👊Automating Network Health Checks with PowerShell & Scheduled Tasks⚙️

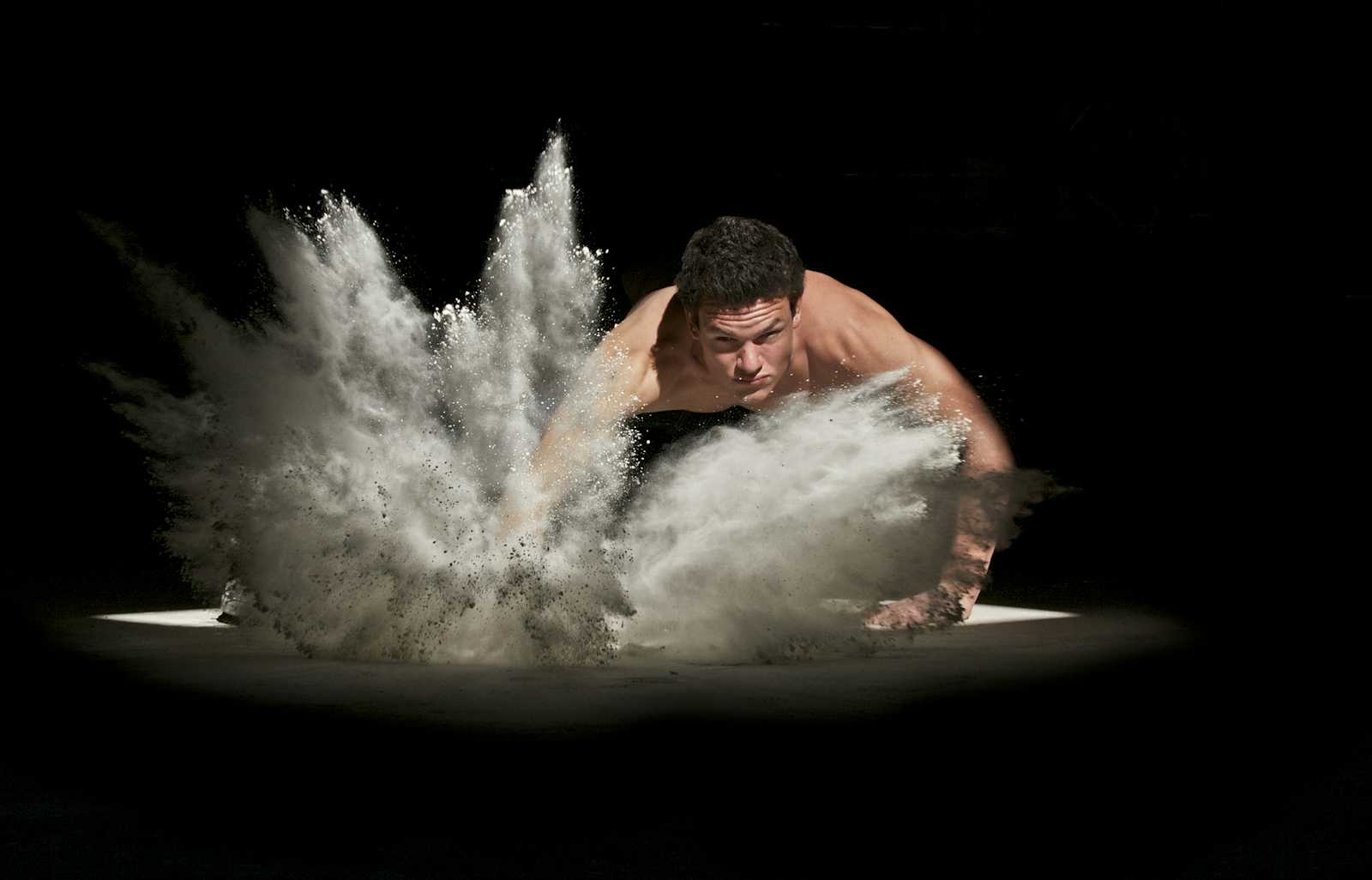
Monitoring network connectivity is crucial, especially if you're managing infrastructure or working with mission-critical applications. A simple way to ensure your network remains healthy is by periodically checking latency to key destinations and logging the results.
In this guide, I'll show you how to:
Use PowerShell to ping both an external server (
1.1.1.1
) and your default gateway.Send the latency results to an external monitoring API.
Automate the script using Windows Task Scheduler so it runs every 10 minutes.
Step 1: The PowerShell Script
We’ll create a script that:
✅ Dynamically detects the default gateway.
✅ Pings both 1.1.1.1 (Cloudflare DNS) and the gateway.
✅ Sends the latency results to an external API (digger.uptimekuma.com
).
✅ Gracefully handles failures (e.g., network down).
The Script (heartbeat.ps
1
)
# Get the default gateway reliably
$gateway = (Get-NetIPConfiguration | Where-Object { $_.IPv4DefaultGateway -ne $null -and $_.NetAdapter.Status -eq "Up" }).IPv4DefaultGateway.NextHop
# Function to calculate average ping latency
function Get-AveragePing {
param ($Target)
try {
$pingResults = Test-Connection -ComputerName $Target -Count 5 -ErrorAction Stop
return ($pingResults | Measure-Object -Property ResponseTime -Average).Average
} catch {
return "N/A"
}
}
# Ping 1.1.1.1 and send the result
$latency1 = Get-AveragePing -Target "1.1.1.1"
$api1 = "https://digger.uptimekuma.com/api/push/O6SLd8pNDv?status=up&msg=OK&ping=$latency1"
Invoke-RestMethod -Uri $api1 -Method Get
# Ping the local gateway and send the result
$latency2 = Get-AveragePing -Target $gateway
$api2 = "https://digger.uptimekuma.com/api/push/GwQREfeG3V?status=up&msg=OK&ping=$latency2"
Invoke-RestMethod -Uri $api2 -Method Get
🔹 How it Works:
Get-NetIPConfiguration
fetches the correct default gateway dynamically.The
Get-AveragePing
function runs 5 pings to the given target and calculates the average response time.Two API calls send latency results to
digger.uptimekuma.com
.If a ping fails, it returns
"N/A"
instead of crashing.
Step 2: Scheduling the Script with Task Scheduler
We’ll now automate this script to run every 10 minutes using Task Scheduler.
1. Save the Script
Open Notepad, paste the script, and save it as:
C:\Binaries\heartbeat.ps1
2. Allow PowerShell Scripts to Run
By default, Windows restricts PowerShell scripts. To fix this:
Open PowerShell as Administrator
Run:
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
3. Create a Scheduled Task
1️⃣ Open Task Scheduler (Win + R → taskschd.msc → Enter
).
2️⃣ Click "Create Basic Task" on the right.
3️⃣ Name it "Network Heartbeat", then click Next.
4️⃣ Select "Daily", then Next.
5️⃣ Set Start Time (any time) and Recur every 1 days, then Next.
6️⃣ Choose "Repeat task every 10 minutes", for a duration of 1 day.
7️⃣ Select "Start a Program", then Next.
8️⃣ In Program/Script, enter:
powershell.exe
9️⃣ In Add arguments, enter:
-ExecutionPolicy Bypass -File "C:\Binaries\heartbeat.ps1"
🔟 Click Finish.
Step 3: Verifying Everything Works
Run the Script Manually
To test before scheduling:
powershell.exe -ExecutionPolicy Bypass -File "C:\Binaries\heartbeat.ps1"
If everything is working, you should see API requests sent without errors.
Wrapping Up
🚀 Now, the script will automatically run every 10 minutes and log latency metrics to an external API. This provides continuous monitoring without manual intervention.
🔧 Need More? Modify the script to:
Log results to a file instead.
Send email alerts if latency exceeds a threshold.
Monitor additional network endpoints.
This method is lightweight, effective, and fully automated—ideal for keeping an eye on network health!
Subscribe to my newsletter
Read articles from Ronald Bartels directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ronald Bartels
Ronald Bartels
Driving SD-WAN Adoption in South Africa