": "inactive", "tags": ["sale"] }
]
4. $project
Query: Include only name
and price
fields.
const result = await collection.aggregate([
{ $project: { name: 1, price: 1, _id: 0 } }
]).toArray();
Output:
[
{ "name": "Product A", "price": 100 },
{ "name": "Product B", "price": 200 },
{ "name": "Product C", "price": 50 },
{ "name": "Product D", "price": 80 },
{ "name": "Product E", "price": 30 }
]
5. $unwind
Query: Deconstruct the tags
array.
const result = await collection.aggregate([{ $unwind: "$tags" }]).toArray();
Output:
[
{ "_id": 1, "name": "Product A", "category": "Electronics", "price": 100, "status": "active", "tags": "new" },
{ "_id": 1, "name": "Product A", "category": "Electronics", "price": 100, "status": "active", "tags": "sale" },
{ "_id": 2, "name": "Product B", "category": "Electronics", "price": 200, "status": "inactive", "tags": "popular" },
{ "_id": 3, "name": "Product C", "category": "Books", "price": 50, "status": "active", "tags": "bestseller" },
{ "_id": 3, "name": "Product C", "category": "Books", "price": 50, "status": "active", "tags": "new" },
{ "_id": 4, "name": "Product D", "category": "Books", "price": 80, "status": "active", "tags": "new" },
{ "_id": 5, "name": "Product E", "category": "Clothing", "price": 30, "status": "inactive", "tags": "sale" }
]
6. $lookup
Query: Join with an orders
collection to include order details. Assume orders
collection:
[
{ "_id": 101, "productId": 1, "quantity": 2 },
{ "_id": 102, "productId": 3, "quantity": 1 }
]
const result = await collection.aggregate([
{ $lookup: {
from: "orders",
localField: "_id",
foreignField: "productId",
as: "orderDetails"
} }
]).toArray();
Output:
[
{ "_id": 1, "name": "Product A", "orderDetails": [{ "_id": 101, "productId": 1, "quantity": 2 }] },
{ "_id": 3, "name": "Product C", "orderDetails": [{ "_id": 102, "productId": 3, "quantity": 1 }] },
...
]
7. $addFields
Query: Add a discountedPrice
field (10% discount).
const result = await collection.aggregate([
{ $addFields: { discountedPrice: { $multiply: ["$price", 0.9] } } }
]).toArray();
Output:
[
{ "_id": 1, "name": "Product A", "price": 100, "discountedPrice": 90 },
{ "_id": 2, "name": "Product B", "price": 200, "discountedPrice": 180 },
...
]
8. $replaceRoot
Query: Replace root with tags
.
const result = await collection.aggregate([
{ $replaceRoot: { newRoot: { tags: "$tags" } } }
]).toArray();
Output:
[
{ "tags": ["new", "sale"] },
{ "tags": ["popular"] },
...
]
9. $limit
Query: Limit to 2 documents.
const result = await collection.aggregate([{ $limit: 2 }]).toArray();
Output:
[
{ "_id": 1, "name": "Product A", "category": "Electronics", ... },
{ "_id": 2, "name": "Product B", "category": "Electronics", ... }
]
10. $skip
Query: Skip the first 2 documents.
const result = await collection.aggregate([{ $skip: 2 }]).toArray();
Output:
[
{ "_id": 3, "name": "Product C", "category": "Books", ... },
...
]
Subscribe to my newsletter
Read articles from Jayesh Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
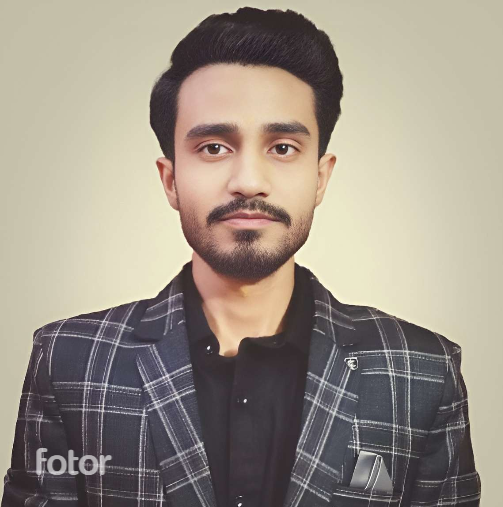
Jayesh Chauhan
Jayesh Chauhan
Hey I am Full Stack Developer.